PYTHON CODING Find the Closest Point to the starting point We want to use the formula below to calculate distance between two coodinates: import math import from math sqrt def distance(p1, p2): distance = sqrt (((p1 [0] - p2 [0]) **2) + ((p1 [1] - p2 [1]) **2)) return distance Now we want to find which of the coodinates in the list (remaining_points) is closest to the starting point. def find_closest (start_point, remaining_points): #enter code here return closest_point EXAMPLE INPUT & OUTPUT: find_closest((5,8), [(5.5,3), (3.5,1.5), (2.5,3.5), (7.5,9), (1,3), (7,9.5), (9,2), (1,0), (6,8), (3,7.5), (8,2.5)]) # should return (6, 8)
PYTHON CODING
Find the Closest Point to the starting point
We want to use the formula below to calculate distance between two coodinates:
import math
import from math sqrt
def distance(p1, p2):
distance = sqrt (((p1 [0] - p2 [0]) **2) + ((p1 [1] - p2 [1]) **2))
return distance
Now we want to find which of the coodinates in the list (remaining_points) is closest to the starting point.
def find_closest (start_point, remaining_points):
#enter code here
return closest_point
EXAMPLE INPUT & OUTPUT:
find_closest((5,8), [(5.5,3), (3.5,1.5), (2.5,3.5), (7.5,9), (1,3), (7,9.5), (9,2), (1,0), (6,8), (3,7.5), (8,2.5)])
# should return (6, 8)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Can you show me how you would implement Step 3 within the code please?
Step 3:
a=int(input())
b=int(input())
starting_point=[]
starting_point.append(a)
starting_point.append(b)
remaining_points=[]
for i in range(n):
temp=[]
a=int(input())
b=int(input())
temp.append(a)
temp.append(b)
remaining_points.append(temp) #appending each point as a list
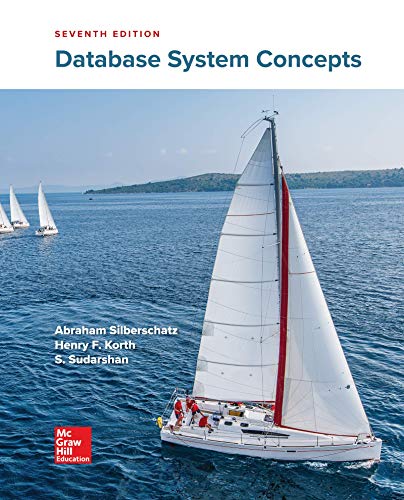
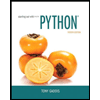
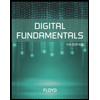
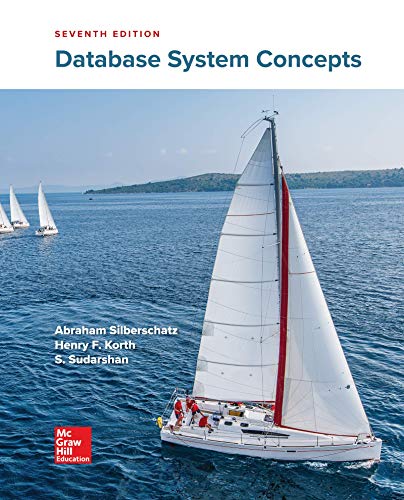
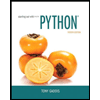
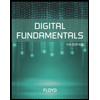
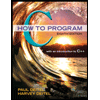
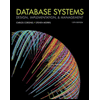
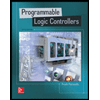