Consider the following array declaration, written for a 16 bit processor If the starting address of sample_datais 0x2800, at what address is sample_data[150]located? Show your work.
Consider the following array declaration, written for a 16 bit processor If the starting address of sample_datais 0x2800, at what address is sample_data[150]located? Show your work.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Consider the following array declaration, written for a 16 bit processor
If the starting address of sample_datais 0x2800, at what address is sample_data[150]located? Show your work.
![### Example Code for Data Sampling in Embedded Systems
Below is a simple example of a C program for an embedded system. This program initializes an array and continuously collects data from a port, storing it in the array:
```c
void main(void) {
int idx = 0;
int sample_data[512];
while(1) {
sample_data[idx++] = P3IN & 0x0F;
// . . .
}
}
```
#### Explanation
1. **Function Declaration:**
```c
void main(void) {
```
- The `main` function is the entry point of the program. `void` indicates that it does not return a value.
2. **Variable Initialization:**
```c
int idx = 0;
int sample_data[512];
```
- An integer `idx` is initialized to `0`. It will be used as an index for the array.
- An integer array `sample_data` of size `512` is declared to store sampled data.
3. **Infinite Loop:**
```c
while(1) {
```
- The `while(1)` loop runs indefinitely, representing continuous data collection.
4. **Data Sampling:**
```c
sample_data[idx++] = P3IN & 0x0F;
```
- `P3IN & 0x0F` reads data from port `P3IN` and performs a bitwise AND with `0x0F` to mask the high 4 bits, effectively only retaining the lower 4 bits.
- The result is stored in the `sample_data` array at the current index `idx`.
- The index `idx` is incremented after each read.
5. **Comment Placeholder:**
```c
// . . .
```
- Indicates where additional code or comments might be added.
This simple code structure is typically used for data acquisition in embedded systems, where continuous monitoring and sampling are required.
#### Diagram Explanation
There are no graphs or diagrams in the provided image. However, if we were to create a flowchart for this program, it would look something like this:
1. **Start**
2. **Initialize Index (idx = 0)**
3. **Initialize Sample Data Array [512]**
4. **Enter Infinite Loop**
- **Read Port Data (P](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8cc8c426-b479-4d0a-8eda-aecb8e16874c%2F5cea9ce6-bc58-45d9-8472-9d5204462642%2F62rjai_processed.png&w=3840&q=75)
Transcribed Image Text:### Example Code for Data Sampling in Embedded Systems
Below is a simple example of a C program for an embedded system. This program initializes an array and continuously collects data from a port, storing it in the array:
```c
void main(void) {
int idx = 0;
int sample_data[512];
while(1) {
sample_data[idx++] = P3IN & 0x0F;
// . . .
}
}
```
#### Explanation
1. **Function Declaration:**
```c
void main(void) {
```
- The `main` function is the entry point of the program. `void` indicates that it does not return a value.
2. **Variable Initialization:**
```c
int idx = 0;
int sample_data[512];
```
- An integer `idx` is initialized to `0`. It will be used as an index for the array.
- An integer array `sample_data` of size `512` is declared to store sampled data.
3. **Infinite Loop:**
```c
while(1) {
```
- The `while(1)` loop runs indefinitely, representing continuous data collection.
4. **Data Sampling:**
```c
sample_data[idx++] = P3IN & 0x0F;
```
- `P3IN & 0x0F` reads data from port `P3IN` and performs a bitwise AND with `0x0F` to mask the high 4 bits, effectively only retaining the lower 4 bits.
- The result is stored in the `sample_data` array at the current index `idx`.
- The index `idx` is incremented after each read.
5. **Comment Placeholder:**
```c
// . . .
```
- Indicates where additional code or comments might be added.
This simple code structure is typically used for data acquisition in embedded systems, where continuous monitoring and sampling are required.
#### Diagram Explanation
There are no graphs or diagrams in the provided image. However, if we were to create a flowchart for this program, it would look something like this:
1. **Start**
2. **Initialize Index (idx = 0)**
3. **Initialize Sample Data Array [512]**
4. **Enter Infinite Loop**
- **Read Port Data (P
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
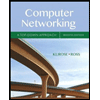
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
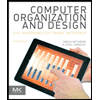
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
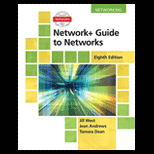
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
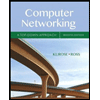
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
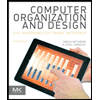
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
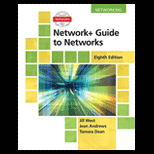
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
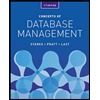
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
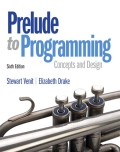
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
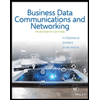
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY