assign, C++ Specifications: 1. Design a class called BankAccount. The member fields of the class are: Account Name, Account Number and Account Balance. There are also other variables called MIN_BALANCE=9.99, REWARDS_AMOUNT=1000.00, REWARDS_RATE=0.04. They look like constants, but for now, they are variables of type double Here is the UML for the class: BankAccount -string accountName // First and Last name of Account holder -int accountNumber // integer -double accountBalance // current balance amount + BankAccount() //default constructor that sets name to "", account number to 0 and balance to 0 +BankAccount(string accountName, int accountNumber, double accountBalance) // regular constructor +getAccountBalance(): double // returns the balance +getAccountName: string // returns name +getAccountNumber: int +setAccountBalance(double amount) : void +withdraw(double amount) : bool //deducts from balance and returns true if resulting balance is less than minimum balance +deposit(double amount): void //adds amount to balance. If amount is greater than rewards amount, calls // addReward method -addReward(double amount) void // adds rewards rate * amount to balance +toString(): String // return the account information as a string with three lines. "Account Name: " name "Account Number:" number "Account Balance:" balance 2. make a file called BankAccountHeader.h which is the class specification file containing the class declaration, member variable declarations and the instance methods prototypes of the BankAccount class as given in the UML diagram above. 3. make a file called BankAccount.cpp which implements the BankAccount class as given in the UML diagram above. The class will have member variables( attributes/data) and instance methods(behaviours/functions that initialize, access and process data) 4. make a driver class BankAccountMain.cpp to do the following: a. Declare and instantiate a bank account called accountZero using the default constructor b. Declare and instantiate a bank account called accountOne with name= "Matilda Patel" number =1232, balance=-4.00 c. Declare and instantiate a bank account called accountTwo with name = "Fernando Diaz", number=1234, balance=250 d. Declare and instantiate a bank account called accountThree with name="Howard Chen", number=1236, balance = 194.56 e. Display the bank accounts in the three line format as above f. Deposit 999 dollars in Fernando's account and 1000.25 in Howards account g. Display their account information h. Withdraw 10000 from Matilda's account and 90 dollars from Fernando's account i. Display the results. If withdrawal is not possible your program should say "Insufficient funds" otherwise it should say "Remaining Balance :" balance amount j. Print the total amount of all the bank accounts created. 5. Note that the main function of the driver is to declare bank objects and to display information. Write a method called display that inputs a string and displays that string to the console. Here is the function header: void display (string information); For now, you may declare the function prototype at the beginning of the driver file, and the function definition at the bottom of the same file. We will separate the function definitions into another function header file when the number gets larger. Your output needs to look like this
m02 assign, C++
Specifications:
1. Design a class called BankAccount. The member fields of the class are: Account Name,
Account Number and Account Balance. There are also other variables called
MIN_BALANCE=9.99, REWARDS_AMOUNT=1000.00, REWARDS_RATE=0.04. They
look like constants, but for now, they are variables of type double
Here is the UML for the class:
BankAccount
-string accountName // First and Last name of Account holder
-int accountNumber // integer
-double accountBalance // current balance amount
+ BankAccount() //default constructor that sets name to "", account number to 0
and balance to 0
+BankAccount(string accountName, int accountNumber, double accountBalance) // regular
constructor
+getAccountBalance(): double // returns the balance
+getAccountName: string // returns name
+getAccountNumber: int
+setAccountBalance(double amount) : void
+withdraw(double amount) : bool //deducts from balance and returns true if resulting balance
is less than minimum balance
+deposit(double amount): void //adds amount to balance. If amount is greater than rewards
amount, calls
// addReward method
-addReward(double amount) void // adds rewards rate * amount to balance
+toString(): String // return the account information as a string with three lines.
"Account Name: " name
"Account Number:" number
"Account Balance:" balance
2. make a file called BankAccountHeader.h which is the class specification file containing
the class declaration, member variable declarations and the instance methods
prototypes of the BankAccount class as given in the UML diagram above.
3. make a file called BankAccount.cpp which implements the BankAccount class as given
in the UML diagram above. The class will have member variables( attributes/data) and
instance methods(behaviours/functions that initialize, access and process data)
4. make a driver class BankAccountMain.cpp to do the following:
a. Declare and instantiate a bank account called accountZero using the default
constructor
b. Declare and instantiate a bank account called accountOne with name= "Matilda
Patel" number =1232, balance=-4.00
c. Declare and instantiate a bank account called accountTwo with name =
"Fernando Diaz", number=1234, balance=250
d. Declare and instantiate a bank account called accountThree with
name="Howard Chen", number=1236, balance = 194.56
e. Display the bank accounts in the three line format as above
f. Deposit 999 dollars in Fernando's account and 1000.25 in Howards account
g. Display their account information
h. Withdraw 10000 from Matilda's account and 90 dollars from Fernando's account
i. Display the results. If withdrawal is not possible your program should say
"Insufficient funds" otherwise it should say "Remaining Balance :" balance
amount
j. Print the total amount of all the bank accounts created.
5. Note that the main function of the driver is to declare bank objects and to display
information. Write a method called display that inputs a string and displays that string to
the console. Here is the function header: void display (string information); For now, you
may declare the function prototype at the beginning of the driver file, and the function
definition at the bottom of the same file. We will separate the function definitions into
another function header file when the number gets larger.
Your output needs to look like this:


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Can you please add an execution chart for the code that was answered by tutors like the example below:
execution chart:
1.0 Main()
2.0 CalculatePropertyTax()
3.0 displayMessage( input string messageToDisplay)
3.1 return double getHomeValue()
3.2 return boolean checkHomeValue()
3.3 return double applyPropertyTax(input double homeValue)
3.4 displayPropertyTax(input homeValue)
3.5 return Boolean queryMoreData()
4.0 displayMessage(input string messageToDisplay)
4.1 return char getYesNo()
4.2 return char convertCase(input char)
3.6 displayErrorMessage()
Can you please add an execution chart of this driver program. Thank you.
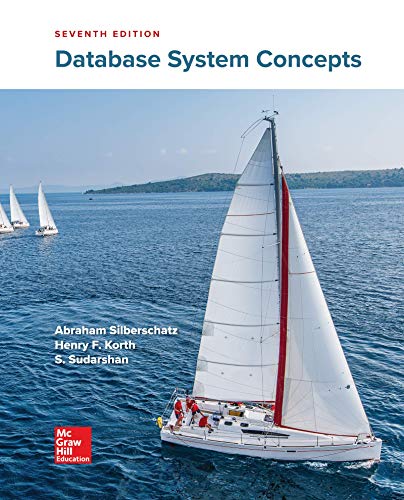
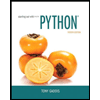
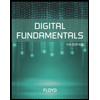
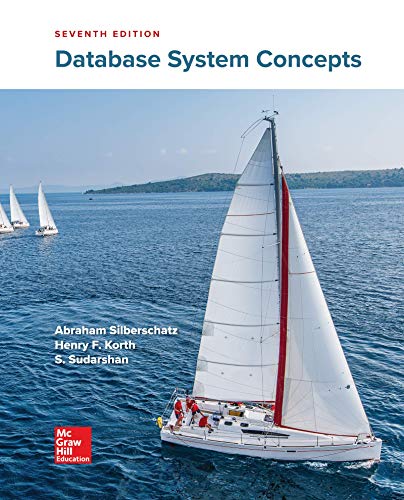
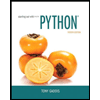
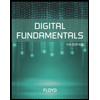
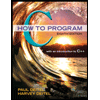
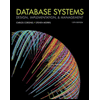
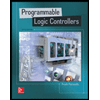