"com.ibm.db2.jdbc.app.DB2Driver"; String URL = "jdbc:db2://*local"; // Register the native JDBC driver. If the driver cannot // be registered, the test cannot continue. try { Class.forName(DRIVER); } catch (Exception e) { System.out.println("Driver failed to register."); System.out.println(e.getMessage()); System.exit(1); } Connection c = null; Statement s = null; // This program creates a table that is // used by prepared statements later. try { // Create the connection properties. Properties properties = new Properties (); properties.put ("user", "userid"); properties.put ("password", "password"); // Connect to the local database. c = DriverManager.getConnection(URL, properties); // Create a Statement object. s = c.createStatement(); // Delete the test table if it exists. Note that // this example assumes throughout that the collection // MYLIBRARY exists on the system. tr
import java.sql.*; import java.util.Properties; public class PreparedStatementExample { public static void main(java.lang.String[] args) { // Load the following from a properties object. String DRIVER = "com.ibm.db2.jdbc.app.DB2Driver"; String URL = "jdbc:db2://*local"; // Register the native JDBC driver. If the driver cannot // be registered, the test cannot continue. try { Class.forName(DRIVER); } catch (Exception e) { System.out.println("Driver failed to register."); System.out.println(e.getMessage()); System.exit(1); } Connection c = null; Statement s = null; // This program creates a table that is // used by prepared statements later. try { // Create the connection properties. Properties properties = new Properties (); properties.put ("user", "userid"); properties.put ("password", "password"); // Connect to the local
Incorrect downvote alsi.

Step by step
Solved in 3 steps with 4 images

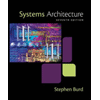
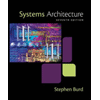