Consider the following example depicted by UML diagram, where the Figure is an abstract class and CompareObjects is an interface receiving a generic data type T. Both classes Rectangle and Triangle have extended the abstract class Figure and implemented the interface CompareObjects. As a consequence, both the classes have to override the abstract methods area and compareObj. One example program to perform everything has been given below for your clear understanding. In the example program, class Rectangle has overridden the area method according to the formula for calculating the area of a Rectangle. It has implemented the CompareObjects interface and replaced the type T by Rectangle. So, the compareObj method receives another Rectangle object for comparison with the calling Rectangle object. Here, the compareObj method is overridden to compare two Rectangle objects based on their area. The compareObj method will return 0 if the two Rectangle objects have same area, 1 if the invoking object has higher area, otherwise -1. On the other hand, class Triangle has overridden the area method according to the formula for calculating the area of a Triangle. But it implemented the CompareObjectsinterface and replaced the type T by Figure. Hence, the compareObj method receives another Figure object (Triangle/Rectangle) for comparison with the calling Triangle object. Here, the compareObj method is overridden to compare two Triangle objects as well as one Triangle and one Rectangle objects based on their area. Demonstration of creation of all the objects and method calling are given inside the main method of the OverallAssignment class. Note that dynamic polymorphism has been shown through a reference of the parent abstract class. Output of this example program is also given at the end for understanding the result. Obviously, this example design and program have been used to demonstrate, and you cannot use this as your solution. Your task will be to design and implement any real-life example similar to the following example with meaningful relationship. Take care of the following I. Design at least one abstract class with at least one variable and one abstract method. II. Design at least one interface with at least one method. III. Declare at least two child classes that extend the abstract class and implement the interface. IV. Create another class (beside these classes) and the main method inside it. Declare necessary references and objects, and call methods to show dynamic polymorphism and other operations. V. Submit the complete code in a single doc file. Submission of the UML diagram of your design is not mandatory. However, if you want, you can put your UML diagram at the beginning of your doc file. +Rectangle(a:double, b:double) Rectangle Triangle +Triangle(a: double, b: double) Figure #dim1: double +Figure(a:double, b:double) +area(): double #dim2: double <> +compareObj(obj:T): int CompareObjects abstract class Figure{ protected double dim1, dim2; public Figure(double a,double b){ dim1 = a; dim2 = b; } public abstract double area(); } interface CompareObjects { int compareObj(T obj); } class Rectangle extends Figure implements CompareObjects { public Rectangle(double a, double b) { super(a, b); } @Override public double area() { return dim1*dim2; } @Override public int compareObj(Rectangle obj) { if(area() == obj.area()) return 0; else if (area() > obj.area()) return 1; else return -1; } } class Triangle extends Figure implements CompareObjects { public Triangle(double a, double b) { super(a, b); } @Override public double area() { return 0.5*dim1*dim2; } @Override public int compareObj(Figure obj) { if(area() == obj.area()) return 0; else if (area() > obj.area()) return 1; else return -1; } } public class OverallAssignment { public static void main(String[] args) { Figure figRef; Rectangle rec1 = new Rectangle(5,7); Rectangle rec2 = new Rectangle(8,3); figRef = rec1; System.out.println(figRef.area()); System.out.println(rec1.compareObj(rec2)); Triangle tr1 = new Triangle(4,10); Triangle tr2 = new Triangle(5,8); figRef = tr1; System.out.println(figRef.area()); System.out.println(tr1.compareObj(tr2)); System.out.println(tr1.compareObj(rec1)); } } This is a sample diagram and code don't use it in your code.make you own idea which is related to real life then code it in java.Thanks
Consider the following example depicted by UML diagram, where the Figure is an abstract class and
CompareObjects is an interface receiving a generic data type T. Both classes Rectangle and Triangle have
extended the abstract class Figure and implemented the interface CompareObjects. As a consequence, both
the classes have to override the abstract methods area and compareObj. One example program to perform
everything has been given below for your clear understanding.
In the example program, class Rectangle has overridden the area method according to the formula for
calculating the area of a Rectangle. It has implemented the CompareObjects interface and replaced the type T
by Rectangle. So, the compareObj method receives another Rectangle object for comparison with the calling
Rectangle object. Here, the compareObj method is overridden to compare two Rectangle objects based on
their area. The compareObj method will return 0 if the two Rectangle objects have same area, 1 if the invoking
object has higher area, otherwise -1.
On the other hand, class Triangle has overridden the area method according to the formula for calculating the
area of a Triangle. But it implemented the CompareObjectsinterface and replaced the type T by Figure. Hence,
the compareObj method receives another Figure object (Triangle/Rectangle) for comparison with the calling
Triangle object. Here, the compareObj method is overridden to compare two Triangle objects as well as one
Triangle and one Rectangle objects based on their area. Demonstration of creation of all the objects and
method calling are given inside the main method of the OverallAssignment class. Note that dynamic
polymorphism has been shown through a reference of the parent abstract class. Output of this example
program is also given at the end for understanding the result. Obviously, this example design and program
have been used to demonstrate, and you cannot use this as your solution.
Your task will be to design and implement any real-life example similar to the following example with
meaningful relationship. Take care of the following
I. Design at least one abstract class with at least one variable and one abstract method.
II. Design at least one interface with at least one method.
III. Declare at least two child classes that extend the abstract class and implement the interface.
IV. Create another class (beside these classes) and the main method inside it. Declare necessary
references and objects, and call methods to show dynamic polymorphism and other operations.
V. Submit the complete code in a single doc file. Submission of the UML diagram of your design is not
mandatory. However, if you want, you can put your UML diagram at the beginning of your doc file.
+Rectangle(a:double, b:double)
Rectangle Triangle
+Triangle(a: double, b: double)
Figure
#dim1: double
+Figure(a:double, b:double)
+area(): double
#dim2: double <<interface>>
+compareObj(obj:T): int
CompareObjects<T>
abstract class Figure{
protected double dim1, dim2;
public Figure(double a,double b){
dim1 = a;
dim2 = b;
}
public abstract double area();
}
interface CompareObjects<T> {
int compareObj(T obj);
}
class Rectangle extends Figure implements CompareObjects<Rectangle> {
public Rectangle(double a, double b) {
super(a, b);
}
@Override
public double area() {
return dim1*dim2;
}
@Override
public int compareObj(Rectangle obj) {
if(area() == obj.area()) return 0;
else if (area() > obj.area()) return 1;
else return -1;
}
}
class Triangle extends Figure implements CompareObjects<Figure> {
public Triangle(double a, double b) {
super(a, b);
}
@Override
public double area() {
return 0.5*dim1*dim2;
}
@Override
public int compareObj(Figure obj) {
if(area() == obj.area()) return 0;
else if (area() > obj.area()) return 1;
else return -1;
}
}
public class OverallAssignment {
public static void main(String[] args) {
Figure figRef;
Rectangle rec1 = new Rectangle(5,7);
Rectangle rec2 = new Rectangle(8,3);
figRef = rec1;
System.out.println(figRef.area());
System.out.println(rec1.compareObj(rec2));
Triangle tr1 = new Triangle(4,10);
Triangle tr2 = new Triangle(5,8);
figRef = tr1;
System.out.println(figRef.area());
System.out.println(tr1.compareObj(tr2));
System.out.println(tr1.compareObj(rec1));
}
}
This is a sample diagram and code don't use it in your code.make you own idea which is related to real life then code it in java.Thanks

