Consider the following simplified code for the Bubble sort algorithm.
Consider the following simplified code for the Bubble sort algorithm.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Step 1: Simplify your summation so that it is written as a polynomial. Show your work.
Step 2: State a big-Oh bound for your polynomial.
![### Bubble Sort Algorithm Complexity Analysis
**Consider the following simplified code for the Bubble sort algorithm:**
```cpp
for (int i = 0; i < list.size() - 1; i++) {
for (int j = 0; j < list.size() - 1; j++) {
if (list.get(j) > list.get(j + 1))
swap(j, j + 1);
}
}
```
---
In lecture, we explored running this procedure with an `ArrayList`. In this quiz problem, we will reconsider this procedure with a `LinkedList`. The pertinent difference lies in the cost of the `get`, `set`, and `swap` operations (assuming `swap` uses `get` and `set` as well). While in `ArrayLists` these operations take constant time, for `LinkedLists`, these operations take \( O(i) \) steps to reach index \( i \). In this worst case, we can see that this could be \( O(n) \).
Let's explore how this impacts the running time of this Bubble sort code.
---
### Performance Analysis
An upper bound on the number of operations carried out by this code can be expressed as a summation where \( n \) is the size of the list and where \( a \) and \( b \) are positive constants:
\[ \sum_{i=1}^n \left[ a + \sum_{j=1}^n bn \right] \]
*(For simplicity, some constants that do not impact the analysis have been eliminated.)*
---
In summary, we have analyzed the differences in performance between using an `ArrayList` and a `LinkedList` for the Bubble sort algorithm. The primary takeaway is that whereas the operations in an `ArrayList` take constant time, those in a `LinkedList` are linear with respect to the index, influencing the overall performance significantly.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8a000fe0-342d-4f80-8d89-1b56a9b64020%2Fb8c0200f-31d4-4869-bc33-4951fd8c0c74%2Fk450qwa_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Bubble Sort Algorithm Complexity Analysis
**Consider the following simplified code for the Bubble sort algorithm:**
```cpp
for (int i = 0; i < list.size() - 1; i++) {
for (int j = 0; j < list.size() - 1; j++) {
if (list.get(j) > list.get(j + 1))
swap(j, j + 1);
}
}
```
---
In lecture, we explored running this procedure with an `ArrayList`. In this quiz problem, we will reconsider this procedure with a `LinkedList`. The pertinent difference lies in the cost of the `get`, `set`, and `swap` operations (assuming `swap` uses `get` and `set` as well). While in `ArrayLists` these operations take constant time, for `LinkedLists`, these operations take \( O(i) \) steps to reach index \( i \). In this worst case, we can see that this could be \( O(n) \).
Let's explore how this impacts the running time of this Bubble sort code.
---
### Performance Analysis
An upper bound on the number of operations carried out by this code can be expressed as a summation where \( n \) is the size of the list and where \( a \) and \( b \) are positive constants:
\[ \sum_{i=1}^n \left[ a + \sum_{j=1}^n bn \right] \]
*(For simplicity, some constants that do not impact the analysis have been eliminated.)*
---
In summary, we have analyzed the differences in performance between using an `ArrayList` and a `LinkedList` for the Bubble sort algorithm. The primary takeaway is that whereas the operations in an `ArrayList` take constant time, those in a `LinkedList` are linear with respect to the index, influencing the overall performance significantly.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
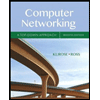
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
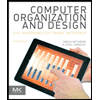
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
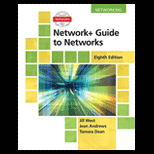
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
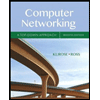
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
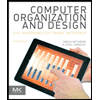
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
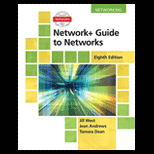
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
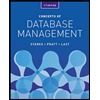
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
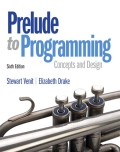
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
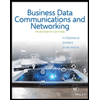
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY