convert the following c++ code in c #include #include using namespace std; class Complex { public: Complex(double r = 0.0, double i = 0.0); // constructor void setReal(double real); void setImag(double imag); double getReal(); double getImag(); Complex operator+(const Complex&)const ; // operator+() Complex operator-(const Complex&)const ; // operator-() /** * read the complex number to an input stream */ friend std::istream & operator >>(std::istream &din, Complex &); /** * Friend function declarations * Output complex number to an output stream, Example 3+4i */ friend std::ostream & operator <<(std::ostream &dout, const Complex&); operator double(); private: //Declaring variables double real, imaginary; }; //Parameterized constructor Complex::Complex(double r,double i) { this->real=r; this->imaginary=i; } //Function implementation which adds two complex numbers by using the operator overloading '+' Complex Complex::operator +(const Complex &c)const { Complex temp; temp.real=real+c.real; temp.imaginary=imaginary+c.imaginary; return temp; } //Function implementation which subtract two complex numbers by using the operator overloading '-' Complex Complex::operator -(const Complex &c)const { Complex temp; temp.real=real-c.real; temp.imaginary=imaginary-c.imaginary; return temp; } //Function implementation which read complex numbers by using the operator overloading '>>' istream & operator >> (istream &din, Complex &c) { char op,i; din>>c.real; din>>op; din>>c.imaginary; din>>i; if(op=='-') c.imaginary=-(c.imaginary); return din; } //Function implementation which display complex numbers by using the operator overloading '<<' ostream & operator << (ostream &dout, const Complex &c) { if(c.imaginary<0) dout<>X; cin>>Y; cout<<"Complex Number#1:"<
convert the following c++ code in c
#include <iostream>
#include <iomanip>
using namespace std;
class Complex
{
public:
Complex(double r = 0.0, double i = 0.0); // constructor
void setReal(double real);
void setImag(double imag);
double getReal();
double getImag();
Complex operator+(const Complex&)const ; // operator+()
Complex operator-(const Complex&)const ; // operator-()
/**
* read the complex number to an input stream
*/
friend std::istream & operator >>(std::istream &din, Complex &);
/**
* Friend function declarations
* Output complex number to an output stream, Example 3+4i
*/
friend std::ostream & operator <<(std::ostream &dout, const Complex&);
operator double();
private:
//Declaring variables
double real, imaginary;
};
//Parameterized constructor
Complex::Complex(double r,double i)
{
this->real=r;
this->imaginary=i;
}
//Function implementation which adds two complex numbers by using the operator overloading '+'
Complex Complex::operator +(const Complex &c)const
{
Complex temp;
temp.real=real+c.real;
temp.imaginary=imaginary+c.imaginary;
return temp;
}
//Function implementation which subtract two complex numbers by using the operator overloading '-'
Complex Complex::operator -(const Complex &c)const
{
Complex temp;
temp.real=real-c.real;
temp.imaginary=imaginary-c.imaginary;
return temp;
}
//Function implementation which read complex numbers by using the operator overloading '>>'
istream & operator >> (istream &din, Complex &c)
{
char op,i;
din>>c.real;
din>>op;
din>>c.imaginary;
din>>i;
if(op=='-')
c.imaginary=-(c.imaginary);
return din;
}
//Function implementation which display complex numbers by using the operator overloading '<<'
ostream & operator << (ostream &dout, const Complex &c)
{
if(c.imaginary<0)
dout<<c.real<<" - "<<(-c.imaginary)<<" i ";
else
dout<<c.real<<" + "<<c.imaginary<<" i ";
return dout;
}
// conversion operator: return float value of fraction
Complex::operator double() {
return double(real) / double(imaginary);
}
int main()
{
Complex X,Y,Z;
cout<<"Enter two complex numbers (in form 7.1+8.3i):"<<endl;
cin>>X;
cin>>Y;
cout<<"Complex Number#1:"<<X<<endl;
cout<<"Complex Number#2:"<<Y<<endl;
Z=X+Y;
cout<<X<<" + "<<Y<<" = "<<Z<<endl;
system("pause");
return 0;
}
#include <iostream>
#include <iomanip>
using namespace std;
class Complex
{
public:
Complex(double r = 0.0, double i = 0.0); // constructor
void setReal(double real);
void setImag(double imag);
double getReal();
double getImag();
Complex operator+(const Complex&)const ; // operator+()
Complex operator-(const Complex&)const ; // operator-()
/**
* read the complex number to an input stream
*/
friend std::istream & operator >>(std::istream &din, Complex &);
/**
* Friend function declarations
* Output complex number to an output stream, Example 3+4i
*/
friend std::ostream & operator <<(std::ostream &dout, const Complex&);
operator double();
private:
//Declaring variables
double real, imaginary;
};
//Parameterized constructor
Complex::Complex(double r,double i)
{
this->real=r;
this->imaginary=i;
}
//Function implementation which adds two complex numbers by using the operator overloading '+'
Complex Complex::operator +(const Complex &c)const
{
Complex temp;
temp.real=real+c.real;
temp.imaginary=imaginary+c.imaginary;
return temp;
}
//Function implementation which subtract two complex numbers by using the operator overloading '-'
Complex Complex::operator -(const Complex &c)const
{
Complex temp;
temp.real=real-c.real;
temp.imaginary=imaginary-c.imaginary;
return temp;
}
//Function implementation which read complex numbers by using the operator overloading '>>'
istream & operator >> (istream &din, Complex &c)
{
char op,i;
din>>c.real;
din>>op;
din>>c.imaginary;
din>>i;
if(op=='-')
c.imaginary=-(c.imaginary);
return din;
}
//Function implementation which display complex numbers by using the operator overloading '<<'
ostream & operator << (ostream &dout, const Complex &c)
{
if(c.imaginary<0)
dout<<c.real<<" - "<<(-c.imaginary)<<" i ";
else
dout<<c.real<<" + "<<c.imaginary<<" i ";
return dout;
}
// conversion operator: return float value of fraction
Complex::operator double() {
return double(real) / double(imaginary);
}
int main()
{
Complex X,Y,Z;
cout<<"Enter two complex numbers (in form 7.1+8.3i):"<<endl;
cin>>X;
cin>>Y;
cout<<"Complex Number#1:"<<X<<endl;
cout<<"Complex Number#2:"<<Y<<endl;
Z=X+Y;
cout<<X<<" + "<<Y<<" = "<<Z<<endl;
system("pause");
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

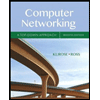
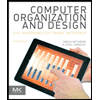
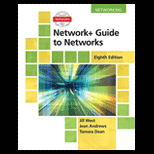
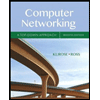
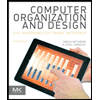
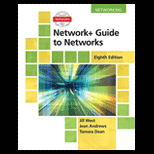
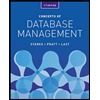
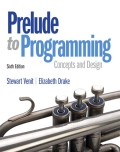
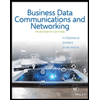