![abstract class Figure{
protected double dim1, dim2;
public Figure(double a,double b){
dim1 = a;
sample
code
dim2 = b;
}
public abstract double area();
}
interface CompareObjects<T> {
int compareObj(T obj);
}
class Rectangle extends Figure implements CompareObjects<Rectangle> {
public Rectangle(double a, double b) {
super (a, b);
}
@Override
public double area() {
return dim1*dim2;
}
@Override
public int compareObj(Rectangle obj) {
if(area() == obj.area()) return 0;
else if (area() > obj.area()) return 1;
else return -1;
}
}
class Triangle extends Figure implements CompareObjects<Figure> {
public Triangle(double a, double b) {
super (a, b);
}
@Override
public double area() {
return 0.5*dim1*dim2;
}
@Override
public int compareObj(Figure obj) {
if(area() == obj.area()) return 0;
else if (area() > obj.area()) return 1;
else return -1;
}
}
public class OverallAssignment {
public static void main(String[] args) {
Figure figRef;
Rectangle rec1 = new Rectangle(5,7);
Rectangle rec2 = new Rectangle(8,3);
figRef = rec1;
System.out.println(figRef.area());
System.out.println(rec1.compareObj(rec2));
Triangle tr1 = new Triangle(4,10);
Triangle tr2 = new Triangle(5,8);
figRef
System.out.println(figRef.area());
System.out.println(tr1.compareObj(tr2));
System.out.println(tr1.compareObj(rec1));
= tr1;
}
}
OutPut:
35.0
1
20.0
-1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb087153c-b059-41a1-beeb-2fa8c3154d98%2Fded5413c-bd23-4eae-9c45-7c9f4e088aec%2Fpdo4rc_processed.jpeg&w=3840&q=75)

Step by step
Solved in 2 steps with 1 images

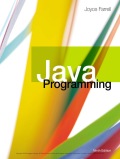
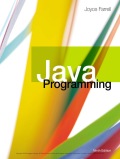