Complete Programming Exercise #6 from Chapter 18. Upload all source code files and screenshot of your output. Write a program to test the selection sort algorithm for array-based lists as given in this chapter. USE THIS FILE template int seqSearch(const elemType list[], int length, const elemType& item) { int loc; bool found = false; loc = 0; while (loc < length && !found) if (list[loc] == item) found = true; else loc++; if (found) return loc; else return -1; } //end seqSearch template int binarySearch(const elemType list[], int length, const elemType& item) { int first = 0; int last = length - 1; int mid; bool found = false; while (first <= last && !found) { mid = (first + last) / 2; if (list[mid] == item) found = true; else if (list[mid] > item) last = mid - 1; else first = mid + 1; } if (found) return mid; else return -1; } //end binarySearch template void bubbleSort(elemType list[], int length) { for (int iteration = 1; iteration < length; iteration++) { for (int index = 0; index < length - iteration; index++) { if (list[index] > list[index + 1]) { elemType temp = list[index]; list[index] = list[index + 1]; list[index + 1] = temp; } } } } //end bubbleSort template void selectionSort(elemType list[], int length) { int minIndex; for (int loc = 0; loc < length; loc++) { minIndex = minLocation(list, loc, length - 1); swap(list, loc, minIndex); } } //end selectionSort template void swap(elemType list[], int first, int second) { elemType temp; temp = list[first]; list[first] = list[second]; list[second] = temp; } //end swap template int minLocation(elemType list[], int first, int last) { int minIndex; minIndex = first; for (int loc = first + 1; loc <= last; loc++) if (list[loc] < list[minIndex]) minIndex = loc; return minIndex; } //end minLocation template void insertionSort(elemType list[], int length) { for (int firstOutOfOrder = 1; firstOutOfOrder < length; firstOutOfOrder++) if (list[firstOutOfOrder] < list[firstOutOfOrder - 1]) { elemType temp = list[firstOutOfOrder]; int location = firstOutOfOrder; do { list[location] = list[location - 1]; location--; } while(location > 0 && list[location - 1] > temp); list[location] = temp; } } //end insertionSort template void quickSort(elemType list[], int length) { recQuickSort(list, 0, length - 1); } //end quickSort template void recQuickSort(elemType list[], int first, int last) { int pivotLocation; if (first < last) { pivotLocation = partition(list, first, last); recQuickSort(list, first, pivotLocation - 1); recQuickSort(list, pivotLocation + 1, last); } } //end recQuickSort template int partition(elemType list[], int first, int last) { elemType pivot; int smallIndex; swap(list, first, (first + last) / 2); pivot = list[first]; smallIndex = first; for (int index = first + 1; index <= last; index++) if (list[index] < pivot) { smallIndex++; swap(list, smallIndex, index); } swap(list, first, smallIndex); return smallIndex; } //end partition template void heapSort(elemType list[], int length) { buildHeap(list, length); for (int lastOutOfOrder = length - 1; lastOutOfOrder >= 0; lastOutOfOrder--) { elemType temp = list[lastOutOfOrder];list[lastOutOfOrder] = list[0]; list[0] = temp; heapify(list, 0, lastOutOfOrder - 1); }//end for }//end heapSort template void heapify(elemType list[], int low, int high) { int largeIndex; elemType temp = list[low]; //copy the root node of //the subtree largeIndex = 2 * low + 1; //index of the left child while (largeIndex <= high) { if (largeIndex < high) if (list[largeIndex] < list[largeIndex + 1]) largeIndex = largeIndex + 1; //index of the //largest child if (temp > list[largeIndex]) //subtree //is already in a heap break; else { list[low] = list[largeIndex]; //move the larger //child to the root low = largeIndex; //go to the subtree to //restore the heap largeIndex = 2 * low + 1; } }//end while list[low] = temp; //insert temp into the tree, //that is, list }//end heapify template void buildHeap(elemType list[], int length) { for (int index = length / 2 - 1; index >= 0; index--) heapify(list, index, length - 1); }
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
D.S. Malik - C++ PROGRAMMING 8th edition CHAPTER 18 Page 1357 Exercise #6
Complete Programming Exercise #6 from Chapter 18. Upload all source code files and screenshot of your output.
Write a program to test the selection sort
USE THIS FILE
template <class elemType>
int seqSearch(const elemType list[], int length, const elemType& item)
{
int loc;
bool found = false;
loc = 0;
while (loc < length && !found)
if (list[loc] == item)
found = true;
else
loc++;
if (found)
return loc;
else
return -1;
} //end seqSearch
template <class elemType>
int binarySearch(const elemType list[], int length,
const elemType& item)
{
int first = 0;
int last = length - 1;
int mid;
bool found = false;
while (first <= last && !found)
{
mid = (first + last) / 2;
if (list[mid] == item)
found = true;
else if (list[mid] > item)
last = mid - 1;
else
first = mid + 1;
}
if (found)
return mid;
else
return -1;
} //end binarySearch
template <class elemType>
void bubbleSort(elemType list[], int length)
{
for (int iteration = 1; iteration < length; iteration++)
{
for (int index = 0; index < length - iteration;
index++)
{
if (list[index] > list[index + 1]) {
elemType temp = list[index];
list[index] = list[index + 1];
list[index + 1] = temp;
}
}
}
} //end bubbleSort
template <class elemType>
void selectionSort(elemType list[], int length)
{
int minIndex;
for (int loc = 0; loc < length; loc++)
{
minIndex = minLocation(list, loc, length - 1);
swap(list, loc, minIndex);
}
} //end selectionSort
template <class elemType>
void swap(elemType list[], int first, int second)
{
elemType temp;
temp = list[first];
list[first] = list[second];
list[second] = temp;
} //end swap
template <class elemType>
int minLocation(elemType list[], int first, int last)
{
int minIndex;
minIndex = first;
for (int loc = first + 1; loc <= last; loc++)
if (list[loc] < list[minIndex])
minIndex = loc;
return minIndex;
} //end minLocation
template <class elemType>
void insertionSort(elemType list[], int length)
{
for (int firstOutOfOrder = 1; firstOutOfOrder < length;
firstOutOfOrder++)
if (list[firstOutOfOrder] < list[firstOutOfOrder - 1])
{
elemType temp = list[firstOutOfOrder];
int location = firstOutOfOrder;
do
{
list[location] = list[location - 1];
location--; }
while(location > 0 && list[location - 1] > temp);
list[location] = temp;
}
} //end insertionSort
template <class elemType>
void quickSort(elemType list[], int length)
{
recQuickSort(list, 0, length - 1);
} //end quickSort
template <class elemType>
void recQuickSort(elemType list[], int first, int last)
{
int pivotLocation;
if (first < last)
{
pivotLocation = partition(list, first, last);
recQuickSort(list, first, pivotLocation - 1);
recQuickSort(list, pivotLocation + 1, last);
}
} //end recQuickSort
template <class elemType>
int partition(elemType list[], int first, int last)
{
elemType pivot;
int smallIndex;
swap(list, first, (first + last) / 2);
pivot = list[first];
smallIndex = first;
for (int index = first + 1; index <= last; index++)
if (list[index] < pivot)
{
smallIndex++;
swap(list, smallIndex, index);
}
swap(list, first, smallIndex);
return smallIndex;
} //end partition
template <class elemType>
void heapSort(elemType list[], int length)
{
buildHeap(list, length);
for (int lastOutOfOrder = length - 1; lastOutOfOrder >= 0;
lastOutOfOrder--)
{
elemType temp = list[lastOutOfOrder];list[lastOutOfOrder] = list[0];
list[0] = temp;
heapify(list, 0, lastOutOfOrder - 1);
}//end for
}//end heapSort
template <class elemType>
void heapify(elemType list[], int low, int high)
{
int largeIndex;
elemType temp = list[low]; //copy the root node of
//the subtree
largeIndex = 2 * low + 1; //index of the left child
while (largeIndex <= high)
{
if (largeIndex < high)
if (list[largeIndex] < list[largeIndex + 1])
largeIndex = largeIndex + 1; //index of the
//largest child
if (temp > list[largeIndex]) //subtree
//is already in a heap
break;
else
{
list[low] = list[largeIndex]; //move the larger
//child to the root
low = largeIndex; //go to the subtree to
//restore the heap
largeIndex = 2 * low + 1;
}
}//end while
list[low] = temp; //insert temp into the tree,
//that is, list
}//end heapify
template <class elemType>
void buildHeap(elemType list[], int length)
{
for (int index = length / 2 - 1; index >= 0; index--)
heapify(list, index, length - 1);
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

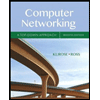
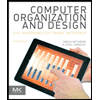
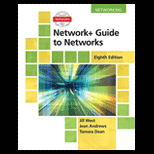
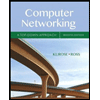
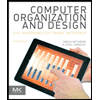
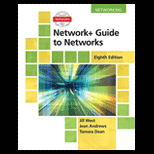
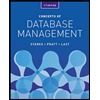
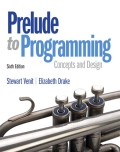
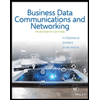