Consider the following C declaration and assume that the machine has 1-byte characters, 2-byte short, 4-byte integers, 4-byte single-precision floating point numbers, 4-byte pointers, 8-byte double- precision floating-point numbers, and the compiler does not reorder fields and leaves no memory gaps. You may consider all other data types as 8-byte. union UI ( int value; char code; double data; void pointer; }; union U2 ( float func; short small [2]; }; union U3 ( B; char text; long long number; }; union U4 ( char name [6]; unsigned int id; }; struct S ( int index; union Ui item; union U4 person; void next;
Consider the following C declaration and assume that the machine has 1-byte characters, 2-byte short, 4-byte integers, 4-byte single-precision floating point numbers, 4-byte pointers, 8-byte double- precision floating-point numbers, and the compiler does not reorder fields and leaves no memory gaps. You may consider all other data types as 8-byte. union UI ( int value; char code; double data; void pointer; }; union U2 ( float func; short small [2]; }; union U3 ( B; char text; long long number; }; union U4 ( char name [6]; unsigned int id; }; struct S ( int index; union Ui item; union U4 person; void next;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Topic Video
Question
100%
![**Educational Text on C Declaration and Memory Layout**
---
Consider the following C declaration and assume the following for the machine:
- **1-byte characters**
- **2-byte shorts**
- **4-byte integers**
- **4-byte single-precision floating-point numbers**
- **4-byte pointers**
- **8-byte double-precision floating-point numbers**
The compiler does not reorder fields and leaves no memory gaps. All other data types are considered as 8-byte:
```c
union U1 {
int value;
char code;
double data;
void* pointer;
};
union U2 {
float func;
short small[2];
};
union U3 {
char text;
long long number;
};
union U4 {
char name[6];
unsigned int id;
};
struct S {
int index;
union U1 item;
union U4 person;
void* next;
} s;
```
**Questions to Consider:**
1. **How many bytes does each `union` declared in the code and `struct S` occupy?**
2. **If the memory address of `S` starts from 3000, what are the start addresses of `S.item.pointer` and `S.person.name[2]`?**
3. **If the compiler uses a simple memory alignment mechanism that aligns variables to 8-byte boundaries, what will be the size of `S`?**
---
**Explanation and Analysis:**
1. **Union Sizes:**
- Each union is sized according to its largest member.
- `union U1`: Largest member is `double data` (8 bytes).
- `union U2`: Largest member is `func` or `small[2]` (4 bytes each, totaling 8 bytes).
- `union U3`: Largest member is `number` (8 bytes).
- `union U4`: Largest member is `id` (4 bytes, but in practice unions are aligned to larger sizes).
2. **Struct S Size:**
- Includes size of `index` (4 bytes), `union U1` (8 bytes), `union U4` (aligned to 8 bytes), and `next` (4 bytes).
- Alignment adjustments may lead to additional padding.
3. **Address Calculations:**
- Given starting address 3000, calculate addresses based on sizes and alignments.
This exercise emphasizes understanding memory](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F12050043-4d02-4d31-b9b4-7dfbc30a9c5d%2F35c4bcc0-116c-48eb-9125-403ca76ef2c7%2F1pv52w6_processed.png&w=3840&q=75)
Transcribed Image Text:**Educational Text on C Declaration and Memory Layout**
---
Consider the following C declaration and assume the following for the machine:
- **1-byte characters**
- **2-byte shorts**
- **4-byte integers**
- **4-byte single-precision floating-point numbers**
- **4-byte pointers**
- **8-byte double-precision floating-point numbers**
The compiler does not reorder fields and leaves no memory gaps. All other data types are considered as 8-byte:
```c
union U1 {
int value;
char code;
double data;
void* pointer;
};
union U2 {
float func;
short small[2];
};
union U3 {
char text;
long long number;
};
union U4 {
char name[6];
unsigned int id;
};
struct S {
int index;
union U1 item;
union U4 person;
void* next;
} s;
```
**Questions to Consider:**
1. **How many bytes does each `union` declared in the code and `struct S` occupy?**
2. **If the memory address of `S` starts from 3000, what are the start addresses of `S.item.pointer` and `S.person.name[2]`?**
3. **If the compiler uses a simple memory alignment mechanism that aligns variables to 8-byte boundaries, what will be the size of `S`?**
---
**Explanation and Analysis:**
1. **Union Sizes:**
- Each union is sized according to its largest member.
- `union U1`: Largest member is `double data` (8 bytes).
- `union U2`: Largest member is `func` or `small[2]` (4 bytes each, totaling 8 bytes).
- `union U3`: Largest member is `number` (8 bytes).
- `union U4`: Largest member is `id` (4 bytes, but in practice unions are aligned to larger sizes).
2. **Struct S Size:**
- Includes size of `index` (4 bytes), `union U1` (8 bytes), `union U4` (aligned to 8 bytes), and `next` (4 bytes).
- Alignment adjustments may lead to additional padding.
3. **Address Calculations:**
- Given starting address 3000, calculate addresses based on sizes and alignments.
This exercise emphasizes understanding memory
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
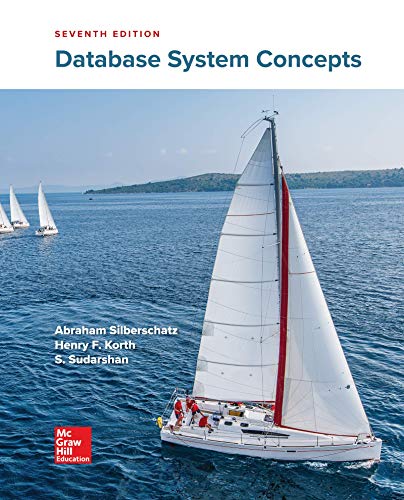
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
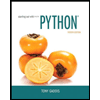
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
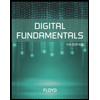
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
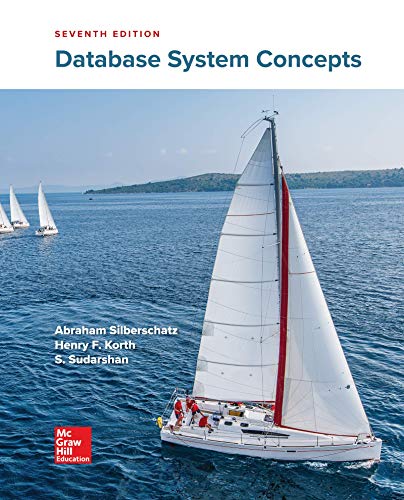
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
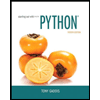
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
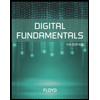
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
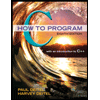
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
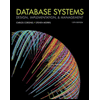
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
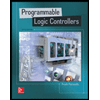
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education