Code needed for the following: Use the final numerical grade to look up the corresponding letter grade as follows: 90 - 100 = A 80 - 89 = B 70 - 79 = C 60 - 69 = D < 60 = F Use the final letter grade to look up a "witty" comment of your choice, using a SWITCH statement. A statement must be output for each letter grade above. For example: A = "Most excellent work” B = "IMHO You Passed" etc. Request an output file name from the user. Do not “hard-code” the file name in the program. Do not “hard-code” the file name in the program. Check to ensure the output file opens. If it does not open, display an appropriate message to the user and exit the program. For each student, output the following to the console (screen) and the output file in a neat, readable format. Output each input line on one output line. Use manipulators to output values in readable columns: Student Name, Total Points (numerical grade): NN. Letter Grade: X. Depending on letter grade, The witty comment. Code written thus far: _______________________________________________________#include <iostream>#include <fstream>#include <iomanip> using namespace std; int main() {int totalGrades; // This is the total number of test grades int grade; // This is the student's test grade ifstream inFile; // This is a declaration of the inFile that holds all the grades string fileName; // This is the filename that the user will enter string name; // This is the name of the student string surname; int gradeCount; // This is to keep count of the number of grades entered int hwGradesN; // Number of homework grades int hwGradesV; // Homework grade values float hwGradesP; // Homework grade percentage int progGradesN; // Number of program grades int progGradesV; // Program grade values float progGradesP; // Program grade percentage int examGradesN; // Number of exam grades int examGradesV; // Exam grade values float examGradesP; // Exam grade percentage int totalHWpoints; // Total homework points earned int totalProgpoints; // Total program points earned int totalExampoints; // Total exam points earned int maxHWpoints; // The maximum points available for homework grades int maxProgpoints; // The maximum points available for program grades int maxExampoints; // The maximum points available for exam grades float finalGrade; // Final grade float average; // The average of all the grades cout << "Enter the input file name to read student grades: "; cin >> fileName; // Open the file with the grades inFile.open(fileName.c_str ()); // Check to make sure the file opened correctly if (!inFile) {cout << "File did not open correctly." << endl;return 1;}// Reads scores from file} while(!inFile.eof()){ totalHWpoints=0; totalProgpoints=0; totalExampoints=0; inFile>>name; inFile>>surname; inFile>>hwGradesN; maxHWpoints = hwGradesN*100; while(hwGradesN--){ inFile>>hwGradesV; totalHWpoints+=hwGradesV; } inFile>>hwGradesP; maxProgpoints = hwGradesP*100; inFile>>progGradesN; while(progGradesN--){ inFile>>progGradesV; totalProgpoints+=progGradesV; } inFile>>progGradesP; inFile>>examGradesN; maxExampoints = examGradesN*100; while(examGradesN--){ inFile>>examGradesV; totalExampoints+=examGradesV; } inFile>>examGradesP; finalGrade= (((totalHWpoints/maxHWpoints) * hwGradesP) + ((totalProgpoints/maxProgpoints) * progGradesP) + ((totalExampoints/maxExampoints) * examGradesP)) * 100; cout << "Final Grade of "<<name<<" "<<surname<<": " << finalGrade << endl; } // Calculate the average. //average = (score1 + score2 + score3) / 300; // cout << << average << endl; // Display the average. // if (average>=90) finalGrade = 'A'; // if (average<90 && average >=80 ) finalGrade = 'B'; // if (average<80 && average >=70) finalGrade = 'C'; // if (average<70 && average >=60) finalGrade = 'D'; // if (average<=60) grade = 'F'; finalGrade = 'F'; // // cout << "Final Grade: " << finalGrade << endl; return 0;}}
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Code needed for the following:
Use the final numerical grade to look up the corresponding letter grade as follows:
90 - 100 = A
80 - 89 = B
70 - 79 = C
60 - 69 = D
< 60 = F
Use the final letter grade to look up a "witty" comment of your choice, using a SWITCH statement. A statement must be output for each letter grade above. For example:
A = "Most excellent work”
B = "IMHO You Passed"
etc.
Request an output file name from the user. Do not “hard-code” the file name in the
For each student, output the following to the console (screen) and the output file in a neat, readable format. Output each input line on one output line. Use manipulators to output values in readable columns:
Student Name, Total Points (numerical grade): NN. Letter Grade: X. Depending on letter grade, The witty comment.
Code written thus far:
_______________________________________________________
#include <iostream>
#include <fstream>
#include <iomanip>
using namespace std;
int main()
{
int totalGrades; // This is the total number of test grades
int grade; // This is the student's test grade
ifstream inFile; // This is a declaration of the inFile that holds all the grades
string fileName; // This is the filename that the user will enter
string name; // This is the name of the student
string surname;
int gradeCount; // This is to keep count of the number of grades entered
int hwGradesN; // Number of homework grades
int hwGradesV; // Homework grade values
float hwGradesP; // Homework grade percentage
int progGradesN; // Number of program grades
int progGradesV; // Program grade values
float progGradesP; // Program grade percentage
int examGradesN; // Number of exam grades
int examGradesV; // Exam grade values
float examGradesP; // Exam grade percentage
int totalHWpoints; // Total homework points earned
int totalProgpoints; // Total program points earned
int totalExampoints; // Total exam points earned
int maxHWpoints; // The maximum points available for homework grades
int maxProgpoints; // The maximum points available for program grades
int maxExampoints; // The maximum points available for exam grades
float finalGrade; // Final grade
float average; // The average of all the grades
cout << "Enter the input file name to read student grades: ";
cin >> fileName;
// Open the file with the grades
inFile.open(fileName.c_str ());
// Check to make sure the file opened correctly
if (!inFile)
{
cout << "File did not open correctly." << endl;
return 1;
}
// Reads scores from file
}
while(!inFile.eof()){
totalHWpoints=0;
totalProgpoints=0;
totalExampoints=0;
inFile>>name;
inFile>>surname;
inFile>>hwGradesN;
maxHWpoints = hwGradesN*100;
while(hwGradesN--){
inFile>>hwGradesV;
totalHWpoints+=hwGradesV;
}
inFile>>hwGradesP;
maxProgpoints = hwGradesP*100;
inFile>>progGradesN;
while(progGradesN--){
inFile>>progGradesV;
totalProgpoints+=progGradesV;
}
inFile>>progGradesP;
inFile>>examGradesN;
maxExampoints = examGradesN*100;
while(examGradesN--){
inFile>>examGradesV;
totalExampoints+=examGradesV;
}
inFile>>examGradesP;
finalGrade= (((totalHWpoints/maxHWpoints) * hwGradesP) + ((totalProgpoints/maxProgpoints) * progGradesP) + ((totalExampoints/maxExampoints) * examGradesP)) * 100;
cout << "Final Grade of "<<name<<" "<<surname<<": " << finalGrade << endl;
}
// Calculate the average.
//average = (score1 + score2 + score3) / 300;
// cout << << average << endl; // Display the average.
// if (average>=90) finalGrade = 'A';
// if (average<90 && average >=80 ) finalGrade = 'B';
// if (average<80 && average >=70) finalGrade = 'C';
// if (average<70 && average >=60) finalGrade = 'D';
// if (average<=60) grade = 'F'; finalGrade = 'F';
//
// cout << "Final Grade: " << finalGrade << endl;
return 0;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

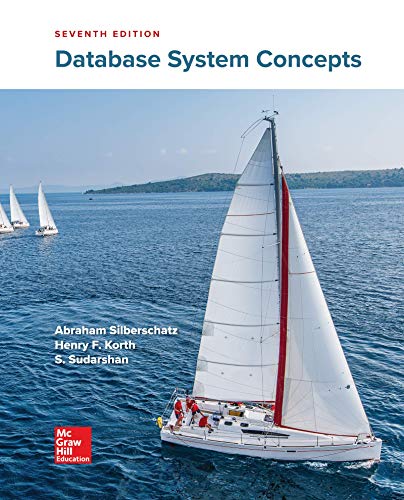
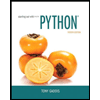
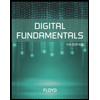
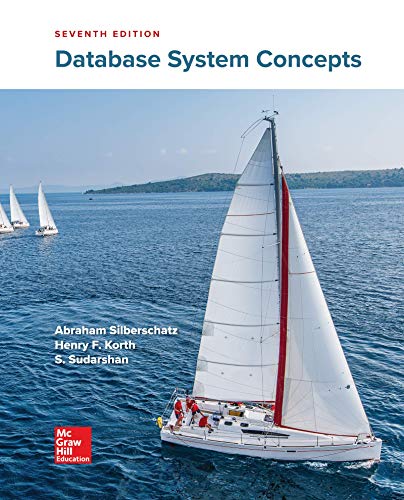
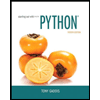
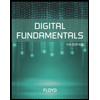
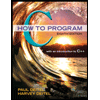
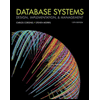
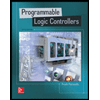