Change the following code so that when player wins the game, the game continues by creating new GameGUI with the same player. However the player's starting position is same, everything else should be reseted. public static void main(String[] args) { Labyrinth labyrinth = new Labyrinth(10); Player player = new Player(9, 0); Random rand = new Random(); Dragon dragon = new Dragon(rand.nextInt(10), 9); JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon); frame.setLayout(new BorderLayout()); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(gui, BorderLayout.CENTER); frame.pack(); frame.setResizable(false); frame.setVisible(true); } public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private Timer timer; private long elapsedTime; private final ImageIcon playerIcon = new ImageIcon("data/images/player.png"); private final ImageIcon dragonIcon = new ImageIcon("data/images/dragon.png"); private final ImageIcon wallIcon = new ImageIcon("data/images/wall.png"); private final ImageIcon emptyIcon = new ImageIcon("data/images/empty.png"); public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) { this.labyrinth = labyrinth; this.player = player; this.dragon = dragon; String playerName = JOptionPane.showInputDialog("Enter your name:"); player.setName(playerName); setFocusable(true); addKeyListener(new KeyAdapter() { @Override public void keyPressed(KeyEvent e) { char move = switch (e.getKeyCode()) { case KeyEvent.VK_W -> 'W'; case KeyEvent.VK_S -> 'S'; case KeyEvent.VK_A -> 'A'; case KeyEvent.VK_D -> 'D'; default -> ' '; }; if (move != ' ') { player.move(move, labyrinth); dragon.move(labyrinth); repaint(); checkGameState(); } } }); } private void checkGameState() { if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) { player.setScore(player.getScore() + 1); JOptionPane.showMessageDialog(this, "You escaped! Congratulations!"); System.exit(0); } if (Math.abs(player.getX() - dragon.getX()) <= 1 && Math.abs(player.getY() - dragon.getY()) <= 1) { String name = player.getName(); int score = player.getScore(); Object[] options = {"Quit", "Restart"}; int option = JOptionPane.showOptionDialog(this, "The dragon caught you! Game Over.", "Game Over", JOptionPane.YES_NO_OPTION, JOptionPane.QUESTION_MESSAGE, null, options, options[0]); if (option == JOptionPane.YES_OPTION) { System.exit(0); } else if (option == JOptionPane.NO_OPTION) { //restartGame(); } } }
Change the following code so that when player wins the game, the game continues by creating new GameGUI with the same player. However the player's starting position is same, everything else should be reseted.
public static void main(String[] args) {
Labyrinth labyrinth = new Labyrinth(10);
Player player = new Player(9, 0);
Random rand = new Random();
Dragon dragon = new Dragon(rand.nextInt(10), 9);
JFrame frame = new JFrame("Labyrinth Game");
GameGUI gui = new GameGUI(labyrinth, player, dragon);
frame.setLayout(new BorderLayout());
frame.setSize(600, 600);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(gui, BorderLayout.CENTER);
frame.pack();
frame.setResizable(false);
frame.setVisible(true); }
public class GameGUI extends JPanel {
private final Labyrinth labyrinth;
private final Player player;
private final Dragon dragon;
private Timer timer;
private long elapsedTime;
private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");
private final ImageIcon dragonIcon = new ImageIcon("data/images/dragon.png");
private final ImageIcon wallIcon = new ImageIcon("data/images/wall.png");
private final ImageIcon emptyIcon = new ImageIcon("data/images/empty.png");
public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) {
this.labyrinth = labyrinth;
this.player = player;
this.dragon = dragon;
String playerName = JOptionPane.showInputDialog("Enter your name:");
player.setName(playerName);
setFocusable(true);
addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
char move = switch (e.getKeyCode()) {
case KeyEvent.VK_W -> 'W';
case KeyEvent.VK_S -> 'S';
case KeyEvent.VK_A -> 'A';
case KeyEvent.VK_D -> 'D';
default -> ' ';
};
if (move != ' ') {
player.move(move, labyrinth);
dragon.move(labyrinth);
repaint();
checkGameState();
}
}
});
}
private void checkGameState() {
if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) {
player.setScore(player.getScore() + 1);
JOptionPane.showMessageDialog(this, "You escaped! Congratulations!");
System.exit(0);
}
if (Math.abs(player.getX() - dragon.getX()) <= 1 &&
Math.abs(player.getY() - dragon.getY()) <= 1) {
String name = player.getName();
int score = player.getScore();
Object[] options = {"Quit", "Restart"};
int option = JOptionPane.showOptionDialog(this, "The dragon caught you! Game Over.", "Game Over", JOptionPane.YES_NO_OPTION, JOptionPane.QUESTION_MESSAGE, null, options, options[0]);
if (option == JOptionPane.YES_OPTION) {
System.exit(0);
} else if (option == JOptionPane.NO_OPTION) {
//restartGame();
}
}
}

Step by step
Solved in 2 steps

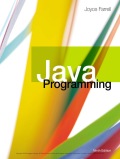
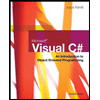
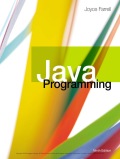
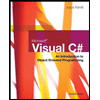
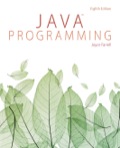
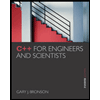