Consider the following equations. 5 Σ(3i – 2)2 i=1 = 1 import math 2 3 def iii(stop): = n = i=1 (3i - 2)² n i=1 23 cos(x) cos(2x)...cos(2n-1x) for the last expression n = 1, 2, 3, ... and sin(x) = 0. Some helpful background: Before we move ahead, note that equation-32, 33 and 34 are examples of analytical (closed from) solution. What it means is that, you can either solve it via the formula as shown in equations 32-34, or you can calculate each term individually, but the two solutions will match. Isn't that amazing that some people figured out that rather than calculating and adding each term in a loop, you can directly get the final result just by plugging the values in the formula :). Fasinating as it is to some of us, the analytical solutions are not available for all problems. We give them here for you to check that both loop based (going over each term) and the closed form solutions mathch. Equation-35 is the loop based calculation (for n = 5), and equation-37 is the direct result by plugging the value in the formula of equation-32. (6n²-3m = 3n-1) (n²(n + 1)² sin (2x) 2n sin(x) (3 − 2)² + (6 − 2)² + (9 − 2)² + (12 − 2)² + (15 − 2)² (32) 1² +4² +7² +10² + 13² = 1 + 16 +49 +100+ 169 = 335 (1/2)5(6(5²) – (3(5)) − 1) = (5/2)(150 – 16) = 335 (33) (34) (35) (36) (37) Complete the code below that uses a loop to calculate the sum (the closed-form is included to help you validate your code)
Consider the following equations. 5 Σ(3i – 2)2 i=1 = 1 import math 2 3 def iii(stop): = n = i=1 (3i - 2)² n i=1 23 cos(x) cos(2x)...cos(2n-1x) for the last expression n = 1, 2, 3, ... and sin(x) = 0. Some helpful background: Before we move ahead, note that equation-32, 33 and 34 are examples of analytical (closed from) solution. What it means is that, you can either solve it via the formula as shown in equations 32-34, or you can calculate each term individually, but the two solutions will match. Isn't that amazing that some people figured out that rather than calculating and adding each term in a loop, you can directly get the final result just by plugging the values in the formula :). Fasinating as it is to some of us, the analytical solutions are not available for all problems. We give them here for you to check that both loop based (going over each term) and the closed form solutions mathch. Equation-35 is the loop based calculation (for n = 5), and equation-37 is the direct result by plugging the value in the formula of equation-32. (6n²-3m = 3n-1) (n²(n + 1)² sin (2x) 2n sin(x) (3 − 2)² + (6 − 2)² + (9 − 2)² + (12 − 2)² + (15 − 2)² (32) 1² +4² +7² +10² + 13² = 1 + 16 +49 +100+ 169 = 335 (1/2)5(6(5²) – (3(5)) − 1) = (5/2)(150 – 16) = 335 (33) (34) (35) (36) (37) Complete the code below that uses a loop to calculate the sum (the closed-form is included to help you validate your code)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
THIS IS A PYTHON HOMEWORK
![## Consider the Following Equations
### Equations:
\[
\sum_{i=1}^{n} (3i - 2)^2 = \frac{1}{2}n(6n^2 - 3n - 1) \quad (32)
\]
\[
\sum_{i=1}^{n} i^3 = \frac{1}{4}n^2(n + 1)^2 \quad (33)
\]
\[
\cos(x) \cdot \cos(2x) \cdots \cos(2^{n-1}x) = \frac{\sin(2^n x)}{2^n \sin(x)} \quad (34)
\]
For the last expression, \( n = 1, 2, 3, \ldots \) and \(\sin(x) \neq 0\).
### Some Helpful Background:
Before we move ahead, note that equations 32, 33, and 34 are examples of analytical (closed-form) solutions. This means that you can either solve them via the formulas as shown or calculate each term individually, and both solutions will match. Isn’t it amazing that some people figured out that rather than calculating and adding each term in a loop, you can directly get the final result by plugging the values in the formula?
While this is fascinating to some of us, analytical solutions are not available for all problems. These examples are provided to help check that both loop-based (calculating each term iteratively) and closed-form solutions match. Equations 35 to 37 demonstrate this for \( n = 5 \).
