cannot be over than the current balance. Once the amount gets validated, its value will be returned. This function call will be like: withdrawal = get_withdrawal('Enter withdrawal for month' + str(month) + ': current_balance) 3. main() -- the main program The variables and constants are used in the program (main() function) are: # constant TOTAL MONTHS = 3 # variables starting_balance = 0.0, current_balance = 0.0, deposit = 0.0, total_deposit = 0.0, withdrawal = 0.0, total_withdrawal = 0.0 The following is the screen shot of output. Enter starting balance:100 Enter deposit for month 1:-100 Amount cannot be negative!Enter again:100 Enter withdrawal for month 1:500 Withdrawal cannot be negative or greater than balance:50 Enter deposit for month 2:100 Enter withdrawal for month 2:50 Enter deposit for month 3:100 Enter withdrawal for month 3:50 Starting balance:$ 100.0
cannot be over than the current balance. Once the amount gets validated, its value will be returned. This function call will be like: withdrawal = get_withdrawal('Enter withdrawal for month' + str(month) + ': current_balance) 3. main() -- the main program The variables and constants are used in the program (main() function) are: # constant TOTAL MONTHS = 3 # variables starting_balance = 0.0, current_balance = 0.0, deposit = 0.0, total_deposit = 0.0, withdrawal = 0.0, total_withdrawal = 0.0 The following is the screen shot of output. Enter starting balance:100 Enter deposit for month 1:-100 Amount cannot be negative!Enter again:100 Enter withdrawal for month 1:500 Withdrawal cannot be negative or greater than balance:50 Enter deposit for month 2:100 Enter withdrawal for month 2:50 Enter deposit for month 3:100 Enter withdrawal for month 3:50 Starting balance:$ 100.0
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In Python please

Transcribed Image Text:cannot be over than the current balance. Once the amount gets validated, its value
will be returned.
This function call will be like:
withdrawal = get_withdrawal('Enter withdrawal for month' + str(month) + ':
current_balance)
3. main() -- the main program
The variables and constants are used in the program (main() function) are:
# constant
TOTAL_ MONTHS = 3
# variables
starting_balance = 0.0, current_balance = 0.0, deposit = 0.0, total_deposit = 0.0,
withdrawal = 0.0, total_withdrawal = 0.0
The following is the screen shot of output.
Enter starting balance:100
Enter deposit for month 1:-100
Amount cannot be negative! Enter again:100
Enter withdrawal for month 1:500
Withdrawal cannot be negative or greater than balance:50
Enter deposit for month 2:100
Enter withdrawal for month 2:50
Enter deposit for month 3:100
Enter withdrawal for month 3:50
Starting balance:$ 100.0
A

Transcribed Image Text:Write a program that calculates the balance of a checking account at the end of a
three-month period. It should ask the user for the starting balance. Then a loop (for
loop is recommended) should iterate once for every month in the period, performing
the followings:
1. Ask the user for the total amount deposited into the account during that month.
Do not accept negative number. This deposit should be added to the current
balance to make sure current balance is up to date.
2. Ask the user for the total amount withdrawn from the account during that month.
Do not accept negative amount or amount greater than the current balance. The
withdrawal amount should be subtracted from current balance, so current balance
is up to date.
3. Update the total deposit
4. Update the total withdrawal
After the last iteration, the program should display the following information:
1. starting balance at the beginning of the three-month
2. total deposits/withdrawals made during the three-month period
3. final balance (current balance)
Including the following functions in your program:
1. get_amount(msg) - This is the function can be used to ask user input such as
starting balance or current deposit. The parameter msg is a string which will be the
question to pass into the function such as 'Enter starting balance:' or 'Enter deposit
for month 1: The amount user entered cannot be negative number. Once the
amount gets validated, its value will be returned.
This function call will be like: startingBalance = get_amount('Enter starting balance:')
deposit = get_amount('Enter deposit for month' +
str(month)+':')
2. get_withdrawal(msg, balance) - This function is used to ask user input for current
withdrawal. The parameter msg is a string which will be the question to pass into
the function such as 'Enter withdrawal for month 1: The parameter balance will
be the current balance to pass into the function to make sure that withdrawal
balone
Once the amount
stedite uolu
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
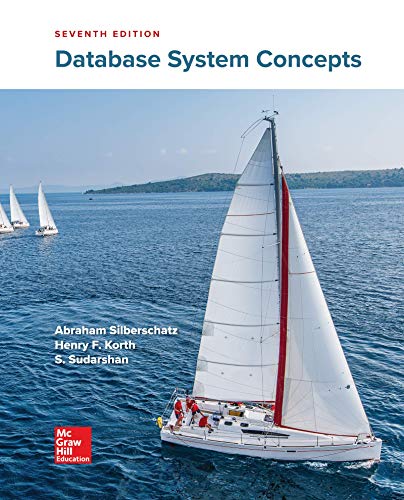
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
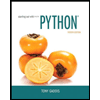
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
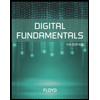
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
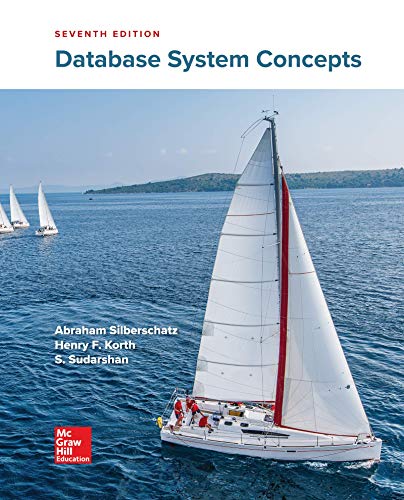
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
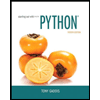
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
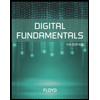
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
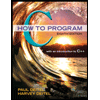
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
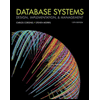
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
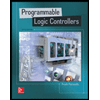
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education