Computer Science Complete the program 'Ring-1.c' to make the program work well for any number of processes specified by users and have one possible output sequence as follows if we run the program using 4 processes. Process 0: Token value < 4 > is received from process 3 Process 2: Token value < 2 > is received from process 1 Process 1: Token value < 1 > is received from process 0 Process 3: Token value < 3 > is received from process 2 #include #include #include int main(void) { int comm_sz, my_rank; MPI_Init(NULL, NULL); MPI_Comm_size(MPI_COMM_WORLD, &comm_sz); MPI_Comm_rank(MPI_COMM_WORLD, &my_rank); int token = 1; MPI_Send(&token, 1, MPI_INT, (my_rank + 1) % comm_sz, 0, MPI_COMM_WORLD); if (my_rank == 0) { MPI_Recv(&token, 1, MPI_INT, comm_sz - 1, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE); /* print out the message */ printf("Process %d received token value < %d > from process %d\n", my_rank, token, comm_sz - 1); } else { MPI_Recv(&token, 1, MPI_INT, my_rank - 1, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE); /* print out the message */ printf("Process %d received token value < %d > from process %d\n", my_rank, token, my_rank - 1); } MPI_Finalize(); return 0; }.
Computer Science
Complete the program 'Ring-1.c' to make the program work well for any number of processes specified by users and have one possible output sequence as follows if we run the program using 4 processes.
Process 0: Token value < 4 > is received from process 3
Process 2: Token value < 2 > is received from process 1
Process 1: Token value < 1 > is received from process 0
Process 3: Token value < 3 > is received from process 2
#include <stdio.h>
#include <stdlib.h>
#include <mpi.h>
int main(void)
{
int comm_sz, my_rank;
MPI_Init(NULL, NULL);
MPI_Comm_size(MPI_COMM_WORLD, &comm_sz);
MPI_Comm_rank(MPI_COMM_WORLD, &my_rank);
int token = 1;
MPI_Send(&token, 1, MPI_INT, (my_rank + 1) % comm_sz, 0, MPI_COMM_WORLD);
if (my_rank == 0)
{
MPI_Recv(&token, 1, MPI_INT, comm_sz - 1, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE);
/* print out the message */
printf("Process %d received token value < %d > from process %d\n", my_rank, token, comm_sz - 1);
}
else
{
MPI_Recv(&token, 1, MPI_INT, my_rank - 1, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE);
/* print out the message */
printf("Process %d received token value < %d > from process %d\n", my_rank, token, my_rank - 1);
}
MPI_Finalize();
return 0;
}.
What am i missing

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

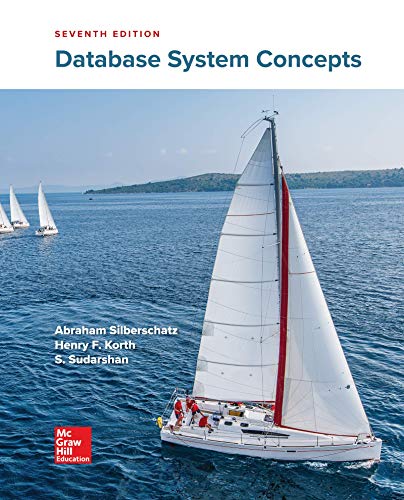
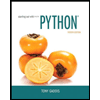
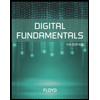
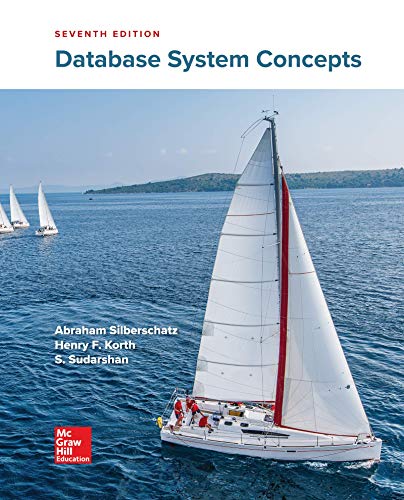
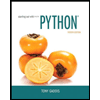
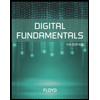
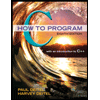
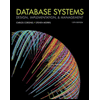
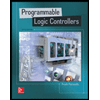