### Loop-Based Calculation:
\[
\sum_{i=1}^{5} (3i - 2)^2 = (3 - 2)^2 + (6 - 2)^2 + (9 - 2)^2 + (12 - 2)^2 + (15 - 2)^2 \quad (35)
\]
Breaking it down:
\[
= 1^2 + 4^2 + 7^2 + 10^2 + 13^2 = 1 + 16 + 49 + 100 + 169 = 335 \quad (36)
\]
### Closed-Form Solution:
\[
= (1/2)5(6(5^2) - 3(5)) - 1 = (5](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1d5e88e6-b1af-4aea-9b08-2dadd85f5e2c%2Fd6df8bb2-7412-439a-8d9f-df1a1fa573d9%2Fcw25v3e0p_processed.png&w=3840&q=75)
Transcribed Image Text:## Consider the Following Equations
### Equations:
\[
\sum_{i=1}^{n} (3i - 2)^2 = \frac{1}{2}n(6n^2 - 3n - 1) \quad (32)
\]
\[
\sum_{i=1}^{n} i^3 = \frac{1}{4}n^2(n + 1)^2 \quad (33)
\]
\[
\cos(x) \cdot \cos(2x) \cdots \cos(2^{n-1}x) = \frac{\sin(2^n x)}{2^n \sin(x)} \quad (34)
\]
For the last expression, \( n = 1, 2, 3, \ldots \) and \(\sin(x) \neq 0\).
### Some Helpful Background:
Before we move ahead, note that equations 32, 33, and 34 are examples of analytical (closed-form) solutions. This means that you can either solve them via the formulas as shown or calculate each term individually, and both solutions will match. Isn’t it amazing that some people figured out that rather than calculating and adding each term in a loop, you can directly get the final result by plugging the values in the formula?
While this is fascinating to some of us, analytical solutions are not available for all problems. These examples are provided to help check that both loop-based (calculating each term iteratively) and closed-form solutions match. Equations 35 to 37 demonstrate this for \( n = 5 \).
### Loop-Based Calculation:
\[
\sum_{i=1}^{5} (3i - 2)^2 = (3 - 2)^2 + (6 - 2)^2 + (9 - 2)^2 + (12 - 2)^2 + (15 - 2)^2 \quad (35)
\]
Breaking it down:
\[
= 1^2 + 4^2 + 7^2 + 10^2 + 13^2 = 1 + 16 + 49 + 100 + 169 = 335 \quad (36)
\]
### Closed-Form Solution:
\[
= (1/2)5(6(5^2) - 3(5)) - 1 = (5
![```python
4 def closed(n):
5 return (1/4)*(n**2)*(n+1)**2
6 sum = 0
7 #complete bounded loop here
8 return [sum,closed(stop)]
9
10 def iv(stop):
11 def closed(n):
12 return (1/2)*n*(6*(n**2)-(3*n)-1)
13 sum = 0
14 #complete bounded loop here
15 return [sum, closed(stop)]
16
17 def vi(x,stop):
18 def closed(x,n):
19 return math.sin(x+(2**n))/((2**n)*math.sin(x))
20 prod = 1
21 #complete bounded loop here
22 return [prod,closed(x,stop)]
23
24 print(iii(5))
25 print(iv(5))
26 print(vi(math.pi,5))
```
**Explanation:**
This Python code contains three functions: `closed`, `iv`, and `vi`, each designed to calculate a mathematical expression based on the given parameters and return a list of values. The functions `iv` and `vi` contain nested functions also named `closed`.
- **Function `closed(n)`**:
- Computes an expression using the formula \((1/4) \cdot (n^2) \cdot (n+1)^2\).
- Returns a list containing the sum (uninitialized loop) and the result of the expression with `n` equal to `stop`.
- **Function `iv(stop)`**:
- Contains a nested function `closed(n)` which computes a different formula: \((1/2) \cdot n \cdot (6 \cdot (n^2) - (3 \cdot n) - 1)\).
- Returns a list with the sum (uninitialized loop) and the result of the nested `closed(stop)`.
- **Function `vi(x, stop)`**:
- Contains a nested function `closed(x, n)` which calculates using trigonometric functions: \(\frac{\sin(x+(2^n))}{((2^n) \cdot \sin(x))}\).
- Returns a list with the product (uninitialized loop) and the result of `closed(x, stop)`.
Print statements show outputs](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1d5e88e6-b1af-4aea-9b08-2dadd85f5e2c%2Fd6df8bb2-7412-439a-8d9f-df1a1fa573d9%2Fz17l3wf_processed.png&w=3840&q=75)
Transcribed Image Text:```python
4 def closed(n):
5 return (1/4)*(n**2)*(n+1)**2
6 sum = 0
7 #complete bounded loop here
8 return [sum,closed(stop)]
9
10 def iv(stop):
11 def closed(n):
12 return (1/2)*n*(6*(n**2)-(3*n)-1)
13 sum = 0
14 #complete bounded loop here
15 return [sum, closed(stop)]
16
17 def vi(x,stop):
18 def closed(x,n):
19 return math.sin(x+(2**n))/((2**n)*math.sin(x))
20 prod = 1
21 #complete bounded loop here
22 return [prod,closed(x,stop)]
23
24 print(iii(5))
25 print(iv(5))
26 print(vi(math.pi,5))
```
**Explanation:**
This Python code contains three functions: `closed`, `iv`, and `vi`, each designed to calculate a mathematical expression based on the given parameters and return a list of values. The functions `iv` and `vi` contain nested functions also named `closed`.
- **Function `closed(n)`**:
- Computes an expression using the formula \((1/4) \cdot (n^2) \cdot (n+1)^2\).
- Returns a list containing the sum (uninitialized loop) and the result of the expression with `n` equal to `stop`.
- **Function `iv(stop)`**:
- Contains a nested function `closed(n)` which computes a different formula: \((1/2) \cdot n \cdot (6 \cdot (n^2) - (3 \cdot n) - 1)\).
- Returns a list with the sum (uninitialized loop) and the result of the nested `closed(stop)`.
- **Function `vi(x, stop)`**:
- Contains a nested function `closed(x, n)` which calculates using trigonometric functions: \(\frac{\sin(x+(2^n))}{((2^n) \cdot \sin(x))}\).
- Returns a list with the product (uninitialized loop) and the result of `closed(x, stop)`.
Print statements show outputs
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
you do not do anything with this question, the question ask you to complete all bounded loop in different function.
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
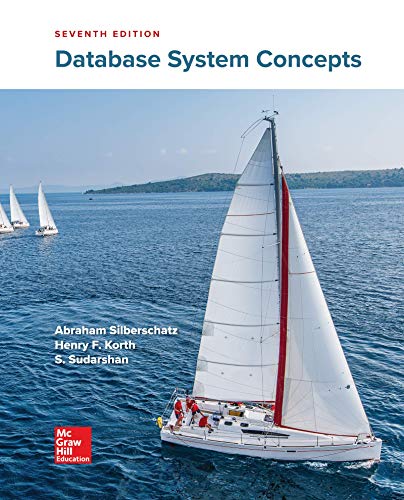
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
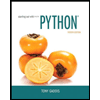
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
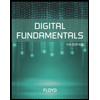
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
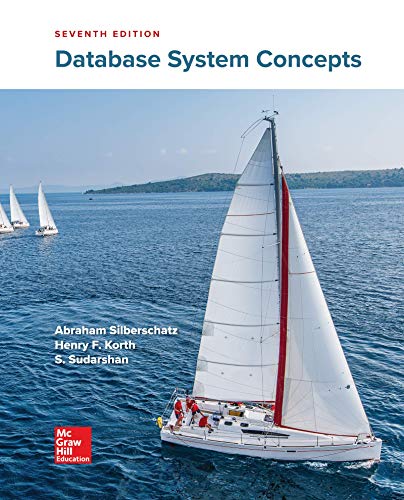
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
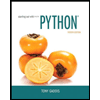
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
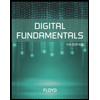
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
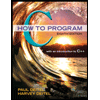
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
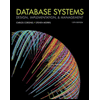
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
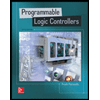
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education