Can you help me write a pseudocode for the following code The language is Java
Can you help me write a pseudocode for the following code The language is Java
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Can you help me write a pseudocode for the following code
The language is Java 
![// Java program for Josephus problem
import java.util.*;
// Josephus class
class Josephus {
public List<Integer> list;
public List<Integer> order;
Josephus (int p){
list = new ArrayList<>();
for (int i-1;i<=p;i++)
list.add (i);
order = new ArrayList<>();
}
// Recursive function
public int josephus (int start, int k)
{
// If size of list is one
// then return its value
if (list.size() == 1) {
return list.get (0);
// Counting kth person and
// check so that it don't go out of bound
start = (start + k) % list.size();
//adding order of survivor
order.add (1ist.get(start));
// Removing the sth person
list.remove (start);
// Calling recursive function again until only one
// person left Start is now the position of previous
// person who is killed Ex. if person at 1-index
// killed then person at 2-index shifted to 1-index
// and counting start from here
return josephus (start, k);
}
public void display(){
order.add(list.get (0));
for (Integer i:order){
System.out.print (i+" ");
}
}
// Main class
class Main{
// Main function
public static void main (String[] args)
{
Scanner sc = new Scanner (System.in);
// Number of people to be executed
System.out.print ( "Enter the total number of persons to be executed:");
int p=sc.nextInt();
System.out.print("\nEnter the starting person to be executed:");
/ Starting person
int k=sc.nextInt ();
System.out.print ("\nEnter the number of persons to be skipped:");
// Number of person to be skipped
int s = sc.nextInt():
// Initialising Josephus object
Josephus jos = new Josephus (p);
// Calling function with starting position at
// k-index so that sth person will be killed
// Storing the safe position
int safePosition = jos.josephus (k-1, s);
//Order in which person were executed
System.out.print ("\norder of victims :");
jos.display ();
// Printing the result
System.out.println("\nsurvivor : " + safePosition);
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F945f572e-3a27-4e3a-a411-d2136e68fdcd%2F12ff7328-8f0b-4974-9ae3-ce4e3a62b501%2Ffxz0e_processed.jpeg&w=3840&q=75)
Transcribed Image Text:// Java program for Josephus problem
import java.util.*;
// Josephus class
class Josephus {
public List<Integer> list;
public List<Integer> order;
Josephus (int p){
list = new ArrayList<>();
for (int i-1;i<=p;i++)
list.add (i);
order = new ArrayList<>();
}
// Recursive function
public int josephus (int start, int k)
{
// If size of list is one
// then return its value
if (list.size() == 1) {
return list.get (0);
// Counting kth person and
// check so that it don't go out of bound
start = (start + k) % list.size();
//adding order of survivor
order.add (1ist.get(start));
// Removing the sth person
list.remove (start);
// Calling recursive function again until only one
// person left Start is now the position of previous
// person who is killed Ex. if person at 1-index
// killed then person at 2-index shifted to 1-index
// and counting start from here
return josephus (start, k);
}
public void display(){
order.add(list.get (0));
for (Integer i:order){
System.out.print (i+" ");
}
}
// Main class
class Main{
// Main function
public static void main (String[] args)
{
Scanner sc = new Scanner (System.in);
// Number of people to be executed
System.out.print ( "Enter the total number of persons to be executed:");
int p=sc.nextInt();
System.out.print("\nEnter the starting person to be executed:");
/ Starting person
int k=sc.nextInt ();
System.out.print ("\nEnter the number of persons to be skipped:");
// Number of person to be skipped
int s = sc.nextInt():
// Initialising Josephus object
Josephus jos = new Josephus (p);
// Calling function with starting position at
// k-index so that sth person will be killed
// Storing the safe position
int safePosition = jos.josephus (k-1, s);
//Order in which person were executed
System.out.print ("\norder of victims :");
jos.display ();
// Printing the result
System.out.println("\nsurvivor : " + safePosition);
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
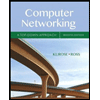
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
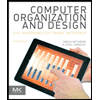
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
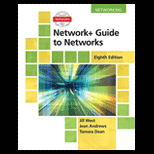
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
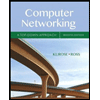
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
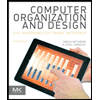
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
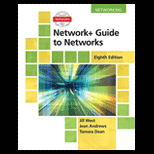
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
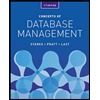
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
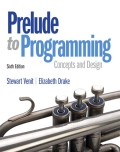
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
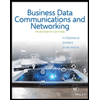
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY