Consider the same code, copied here for reference, but clearly with an array that has no valid sequence: int Length 10; = int Seq[] = {-10, 0, 1, 500, 7, 11, -34, 14, 1024, 18}; int Diff; int i, j; int main() { for (i=0; i
Consider the same code, copied here for reference, but clearly with an array that has no valid sequence: int Length 10; = int Seq[] = {-10, 0, 1, 500, 7, 11, -34, 14, 1024, 18}; int Diff; int i, j; int main() { for (i=0; i
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Please answer the question in C code
![## Detecting an Arithmetic Sequence in an Array
### Example Code
Consider the same code, copied here for reference, but clearly with an array that has no valid sequence:
```c
int Length = 10;
int Seq[] = {-10, 0, 1, 500, 7, 11, -34, 14, 1024, 18};
int Diff;
int i, j;
int main() {
for (i=0; i<Length-2; i++) {
Diff = Seq[i+1]-Seq[i]; // The difference, if it's an arithmetic sequence
for (j=i+1; j<Length-1; j++) {
if ( (Seq[j+1]-Seq[j]) != Diff) break; // Quit trying, if an element fails
}
if (j==9) {
printf("The arithmetic sequence starts at Seq[%d]\n", i);
break;
}
}
if (i==8) {
printf("There is no arithmetic sequence.\n");
}
return 0;
}
```
### Explanation
This code is designed to find if there is an arithmetic sequence in the given array `Seq`. An arithmetic sequence is a sequence of numbers with a constant difference between consecutive terms.
- **Outer Loop (`i` loop)**: This loop runs from the start of the array to the second-to-last element.
- **Inner Loop (`j` loop)**: This loop checks if all subsequent differences are the same as the initial difference calculated (`Diff`).
- If a differing element is found, the inner loop breaks, and the outer loop continues with the next starting index.
- If the loop completes without breaking prematurely, it indicates an arithmetic sequence starting at the current index.
### Missing Numbers Calculation
In the box below, type two numbers, representing what should be in the two blanks below (in the same order).
- The statement
```c
Diff = Seq[i+1]-Seq[i];
```
will be executed **8** times, and the statement
```c
if ( (Seq[j+1]-Seq[j]) != Diff)
```
will be executed **36** times.
This is because:
1. The outer loop runs `Length - 2` times, which is `10 - 2 = 8` times.
2. The inner loop](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F37ffeb4c-90e5-4cb9-a6b6-d76475059d8f%2F0d2305b6-95d0-4a25-b2f2-c24064cbe6ef%2Fbamwrco_processed.jpeg&w=3840&q=75)
Transcribed Image Text:## Detecting an Arithmetic Sequence in an Array
### Example Code
Consider the same code, copied here for reference, but clearly with an array that has no valid sequence:
```c
int Length = 10;
int Seq[] = {-10, 0, 1, 500, 7, 11, -34, 14, 1024, 18};
int Diff;
int i, j;
int main() {
for (i=0; i<Length-2; i++) {
Diff = Seq[i+1]-Seq[i]; // The difference, if it's an arithmetic sequence
for (j=i+1; j<Length-1; j++) {
if ( (Seq[j+1]-Seq[j]) != Diff) break; // Quit trying, if an element fails
}
if (j==9) {
printf("The arithmetic sequence starts at Seq[%d]\n", i);
break;
}
}
if (i==8) {
printf("There is no arithmetic sequence.\n");
}
return 0;
}
```
### Explanation
This code is designed to find if there is an arithmetic sequence in the given array `Seq`. An arithmetic sequence is a sequence of numbers with a constant difference between consecutive terms.
- **Outer Loop (`i` loop)**: This loop runs from the start of the array to the second-to-last element.
- **Inner Loop (`j` loop)**: This loop checks if all subsequent differences are the same as the initial difference calculated (`Diff`).
- If a differing element is found, the inner loop breaks, and the outer loop continues with the next starting index.
- If the loop completes without breaking prematurely, it indicates an arithmetic sequence starting at the current index.
### Missing Numbers Calculation
In the box below, type two numbers, representing what should be in the two blanks below (in the same order).
- The statement
```c
Diff = Seq[i+1]-Seq[i];
```
will be executed **8** times, and the statement
```c
if ( (Seq[j+1]-Seq[j]) != Diff)
```
will be executed **36** times.
This is because:
1. The outer loop runs `Length - 2` times, which is `10 - 2 = 8` times.
2. The inner loop
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
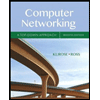
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
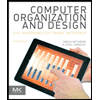
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
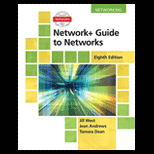
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
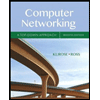
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
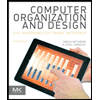
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
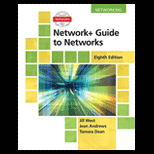
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
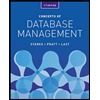
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
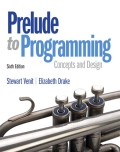
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
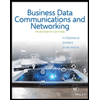
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY