Create a blank 10x10 integer array using constants for the row and column Create a function printArray() that will print every row and column of the array Create a function notFound() that searches each element in the array and returns true if the passed number already exists in the array and false if it does not Create a function fillArray() that will fill the 2 dimensional array with unique random numbers from 0 to 100 inclusive. No cells should have the same number. Use the notFound() function in this function. Print the entire array. Request a column number to print and use a sentinal loop to make sure the row number is valid. If not then request a column number again Create a function printIter() that prints only the passed column using iteration Create a function printRecur() that prints only the passed column using recursion Print the requested column using printIter() and printRecur()
C++
Problem #1:
- Create a blank 10x10 integer array using constants for the row and column
- Create a function printArray() that will print every row and column of the array
- Create a function notFound() that searches each element in the array and returns true if the passed number already exists in the array and false if it does not
- Create a function fillArray() that will fill the 2 dimensional array
with unique random numbers from 0 to 100 inclusive. No cells should have the same number. Use the notFound() function in this function.
- Print the entire array.
- Request a column number to print and use a sentinal loop to make sure the row number is valid. If not then request a column number again
- Create a function printIter() that prints only the passed column using iteration
- Create a function printRecur() that prints only the passed column using recursion
- Print the requested column using printIter() and printRecur()
Sample output:
The array is:
Row 0: 41, 85, 72, 38, 80, 69, 65, 68, 96,
Row 1: 22, 49, 67, 51, 61, 63, 87, 66, 24,
Row 2: 83, 71, 60, 64, 52, 90, 31, 23, 99,
Row 3: 94, 11, 25, 15, 13, 39, 97, 19, 76,
Row 4: 33, 18, 92, 35, 74, 95, 32, 37, 45,
Row 5: 57, 5, 86, 54, 75, 70, 29, 58, 46,
Row 6: 17, 3, 48, 77, 59, 62, 2, 78, 7,
Row 7: 84, 12, 47, 34, 21, 20, 9, 28, 100,
Row 8: 93, 4, 79, 81, 98, 91, 82, 1, 42,
Row 9: 88, 53, 56, 44, 73, 0, 30, 89, 55,
Enter a column number: 22
Enter a column number: -4
Enter a column number: 7
Iterative: 68, 66, 23, 19, 37, 58, 78, 28, 1,
Recursive: 68, 66, 23, 19, 37, 58, 78, 28, 1,

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

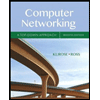
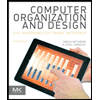
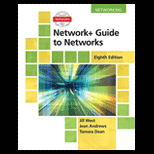
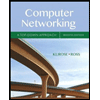
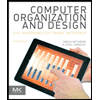
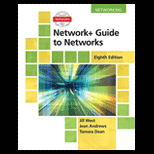
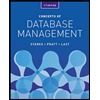
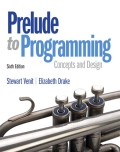
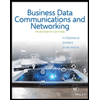