Certainly! Here is the transcription of the code shown in the image: --- ```cpp #include #include int main() { linkedStackType stack1; linkedStackType stack2; int x; // Prompt user for stack elements cout << "Enter elements with indicating the end of the element (with -999)" << endl; // Add the elements to Stack 1 while(1){ cin >> x; if(x == -999) break; stack1.push(x); } stack2 = stack1; // Compare Stack 1 and Stack 2 if(stack1 == stack2){ cout << "Stack1 and Stack2 are identical" << endl; } else { cout << "Stack1 and Stack2 are not identical" << endl; } cout << endl; // Reverse the order of Stack 1 stack1.reverseStack(stack2); if(stack1 == stack2){ cout << "After reverse, the Stack1 and Stack2 are still identical" << endl; } else { cout << "After reverse, the Stack1 and Stack2 are not identical" << endl; } cout << "--------------------------------------------------------" << endl; cout << "The Stack 1 items: " << endl; cout << "--------------------------------------------------------" << endl; // Print the elements of Stack 1 } ``` **Explanation:** - The program includes a stack implementation using the library `linkedStack.h` and standard `iostream`. - Two stacks, `stack1` and `stack2`, are created using a linked stack type for integers. - User is prompted to input elements into `stack1` until they enter `-999`, which indicates the end of inputs. - `stack2` is assigned the same elements as `stack1`. - The program checks if the two stacks are identical and outputs the result. - The order of elements in `stack1` is then reversed. - It checks again if the stacks are identical after the reversal and outputs the result. - It indicates where the program will print the elements of `stack1`. **Note:** The header file `linkedStack.h` is not found, which would cause a build failure. This needs to be resolved to successfully compile and run the program.
Certainly! Here is the transcription of the code shown in the image: --- ```cpp #include #include int main() { linkedStackType stack1; linkedStackType stack2; int x; // Prompt user for stack elements cout << "Enter elements with indicating the end of the element (with -999)" << endl; // Add the elements to Stack 1 while(1){ cin >> x; if(x == -999) break; stack1.push(x); } stack2 = stack1; // Compare Stack 1 and Stack 2 if(stack1 == stack2){ cout << "Stack1 and Stack2 are identical" << endl; } else { cout << "Stack1 and Stack2 are not identical" << endl; } cout << endl; // Reverse the order of Stack 1 stack1.reverseStack(stack2); if(stack1 == stack2){ cout << "After reverse, the Stack1 and Stack2 are still identical" << endl; } else { cout << "After reverse, the Stack1 and Stack2 are not identical" << endl; } cout << "--------------------------------------------------------" << endl; cout << "The Stack 1 items: " << endl; cout << "--------------------------------------------------------" << endl; // Print the elements of Stack 1 } ``` **Explanation:** - The program includes a stack implementation using the library `linkedStack.h` and standard `iostream`. - Two stacks, `stack1` and `stack2`, are created using a linked stack type for integers. - User is prompted to input elements into `stack1` until they enter `-999`, which indicates the end of inputs. - `stack2` is assigned the same elements as `stack1`. - The program checks if the two stacks are identical and outputs the result. - The order of elements in `stack1` is then reversed. - It checks again if the stacks are identical after the reversal and outputs the result. - It indicates where the program will print the elements of `stack1`. **Note:** The header file `linkedStack.h` is not found, which would cause a build failure. This needs to be resolved to successfully compile and run the program.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
What I'm I getting wrong?

Transcribed Image Text:Certainly! Here is the transcription of the code shown in the image:
---
```cpp
#include <linkedStack.h>
#include <iostream>
int main() {
linkedStackType<int> stack1;
linkedStackType<int> stack2;
int x;
// Prompt user for stack elements
cout << "Enter elements with indicating the end of the element (with -999)" << endl;
// Add the elements to Stack 1
while(1){
cin >> x;
if(x == -999) break;
stack1.push(x);
}
stack2 = stack1;
// Compare Stack 1 and Stack 2
if(stack1 == stack2){
cout << "Stack1 and Stack2 are identical" << endl;
} else {
cout << "Stack1 and Stack2 are not identical" << endl;
}
cout << endl;
// Reverse the order of Stack 1
stack1.reverseStack(stack2);
if(stack1 == stack2){
cout << "After reverse, the Stack1 and Stack2 are still identical" << endl;
} else {
cout << "After reverse, the Stack1 and Stack2 are not identical" << endl;
}
cout << "--------------------------------------------------------" << endl;
cout << "The Stack 1 items: " << endl;
cout << "--------------------------------------------------------" << endl;
// Print the elements of Stack 1
}
```
**Explanation:**
- The program includes a stack implementation using the library `linkedStack.h` and standard `iostream`.
- Two stacks, `stack1` and `stack2`, are created using a linked stack type for integers.
- User is prompted to input elements into `stack1` until they enter `-999`, which indicates the end of inputs.
- `stack2` is assigned the same elements as `stack1`.
- The program checks if the two stacks are identical and outputs the result.
- The order of elements in `stack1` is then reversed.
- It checks again if the stacks are identical after the reversal and outputs the result.
- It indicates where the program will print the elements of `stack1`.
**Note:** The header file `linkedStack.h` is not found, which would cause a build failure. This needs to be resolved to successfully compile and run the program.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
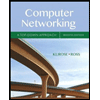
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
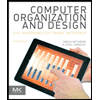
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
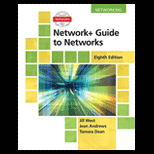
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
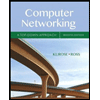
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
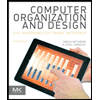
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
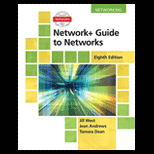
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
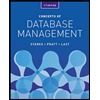
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
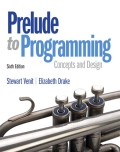
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
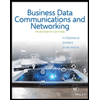
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY