Consider a program to determine whether a given string of parentheses (single type) is properly nested. A string 'S' consisting of 'N' characters is called properly nested if: 'S' is empty; 'S' has the form "(U)" where 'U' is a properly nested string; 'S' has the form "VW" where 'V' and 'W' are properly nested strings. For example, string "(()(())())" is properly nested but string "())" isn't. String 'S' consists only of the characters "(" and/or ")". Note : Use push() to add the parenthesis to the stack and pop() function to remove the parenthesis from the stack Input Format: The first line of input is a string. Output Format : The output is 0 if parenthesis is nested in balance or 1 if parenthesis is not nested in balance, based on the input string. Sample Input 1: (()(())()) Sample Output 1: 1 Sample Input 2: (()) Sample Output 2: 0 ------------Program Below---------------- main.cpp ( place to fill at no 11, 13 , 24 ) 1 #include 2 #include 3 #include 4 #include "Stack.cpp" 5 using namespace std; 6 string checkBalance(string str) { 7 Stack s; 8 for (int i = 0; i < str.length(); i++) { 9 char ch = str[i]; 10 if (ch == '(') { 11 s._______(ch); 12 } else if ((ch == ')') && (!s.empty())) { 13 if ((char) s._________() == '(' && ch == ')') { 14 s.pop(); 15 } else { 16 return "0"; 17 } 18 } else { 19 if ( ch == ')') { 20 return "0"; 21 } 22 } 23 } 24 if (s._____________()) 25 return "1"; 26 else 27 return "0"; 28 }; 29 int main() 30 { 31 string str; 32 cin >> str; 33 cout << checkBalance(str); 34 }; stack.cpp ( place to fill at no 21, 28 ) 1 #include 2 using namespace std; 3 class Stack { 4 private: 5 char data[30]; 6 int index = 0; 7 int size = 0; 8 public: 9 void push(char c) { 10 if (index >= size) { 11 char temp[size]; 12 size = size * 2; 13 for (int i = 0; i < index; i++) { 14 temp[i] = this->data[i]; 15 } 16 memset(data, 0, sizeof(data)); 17 for (int i = 0; i < index; i++) { 18 this->data[i] = temp[i]; 19 } 20 } 21 this->data[index] = _____________; 22 index++; 23 } 24 char pop() { 25 if (index != 0) { 26 char c = data[index - 1]; 27 this->data[index - 1] = '\0'; // Deleted 28 ______________ ; 29 return c; 30 } else 31 return '\0'; 32 } 33 char peek() { 34 try{ 35 if (index != 0) 36 return this->data[index - 1]; 37 else 38 return '\0'; 39 throw (1); 40 } 41 catch (int myNum) { 42 cout << "Exception occured"; 43 } 44 } 45 bool empty() { 46 return index == 0 ? true : false; 47 } 48 };
Given a problem and program, fillup the blank spaces in the program in order to make the program work correctly.
Problem below :-
Consider a program to determine whether a given string of parentheses (single type) is properly nested. A string 'S' consisting of 'N' characters is called properly nested if: Note : Input Format: Output Format : Sample Input 1: Sample Output 1: Sample Input 2: Sample Output 2: |
------------Program Below----------------
main.cpp ( place to fill at no 11, 13 , 24 )
1 #include<cstring> 2 #include<iostream> 3 #include<string> 4 #include "Stack.cpp" 5 using namespace std; 6 string checkBalance(string str) { 7 Stack s; 8 for (int i = 0; i < str.length(); i++) { 9 char ch = str[i]; 10 if (ch == '(') { 11 s._______(ch); 12 } else if ((ch == ')') && (!s.empty())) { 13 if ((char) s._________() == '(' && ch == ')') { 14 s.pop(); 15 } else { 16 return "0"; 17 } 18 } else { 19 if ( ch == ')') { 20 return "0"; 21 } 22 } 23 } 24 if (s._____________()) 25 return "1"; 26 else 27 return "0"; 28 }; 29 int main() 30 { 31 string str; 32 cin >> str; 33 cout << checkBalance(str); 34 }; |
stack.cpp ( place to fill at no 21, 28 )
1 #include <bits/stdc++.h> 2 using namespace std; 3 class Stack { 4 private: 5 char data[30]; 6 int index = 0; 7 int size = 0; 8 public: 9 void push(char c) { 10 if (index >= size) { 11 char temp[size]; 12 size = size * 2; 13 for (int i = 0; i < index; i++) { 14 temp[i] = this->data[i]; 15 } 16 memset(data, 0, sizeof(data)); 17 for (int i = 0; i < index; i++) { 18 this->data[i] = temp[i]; 19 } 20 } 21 this->data[index] = _____________; 22 index++; 23 } 24 char pop() { 25 if (index != 0) { 26 char c = data[index - 1]; 27 this->data[index - 1] = '\0'; // Deleted 28 ______________ ; 29 return c; 30 } else 31 return '\0'; 32 } 33 char peek() { 34 try{ 35 if (index != 0) 36 return this->data[index - 1]; 37 else 38 return '\0'; 39 throw (1); 40 } 41 catch (int myNum) { 42 cout << "Exception occured"; 43 } 44 } 45 bool empty() { 46 return index == 0 ? true : false; 47 } 48 }; |

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

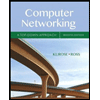
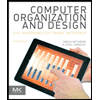
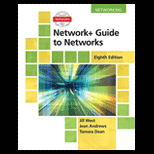
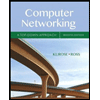
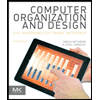
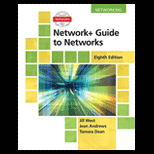
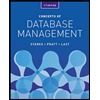
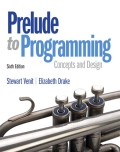
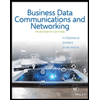