Consider the salary of 14 employees [96315, 176629, 158648, 170033, 137309, 191467, 164221, 106223, 136536, 169858, 88486, 122077, 152924, 141851] Create a Pandas series for the above salary data with the list ['n01', 'n02', 'n03', 'n04', 'n05', 'n06', 'n07', 'n08', 'n09', 'n10', 'n11', 'n12', 'n13', 'n14'] as index. This must be done with a single line of code. Change the index of the series with the following names: ['Mary', 'John', 'Barbara', 'Richard', 'Elizabeth', 'Michael', 'Patricia', 'David', 'Jennifer', 'William', 'Linda', 'Robert', 'James', 'Susan'] Change the salary of Linda to 101000 using explicit index Determine whether George is in the series. Determine whether David is in the series. How many employees make more than 120K?
Consider the salary of 14 employees [96315, 176629, 158648, 170033, 137309, 191467, 164221, 106223, 136536, 169858, 88486, 122077, 152924, 141851]
Create a Pandas series for the above salary data with the list ['n01', 'n02', 'n03', 'n04', 'n05', 'n06', 'n07', 'n08', 'n09', 'n10', 'n11', 'n12', 'n13', 'n14'] as index. This must be done with a single line of code.
Change the index of the series with the following names: ['Mary', 'John', 'Barbara', 'Richard', 'Elizabeth', 'Michael', 'Patricia', 'David', 'Jennifer', 'William', 'Linda', 'Robert', 'James', 'Susan']
Change the salary of Linda to 101000 using explicit index
Determine whether George is in the series.
Determine whether David is in the series.
How many employees make more than 120K?

You can perform these operations using Python and Pandas as follows:
1import pandas as pd
2
3# Create a Pandas series with the given salary data and index
4salary_series = pd.Series([96315, 176629, 158648, 170033, 137309, 191467, 164221, 106223, 136536, 169858, 88486, 122077, 152924, 141851],
5 index=['n01', 'n02', 'n03', 'n04', 'n05', 'n06', 'n07', 'n08', 'n09', 'n10', 'n11', 'n12', 'n13', 'n14'])
6
7# Change the index of the series to the given names
8salary_series.index = ['Mary', 'John', 'Barbara', 'Richard', 'Elizabeth', 'Michael', 'Patricia', 'David', 'Jennifer', 'William', 'Linda', 'Robert', 'James', 'Susan']
9
10# Change the salary of Linda to 101000 using explicit index
11salary_series['Linda'] = 101000
12
13# Determine whether George is in the series
14is_george_present = 'George' in salary_series.index
15
16# Determine whether David is in the series
17is_david_present = 'David' in salary_series.index
18
19# Count the number of employees making more than 120K
20employees_above_120k = (salary_series > 120000).sum()
21
22# Print the results
23print("Salary Series:")
24print(salary_series)
25print("\nIs George in the series?", is_george_present)
26print("Is David in the series?", is_david_present)
27print("Number of employees making more than 120K:", employees_above_120k)
28
Step by step
Solved in 3 steps with 1 images

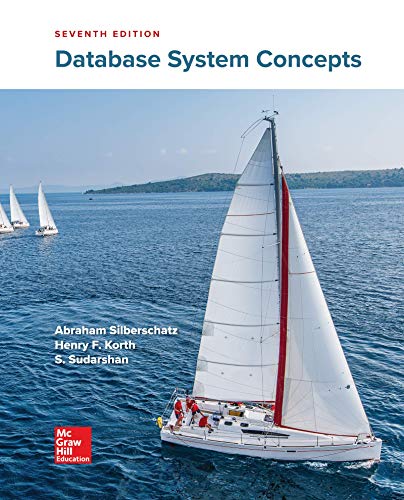
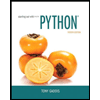
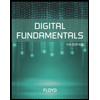
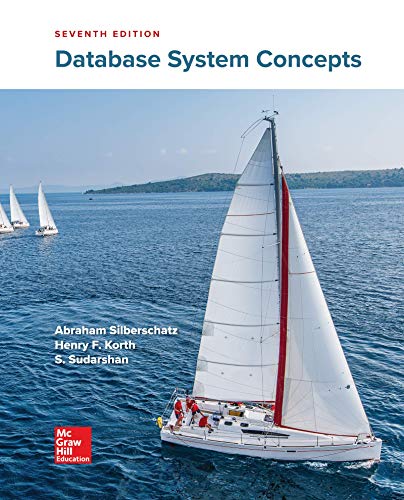
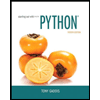
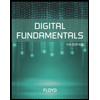
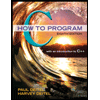
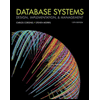
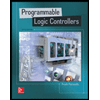