Complete the following C program below to display the addresses of the following entities: all functions global constant gc and string literal "Computer Science" all initialized global variables; all un-initialized global variables; all dynamically allocated memories; all formal parameters in any function; all local variables in any function; the array containing the addresses of all command line arguments and the array containing the addresses of all environment variables; start and end of its command line arguments; start and end of its environment (variables and values). Note: please do not change the program except the areas marked "TO DO" (they have been bolded). #include #include #include #include #include #include extern char **environ; int gx = 10; // initialised global int gy; // uninitialised global char gname1[] = "Hi, there!"; char *gname2 = "Computer Science"; const int gc = 100; int gz; void printAddress(char *description, void *addr) { unsigned long a = (unsigned long) addr; unsigned long b = a & 0x3ff; unsigned long kib = a >> 10; kib = kib & 0x3ff; unsigned long mib = a >> 20; mib = mib & 0x3ff; unsigned long gib = a >> 30; gib = gib & 0x3ff; unsigned long tib = a >> 40; tib = tib & 0x3ff; printf("%70s: %16p (%luTiB, %luGiB, %luMiB, %luKiB, %luB)\n", description, addr, tib, gib, mib, kib, b); return; } int f1(int x1, int x2, float x3, double x4, char x5, int x6 ) { int f1_l1; float f1_l2; char f1_l3; char f1_l3b; double f1_l4; int f1_l5; int f1_l6; // TO DO: // print the addresses of all formal parameters of function f1 // print the addresses of all local variables of function f1 return 0; } void f2() { #define BUFSIZE 1024*1024 char buf[BUFSIZE]; char *p; p = malloc(BUFSIZE); if (p==NULL) { perror("mallc memory"); exit(1); } // TO DO: // print the addresses of local variables buf and p of function f2 // print the addresses of heap allocated memory pointed to by p in function f2 printf("\n==== call function f1 in function f2 ====\n"); f1(10, 20, 10.2, 20.3, 'a', 100); return; } int main(int argc, char *argv[], char *env[]) { printf("==== program text ====\n"); printAddress("start address of function printAddress", printAddress); // TO DO: // print the addresses of function f1, f2 and main printf("\n==== constants and initialised globals ====\n"); // TO DO: // print the addresses of constant gc and string literal "Computer Science" // print the addresses of initialised global variables gx, gname1, gname2 printf("\n==== uninitialised globals ====\n"); // print the addresses of uninitialised global variables gy, gz printf("\n==== formal parameters in function main ====\n"); // TO DO: // print the addresses of formal parameters argv, argv and env printf("\n==== heap ====\n"); char *p1 = malloc(200); char *p2 = malloc(10000); printf("\n==== local variables in main ====\n"); // TO DO: // print the addresses of local variables p1, p2 printf("\n==== heap ====\n"); // TO DO: // print the addresses of heap allocated memmory pointed to by p1 and p2 printf("\n==== call function f2 from main function ====\n"); f2(); printf("\n==== arrays of pointers to cmd line arguments and env variables ====\n"); // TO DO: // print the addresses of arrays of pointers point to cmd line arguments and env variables printf("\n==== command line arguments ====\n"); // TO DO: // print start and end addresses of cmd line arguments printf("\n==== environment ====\n"); // TO DO: // print start and end addresses of environment variables exit(0
- Complete the following C program below to display the addresses of the following entities:
- all functions
- global constant gc and string literal "Computer Science"
- all initialized global variables;
- all un-initialized global variables;
- all dynamically allocated memories;
- all formal parameters in any function;
- all local variables in any function;
- the array containing the addresses of all command line arguments and the array containing the addresses of all environment variables;
- start and end of its command line arguments;
- start and end of its environment (variables and values).
Note: please do not change the program except the areas marked "TO DO" (they have been bolded).
#include <stdlib.h>
#include <stdio.h>
#include <math.h>
#include <string.h>
#include <unistd.h>
#include <sys/resource.h>
extern char **environ;
int gx = 10; // initialised global
int gy; // uninitialised global
char gname1[] = "Hi, there!";
char *gname2 = "Computer Science";
const int gc = 100;
int gz;
void printAddress(char *description, void *addr)
{
unsigned long a = (unsigned long) addr;
unsigned long b = a & 0x3ff;
unsigned long kib = a >> 10; kib = kib & 0x3ff;
unsigned long mib = a >> 20; mib = mib & 0x3ff;
unsigned long gib = a >> 30; gib = gib & 0x3ff;
unsigned long tib = a >> 40; tib = tib & 0x3ff;
printf("%70s: %16p (%luTiB, %luGiB, %luMiB, %luKiB, %luB)\n", description, addr, tib, gib, mib, kib, b);
return;
}
int f1(int x1, int x2, float x3, double x4, char x5, int x6 )
{
int f1_l1;
float f1_l2;
char f1_l3;
char f1_l3b;
double f1_l4;
int f1_l5;
int f1_l6;
// TO DO:
// print the addresses of all formal parameters of function f1
// print the addresses of all local variables of function f1
return 0;
}
void f2()
{
#define BUFSIZE 1024*1024
char buf[BUFSIZE];
char *p;
p = malloc(BUFSIZE);
if (p==NULL) {
perror("mallc memory");
exit(1);
}
// TO DO:
// print the addresses of local variables buf and p of function f2
// print the addresses of heap allocated memory pointed to by p in function f2
printf("\n==== call function f1 in function f2 ====\n");
f1(10, 20, 10.2, 20.3, 'a', 100);
return;
}
int main(int argc, char *argv[], char *env[])
{
printf("==== program text ====\n");
printAddress("start address of function printAddress", printAddress);
// TO DO:
// print the addresses of function f1, f2 and main
printf("\n==== constants and initialised globals ====\n");
// TO DO:
// print the addresses of constant gc and string literal "Computer Science"
// print the addresses of initialised global variables gx, gname1, gname2
printf("\n==== uninitialised globals ====\n");
// print the addresses of uninitialised global variables gy, gz
printf("\n==== formal parameters in function main ====\n");
// TO DO:
// print the addresses of formal parameters argv, argv and env
printf("\n==== heap ====\n");
char *p1 = malloc(200);
char *p2 = malloc(10000);
printf("\n==== local variables in main ====\n");
// TO DO:
// print the addresses of local variables p1, p2
printf("\n==== heap ====\n");
// TO DO:
// print the addresses of heap allocated memmory pointed to by p1 and p2
printf("\n==== call function f2 from main function ====\n");
f2();
printf("\n==== arrays of pointers to cmd line arguments and env variables ====\n");
// TO DO:
// print the addresses of arrays of pointers point to cmd line arguments and env variables
printf("\n==== command line arguments ====\n");
// TO DO:
// print start and end addresses of cmd line arguments
printf("\n==== environment ====\n");
// TO DO:
// print start and end addresses of environment variables
exit(0);
}

The program starts in the main function.
Addresses of various functions, constants, globals, formal parameters, local variables, and dynamic allocations are printed.
The function f2 is called from the main function, which further calls f1. Inside these functions, addresses of their formal parameters, local variables, and dynamic allocations are printed.
Step by step
Solved in 3 steps with 1 images

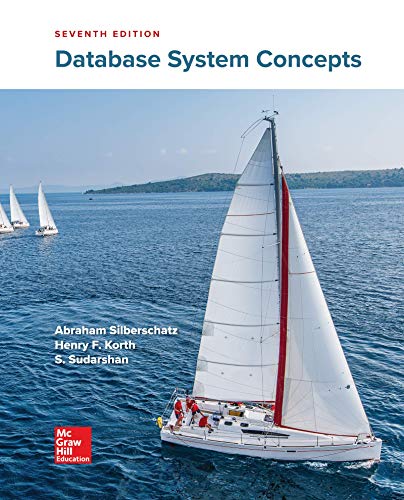
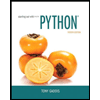
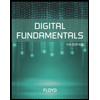
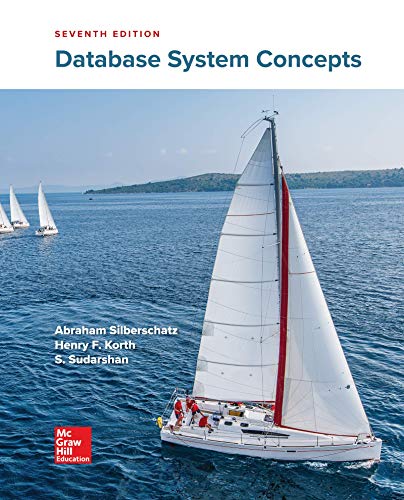
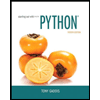
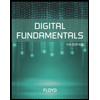
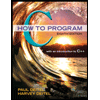
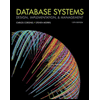
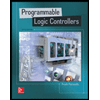