can you make the code down below handle division by zero exception and print the following error: ZeroDivisionError float division by zero print("Please enter the file name :") name = input() # to check whether entered file exists or not try: file = open(name, 'r')#open file in read mode lines = file.readlines()# read data in list of strings in 'lines' list numbers = [] for num in lines: numbers.append(num.strip().split())# change each string in list 'lines' to a list and append to new declarednumber list file.close() # close the file for n in numbers:#numbers becomes a list of list #try block to handle Type error that is flow value must convert into float type try: n[2] = round(float(n[2]),4) #convert n[2] to a float as n[2] contains flow value n.append("{0:.3f}".format(n[2]/3.6)) #append the converted flow value in liter/sec into each list n #try block to handle division by zero error try: sub = round((n[2]-50.285), 4) n.append("{0:.3f}".format(n[2]/sub))#calculate f/(f-50.285) and append it to each list n except ZeroDivisionError: print("cant divide a number with zero") except: print("flow should be a float or integer type")# print if type error occurs file2 = open("FlowProcessed.txt", 'w')#open new file FlowProcessed.txt in write mode file2.write("Date Time (F) m^3/h (F) liters/s X = F/(F-50)\n") file2.write("_______________________________________________________________________________________\n") for n in numbers: if len(n)==5: file2.write("{} {} {} {} {}\n".format(n[0],n[1],n[2],n[3],n[4]))#write the data from numbers list file2.close() except IOError: print('file not found!')#print if file to be read is not found
can you make the code down below handle division by zero exception and print the following error: ZeroDivisionError float division by zero
print("Please enter the file name :")
name = input()
# to check whether entered file exists or not
try:
file = open(name, 'r')#open file in read mode
lines = file.readlines()# read data in list of strings in 'lines' list
numbers = []
for num in lines:
numbers.append(num.strip().split())# change each string in list 'lines' to a list and append to new declarednumber list
file.close() # close the file
for n in numbers:#numbers becomes a list of list
#try block to handle Type error that is flow value must convert into float type
try:
n[2] = round(float(n[2]),4) #convert n[2] to a float as n[2] contains flow value
n.append("{0:.3f}".format(n[2]/3.6)) #append the converted flow value in liter/sec into each list n
#try block to handle division by zero error
try:
sub = round((n[2]-50.285), 4)
n.append("{0:.3f}".format(n[2]/sub))#calculate f/(f-50.285) and append it to each list n
except ZeroDivisionError:
print("cant divide a number with zero")
except:
print("flow should be a float or integer type")# print if type error occurs
file2 = open("FlowProcessed.txt", 'w')#open new file FlowProcessed.txt in write mode
file2.write("Date Time (F) m^3/h (F) liters/s X = F/(F-50)\n")
file2.write("_______________________________________________________________________________________\n")
for n in numbers:
if len(n)==5:
file2.write("{} {} {} {} {}\n".format(n[0],n[1],n[2],n[3],n[4]))#write the data from numbers list
file2.close()
except IOError:
print('file not found!')#print if file to be read is not found

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

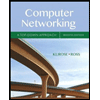
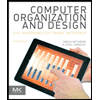
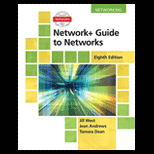
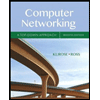
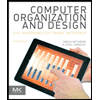
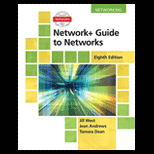
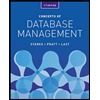
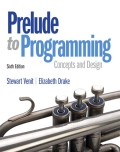
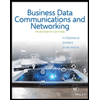