Character userCharacter is read from input. Write a while loop that reads characters from input until character 's' is read. Then, count the total number of characters read. Character 's' should not be included in the count. Ex: If the input is h s, then the output is: 1 1 import java.util.Scanner; 2 3 public class CountCalculator { 4 5 6 7 8 9 10 11 12 public static void main(String[] args) { Scanner scnr = new Scanner (System.in); char userCharacter; int result; result = 0; userCharacter = scnr. next().charAt(0); *Your code goes here */
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
I need help with this problem in Java
![## Challenge Activity: 6.2.2 - While Loops
**Objective:**
Create a program that reads characters from input until the character 's' is encountered. The program will then count and output the total number of characters read, excluding 's'.
### Example:
- **Input:** `h s`
- **Output:** `1`
### Instructions:
Implement the logic to achieve the above objective by completing the Java program below.
```java
import java.util.Scanner;
public class CountCalculator {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
char userCharacter;
int result;
result = 0;
userCharacter = scnr.next().charAt(0);
// Your code goes here
System.out.println(result);
}
}
```
### Steps:
1. **Import the Scanner:** This is used to read input from the user.
2. **Declare Variables:**
- `userCharacter` to store the character input.
- `result` to store the count of characters.
3. **Initialize Scanner:** Use `scnr` to capture input from `System.in`.
4. **Initiate the While Loop:**
- Read characters and count until 's' is encountered.
- Exclude 's' from the count.
5. **Output the Result:** Print the number of characters counted before 's'.
### Key Points:
- Ensure that any character read as 's' should not be included in the count.
- Use a while loop to iterate through the input character-by-character.
- Update and print the result once the loop condition is fulfilled.
Consider testing with different inputs to verify the program's accuracy and handling of edge cases.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4e135d5c-f867-4afd-9f0d-b505f4f19664%2F2a9fcc30-fbe4-4997-9436-8343fa654695%2Ffvy7f1_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

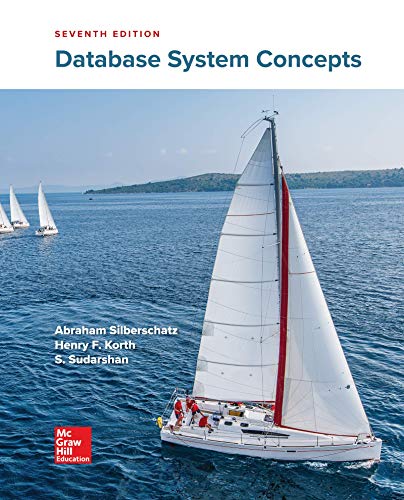
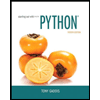
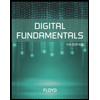
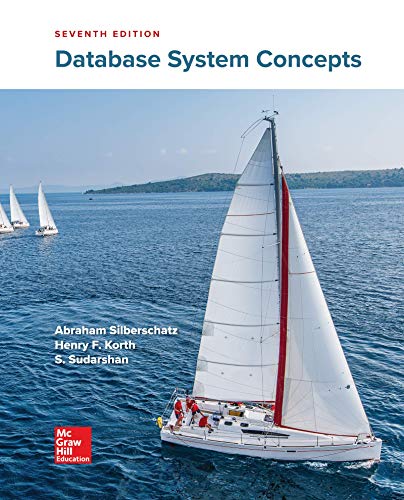
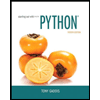
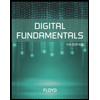
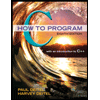
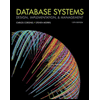
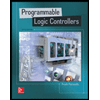