Convert Newton’s method for approximating square roots in Project 1 to a recursive function named newton. (Hint: The estimate of the square root should be passed as a second argument to the function.) An example of the program input and output is shown below: Enter a positive number or enter/return to quit: 2 The program's estimate is 1.4142135623746899 Python's estimate is 1.4142135623730951 Enter a positive number or enter/return to quit Given Code: # Modify the code below """ File: newton.py Project 6.1 Compute the square root of a number (uses function with loop). 1. The input is a number, or enter/return to halt the input process. 2. The outputs are the program's estimate of the square root using Newton's method of successive approximations, and Python's own estimate using math.sqrt. """ import math # Initialize the tolerance TOLERANCE = 0.000001 def newton(x): """Returns the square root of x.""" # Perform the successive approximations estimate = 1.0 while True: estimate = (estimate + x / estimate) / 2 difference = abs(x - estimate ** 2) if difference <= TOLERANCE: break return estimate def main(): """Allows the user to obtain square roots.""" while True: # Receive the input number from the user x = input("Enter a positive number or enter/return to quit: ") if x == "": break x = float(x) # Output the result print("The program's estimate is", newton(x)) print("Python's estimate is ", math.sqrt(x)) if __name__ == "__main__": main()
Convert Newton’s method for approximating square roots in Project 1 to a recursive function named newton. (Hint: The estimate of the square root should be passed as a second argument to the function.)
An example of the
Enter a positive number or enter/return to quit: 2 The program's estimate is 1.4142135623746899 Python's estimate is 1.4142135623730951 Enter a positive number or enter/return to quit
Given Code:
# Modify the code below
"""
File: newton.py
Project 6.1
Compute the square root of a number (uses function with loop).
1. The input is a number, or enter/return to halt the
input process.
2. The outputs are the program's estimate of the square root
using Newton's method of successive approximations, and
Python's own estimate using math.sqrt.
"""
import math
# Initialize the tolerance
TOLERANCE = 0.000001
def newton(x):
"""Returns the square root of x."""
# Perform the successive approximations
estimate = 1.0
while True:
estimate = (estimate + x / estimate) / 2
difference = abs(x - estimate ** 2)
if difference <= TOLERANCE:
break
return estimate
def main():
"""Allows the user to obtain square roots."""
while True:
# Receive the input number from the user
x = input("Enter a positive number or enter/return to quit: ")
if x == "":
break
x = float(x)
# Output the result
print("The program's estimate is", newton(x))
print("Python's estimate is ", math.sqrt(x))
if __name__ == "__main__":
main()

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

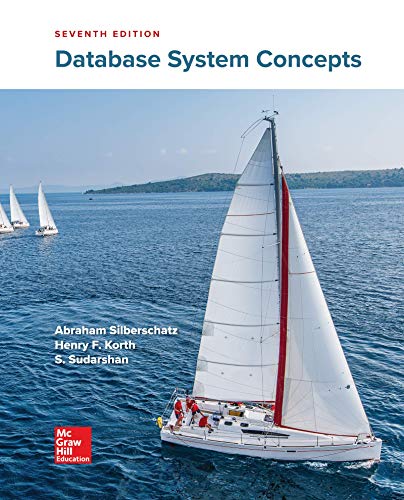
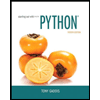
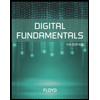
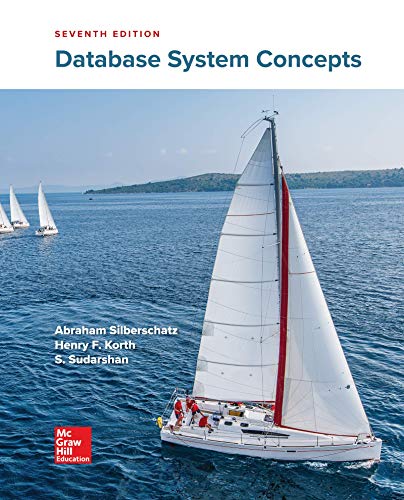
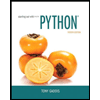
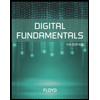
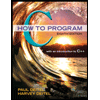
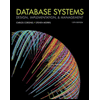
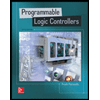