Complete the following Standardization class. Recall that we want to compute the mean and STD for each column! Think about what value we need to set the 'axis' argument equal to in order to achieve this. In the fit() method, compute the mean of the input X using np.mean(). Store the output into the variable self.mean. In the fit() method, compute the STD of the input X using np.std(). Store the output into the variable self.std. In the transform() method, compute and return the standardization for the input X. In other words, convert the standardization general formula into code and return the output. class Standardization(BaseEstimator, TransformerMixin): def __init__(self): pass def fit(self, X, y=None): # TODO 13.1 self.mean = np.mean(X) # TODO 13.2 self.std = np.std(X) # Always return self return self def transform(self, X): # TODO 13.3 return scale = Standardization() scaled_df = scale.fit_transform(X_train.iloc[:, 19:]) display(scaled_df) todo_check([ (scaled_df.shape == (413, 10), 'scaled_df does not have the right shape of (413, 10)'), (np.all(np.isclose(scaled_df.values[0, [1, -1]], np.array([-1.09485913, -0.06781709]),rtol=.01)), 'scaled_df does not contain the correct values!'), (np.all(np.isclose(scale.transform(X_test.iloc[:, 19:].mean()).values, np.array([ 0.01785169, -0.0915082 , 0.09302418, -0.09879271, -0.03740731,0.31735123, 0.00172016, -0.0798864 , 0.05723329, -0.00198524]),rtol=.01)), 'Incorrect results when computing the scaled values for X_test. Make sure your transform() method is correct!') ])
TODO 13
Complete the following Standardization class. Recall that we want to compute the mean and STD for each column! Think about what value we need to set the 'axis' argument equal to in order to achieve this.
-
In the fit() method, compute the mean of the input X using np.mean(). Store the output into the variable self.mean.
-
In the fit() method, compute the STD of the input X using np.std(). Store the output into the variable self.std.
-
In the transform() method, compute and return the standardization for the input X. In other words, convert the standardization general formula into code and return the output.
class Standardization(BaseEstimator, TransformerMixin):
def __init__(self):
pass
def fit(self, X, y=None):
# TODO 13.1
self.mean = np.mean(X)
# TODO 13.2
self.std = np.std(X)
# Always return self
return self
def transform(self, X):
# TODO 13.3
return
scale = Standardization()
scaled_df = scale.fit_transform(X_train.iloc[:, 19:])
display(scaled_df)
todo_check([
(scaled_df.shape == (413, 10), 'scaled_df does not have the right shape of (413, 10)'),
(np.all(np.isclose(scaled_df.values[0, [1, -1]], np.array([-1.09485913, -0.06781709]),rtol=.01)), 'scaled_df does not contain the correct values!'),
(np.all(np.isclose(scale.transform(X_test.iloc[:, 19:].mean()).values, np.array([ 0.01785169, -0.0915082 , 0.09302418, -0.09879271, -0.03740731,0.31735123, 0.00172016, -0.0798864 , 0.05723329, -0.00198524]),rtol=.01)), 'Incorrect results when computing the scaled values for X_test. Make sure your transform() method is correct!')
])

Here is the updated code for the Standardization class:
Code with comments:
class Standardization(BaseEstimator, TransformerMixin):
def __init__(self):
pass
def fit(self, X, y=None):
# Compute the mean of the input X
self.mean = np.mean(X, axis=0)
# Compute the STD of the input X
self.std = np.std(X, axis=0)
# Always return self
return self
def transform(self, X):
# Compute and return the standardization for the input X
return (X - self.mean) / self.std
Step by step
Solved in 2 steps with 1 images

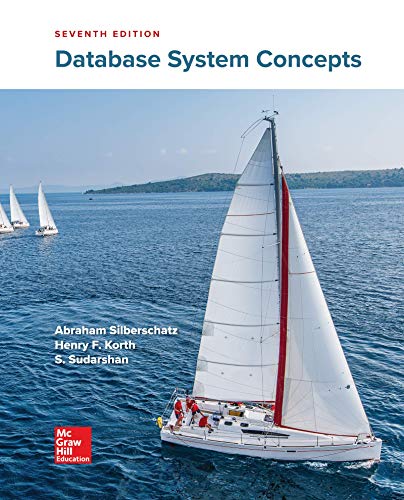
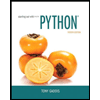
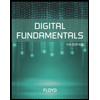
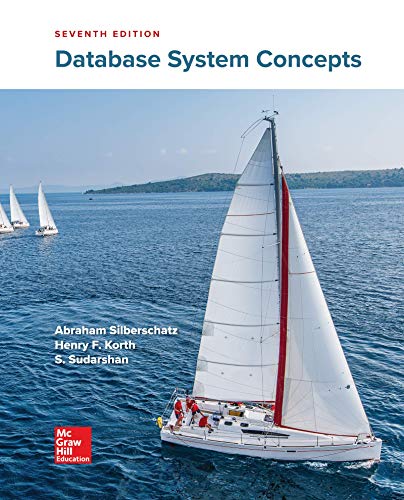
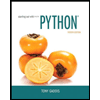
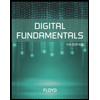
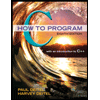
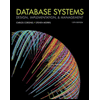
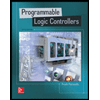