Consider this Date type. Write a factory function to create a date. Throw invalid_argument indicating which part of the date is invalid. You may use the predefined isLeapYear function in your code. struct Date { int day, month, year; };
Consider this Date type. Write a factory function to create a date. Throw invalid_argument indicating which part of the date is invalid. You may use the predefined isLeapYear function in your code. struct Date { int day, month, year; };
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Can someone help me with this?

Transcribed Image Text:### Creating a Valid Date in C++
In this lesson, we'll explore how to create a valid `Date` type using C++. The task involves writing a factory function to create a date and handle invalid input by throwing an `invalid_argument` exception, indicating which part of the date is invalid. You'll utilize a predefined `isLeapYear` function for this purpose.
#### Structure Definition
```cpp
struct Date {
int day, month, year;
};
```
#### File: `udts.cpp`
The following is an implementation description and code snippet from `udts.cpp`:
```cpp
#include <string>
#include <stdexcept>
#include "date.h"
using namespace std;
/**
* Construct a valid Date object.
* @param m the month in the range 1-12
* @param d the day in the range 1-31 (check for leap year)
* @param y the year in the range 1582-2099
* @exception throw invalid_argument if out of range.
* @return the constructed Date object.
*/
Date makeDate(int m, int d, int y)
{
...
}
```
- **Includes**: The code begins by including necessary headers: `<string>`, `<stdexcept>`, and `"date.h"`.
- **Namespace**: The standard namespace is used (`using namespace std;`).
- **Function Documentation**: The `makeDate` function constructs a `Date` object if the input values for month, day, and year are within valid ranges. The function parameters are:
- `m` (month): should be within the range 1-12.
- `d` (day): should be within the range 1-31, and consideration for leap years is required.
- `y` (year): should be between 1582 and 2099.
- **Exception Handling**: The function throws an `invalid_argument` exception if any input value is out of the allowed range, ensuring robust error handling.
- **Function Return**: On successful validation, a `Date` object is returned.
In summary, this code snippet demonstrates how to construct a validated `Date` object in C++, ensuring that the input adheres to the Gregorian calendar rules and handling any errors through exceptions.

Transcribed Image Text:### Date Validation Test Cases
This section reviews the date validation functionality through a series of test cases. Each test case presents an input date and compares the actual output with the expected outcome.
#### Test Case 1
- **Input:**
- `3/15/1625`
- **Actual Result:**
- The system outputs: `Enter date as mm/dd/yyyy: The date is 00/15/-1655662928`
- **Expected Result:**
- It should display: `Enter date as mm/dd/yyyy: The date is 03/15/1625`
- **Outcome:**
- **Fail**
#### Test Case 2
- **Input:**
- `2/29/1900`
- **Actual Result:**
- The system outputs: `Enter date as mm/dd/yyyy: The date is 00/29/-1611356496`
- **Expected Result:**
- It should display: `Enter date as mm/dd/yyyy: day (29) out of range`
- **Outcome:**
- **Fail**
#### Test Case 3
- **Input:**
- `2/29/2000`
- **Actual Result:**
- The system outputs: `Enter date as mm/dd/yyyy: The date is 00/29/-1579305296`
- **Expected Result:**
- It should display: `Enter date as mm/dd/yyyy: The date is 02/29/2000`
- **Outcome:**
- **Fail**
### Explanation of Diagram/Graph
There are no diagrams or graphs in this data set. The representation is solely based on text comparisons between expected and actual date processing results.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
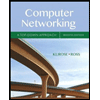
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
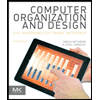
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
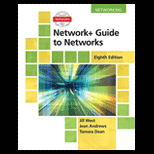
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
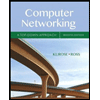
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
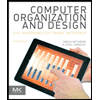
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
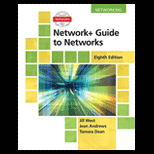
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
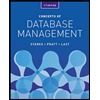
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
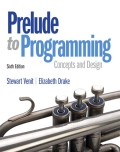
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
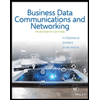
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY