can you explain why the code shown below does not output: pineapple lime and how to fix it when using the driver program shown in screen shot to test. i also attach of the LLNode class // Note: It is important to implement this file as a circular queue without 'front' pointer public class CircularLinkedQueue implements QueueInterface { protected LLNode rear; // reference to the rear of this queue protected int numElements = 0; public CircularLinkedQueue() { rear = null; } public void enqueue(T element) { // Adds element to the rear of this queue. LLNode newNode = new LLNode(element); if (isEmpty()) { rear = newNode; newNode.setLink(newNode); numElements++; } else { rear = newNode; newNode.setLink(rear.getLink()); rear.setLink(newNode); numElements++; } } public T dequeue() { // Throws QueueUnderflowException if this queue is empty; // otherwise, removes front element from this queue and returns it if (isEmpty()) throw new QueueUnderflowException("Dequeue attempted on empty queue"); else { T element; rear = rear.getLink(); element = rear.getInfo(); rear.setInfo(null); if (rear.getLink() == null) rear = null; numElements--; return element; } } public String toString() { String result = "\n"; LLNode cursor = rear; while (cursor != null){ result+=(cursor.getInfo()); cursor = cursor.getLink(); } return result; } public boolean isEmpty() { // Returns true if this queue is empty; otherwise, returns false. return (numElements == 0); } public boolean isFull() { // Returns false - a linked queue is never full. return false; } public int size() { // Returns the number of elements in this queue. return numElements; } }
can you explain why the code shown below does not
output:
pineapple
lime
and how to fix it when using the driver
i also attach of the LLNode class
// Note: It is important to implement this file as a circular queue without 'front' pointer
public class CircularLinkedQueue<T> implements QueueInterface<T> {
protected LLNode<T> rear; // reference to the rear of this queue
protected int numElements = 0;
public CircularLinkedQueue() {
rear = null;
}
public void enqueue(T element) {
// Adds element to the rear of this queue.
LLNode<T> newNode = new LLNode<T>(element);
if (isEmpty()) {
rear = newNode;
newNode.setLink(newNode);
numElements++;
}
else {
rear = newNode;
newNode.setLink(rear.getLink());
rear.setLink(newNode);
numElements++;
}
}
public T dequeue() {
// Throws QueueUnderflowException if this queue is empty;
// otherwise, removes front element from this queue and returns it
if (isEmpty())
throw new QueueUnderflowException("Dequeue attempted on empty queue");
else {
T element;
rear = rear.getLink();
element = rear.getInfo();
rear.setInfo(null);
if (rear.getLink() == null)
rear = null;
numElements--;
return element;
}
}
public String toString() {
String result = "\n";
LLNode<T> cursor = rear;
while (cursor != null){
result+=(cursor.getInfo());
cursor = cursor.getLink();
}
return result;
}
public boolean isEmpty() {
// Returns true if this queue is empty; otherwise, returns false.
return (numElements == 0);
}
public boolean isFull() {
// Returns false - a linked queue is never full.
return false;
}
public int size() {
// Returns the number of elements in this queue.
return numElements;
}
}

![public class Driver {
public static void main(String[] args) {
CircularLinkedQueue<String> cq = new CircularLinkedQueue<String>();
mit
try {
System.out.println(cq.dequeue());
} catch (Exception e) {
menm
ww vm
wwwww
}
cq.enqueue("Tomato");
cg.enqueue("Grape");
cg.dequeue();
cg.dequeue();
cg.engueue("Pineapple");
cg.enqueue("Lime");
System.out.printIn(ca);
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1b2c1e36-9974-49eb-8a0c-fe4d3c635f06%2Fbebb7c5b-b032-4ca8-8919-a966cfe365ad%2Fw28nf34_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

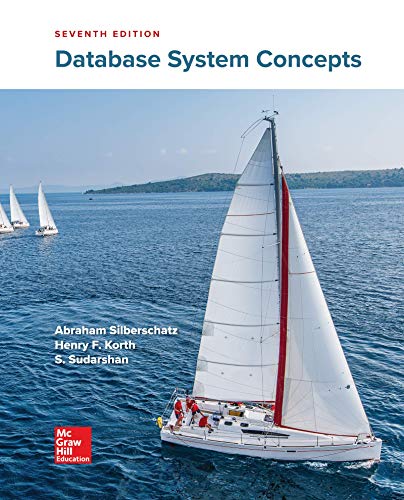
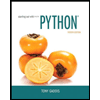
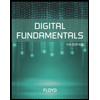
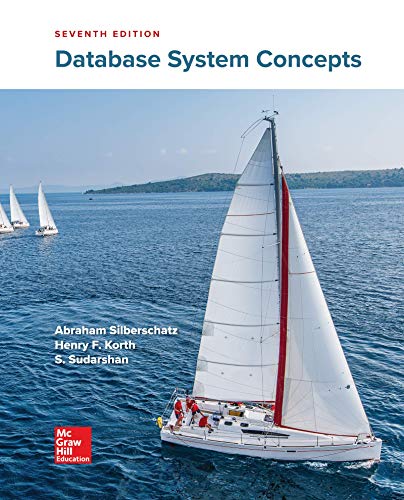
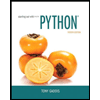
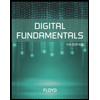
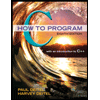
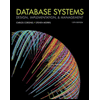
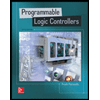