CHALLENGE 3.12.1: Using bool. ACTIVITY Assign is Teenager with true if kidAge is 13 to 19 inclusive. Otherwise, assign is Teenager with false. Learn how our autograder works 48067612799903/7 1 #include 2 #include 3 4 int main(void) ( 5 bool isTeenager; 6 int kidage; 7 8 9 10 11 12 13 14 15 16 17 scanf("%d", &kidage); * Your solution goes here / if (isTeenager) ( printf("Teen\n"); } else ( } printf("Not teen\n");
CHALLENGE 3.12.1: Using bool. ACTIVITY Assign is Teenager with true if kidAge is 13 to 19 inclusive. Otherwise, assign is Teenager with false. Learn how our autograder works 48067612799903/7 1 #include 2 #include 3 4 int main(void) ( 5 bool isTeenager; 6 int kidage; 7 8 9 10 11 12 13 14 15 16 17 scanf("%d", &kidage); * Your solution goes here / if (isTeenager) ( printf("Teen\n"); } else ( } printf("Not teen\n");
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Need help, C
 to understand the grading system.
---
For any queries or further assistance, refer to](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3e035166-c990-4dd0-a7c5-b2c139b07c7d%2F104baf34-fab4-49cc-a6e3-73022822734a%2Fheiqcyf_processed.png&w=3840&q=75)
Transcribed Image Text:### Section 3.12 - Introduction to Programming with C: Boolean Data Type
#### Challenge Activity 3.12.1: Using `bool`
**Objective:** Assign `isTeenager` with `true` if `kidAge` is between 13 and 19 inclusive. Otherwise, assign `isTeenager` with `false`.
**Instructions:**
1. Write a program in C that includes necessary header files and uses the `bool` data type.
2. Read an integer value representing a kid's age.
3. Determine if the age falls within the teenage range (13-19 years).
4. Print "Teen" if the age is within the range, otherwise print "Not teen".
**Code:**
```c
#include <stdio.h>
#include <stdbool.h>
int main(void) {
bool isTeenager;
int kidAge;
scanf("%d", &kidAge);
/* Your solution goes here */
if (isTeenager) {
printf("Teen\n");
}
else {
printf("Not teen\n");
}
return 0;
}
```
**Explanation:**
- The program starts by including the standard input-output library `<stdio.h>` and the boolean library `<stdbool.h>`.
- It declares a boolean variable `isTeenager` and an integer variable `kidAge`.
- The age (`kidAge`) is read from user input using `scanf`.
- You need to write the logic to assign `true` or `false` to `isTeenager` based on whether `kidAge` is between 13 and 19 inclusive.
- Depending on the value of `isTeenager`, the program then prints either "Teen" or "Not teen".
Click the 'Run' button to test your solution after writing the code inside the specified section.
#### Challenge Activity 3.12.2: Bool in Branching Statements
**Objective:** Write an if-else statement to describe an integer.
**Instructions:**
1. Determine if the integer is both even and positive.
2. Print "Positive even number" if both conditions are true.
3. If only one condition is true or both are false, handle accordingly:
- Print "Positive number" if only positive.
- Print "Not a positive number" otherwise.
Click [Learn how our autograder works](URL) to understand the grading system.
---
For any queries or further assistance, refer to
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
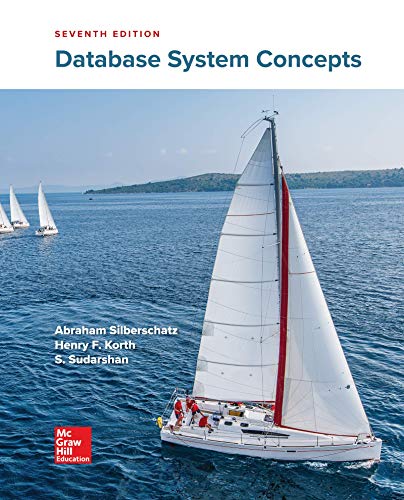
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
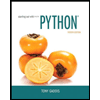
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
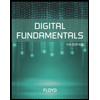
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
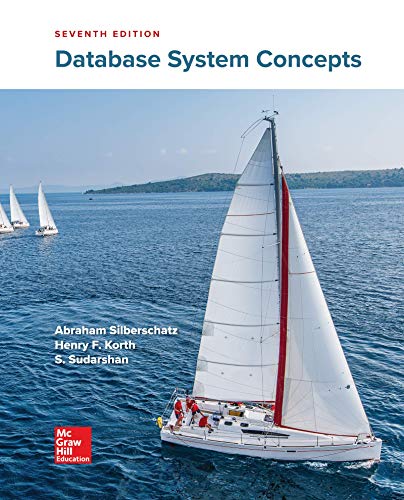
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
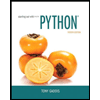
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
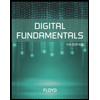
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
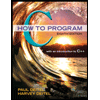
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
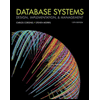
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
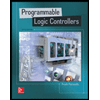
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education