class Employee { private int empNum; private string firstName; private string lastName; private double monthlySalary; public Employee() { empNum = 0; firstName = ""; lastName = ""; monthlySalary = 0.0; } public Employee(int empNum, string firstName, string lastName, double monthlySalary) { this.empNum = empNum; this.firstName = firstName; this.lastName = lastName; this.monthlySalary = monthlySalary; } public double getAnnualPay() { return 12 * monthlySalary; } public void display() { Console.WriteLine("Employee Number: {0}, Name: {1} {2}, Monthly Salary: {3}", empNum, firstName, lastName, monthlySalary.ToString("C")); } public int EmpNum { get => empNum; set => empNum = value; } public string FirstName { get => firstName; set => firstName = value; } public string LastName { get => lastName; set => lastName = value; } public double MonthlySalary { get => monthlySalary; set => monthlySalary = value; } } class TestEmployee { static void Main(string[] args) { Employee emp1 = new Employee(); Employee emp2 = new Employee(2, "Charles", "Darwin", 2905.50); emp1.EmpNum = 1; emp1.FirstName = "Galileo"; emp1.LastName = "Galilei"; emp1.MonthlySalary = 3400.50; emp1.display(); Console.WriteLine("Annual Salary: {0}\n", emp1.getAnnualPay().ToString("C")); emp2.display(); Console.WriteLine("Annual Salary: {0}\n", emp2.getAnnualPay().ToString("C")); Console.ReadLine(); } } }
Can someone create a UML design diagram for the code I provided here? Please and thank you!
using System;
namespace Employee_Class
{
class Employee
{
private int empNum;
private string firstName;
private string lastName;
private double monthlySalary;
public Employee()
{
empNum = 0;
firstName = "";
lastName = "";
monthlySalary = 0.0;
}
public Employee(int empNum, string firstName, string lastName, double monthlySalary)
{
this.empNum = empNum;
this.firstName = firstName;
this.lastName = lastName;
this.monthlySalary = monthlySalary;
}
public double getAnnualPay()
{
return 12 * monthlySalary;
}
public void display()
{
Console.WriteLine("Employee Number: {0}, Name: {1} {2}, Monthly Salary: {3}", empNum, firstName, lastName, monthlySalary.ToString("C"));
}
public int EmpNum
{
get => empNum;
set => empNum = value;
}
public string FirstName
{
get => firstName;
set => firstName = value;
}
public string LastName
{
get => lastName;
set => lastName = value;
}
public double MonthlySalary
{
get => monthlySalary;
set => monthlySalary = value;
}
}
class TestEmployee
{
static void Main(string[] args)
{
Employee emp1 = new Employee();
Employee emp2 = new Employee(2, "Charles", "Darwin", 2905.50);
emp1.EmpNum = 1;
emp1.FirstName = "Galileo";
emp1.LastName = "Galilei";
emp1.MonthlySalary = 3400.50;
emp1.display();
Console.WriteLine("Annual Salary: {0}\n", emp1.getAnnualPay().ToString("C"));
emp2.display();
Console.WriteLine("Annual Salary: {0}\n", emp2.getAnnualPay().ToString("C"));
Console.ReadLine();
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

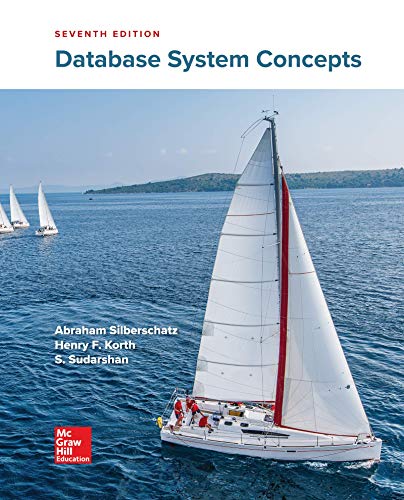
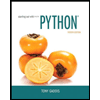
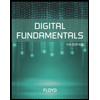
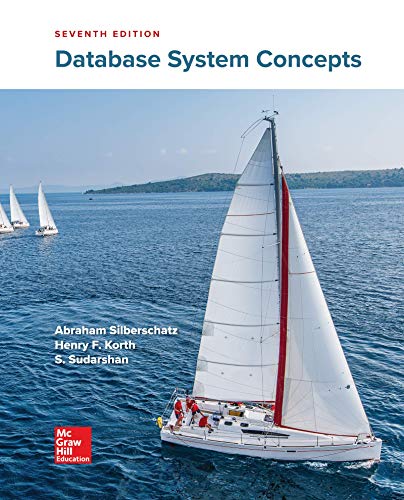
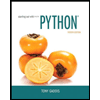
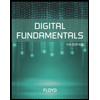
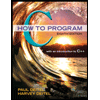
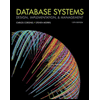
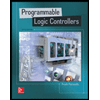