Can someone help me with this? code: public class PigLatinator { /** * Takes a character array and arranges the characters into it's pig-latin form * * The rules for pig-latin are as follows: * 1. If a word starts with a consonant, search through the word beginning at index 0 for the first * instance of a vowel. Split the array at this vowel, moving the consonants to the back of the array, * following a '-'. The add 'ay' at the end of the word. * 2. If a word starts with a vowel add the word "yay" at the end of the word following a '-'. * 3. If a word contains no vowels, just add "ay" at the end of the word following a '-'. * 4. If input is null, return null. * * Hint: Use your isVowel method to cut down on repetitive code * NOTE: Do not use string methods to rearrange your arrays; you should use the indexes * * Example: char[] input = {'h', 'e', 'l', 'l', 'o'} gives * char[] output = {'e', 'l', 'l', 'o', '-', 'h', 'a', 'y'} * char[] input = {'f', 'l', 'a', 'r', 'e'} gives * char[] output = {'a', 'r', 'e', '-', 'f', 'l', 'a', 'y'} * char[] input = {'i', 't'} gives * char[] output = {'i', 't', '-', 'y', 'a', 'y'} * char[] input = {'s', 'h', 'h'} gives * char[] output = {'s', 'h', 'h', '-', 'a', 'y'} * * * @param input The char array to rearrange to pig-latin. * @return A new char array containing the pig-latin of input. If input is null, return null. */ public static char[] pigLatin(char[] input) { return null; } /** * This method is to transform words that begin with a consonant into pig-latin. * If a word starts with a consonant, search through the word beginning at index 0 for the first * instance of a vowel. Split the array at this vowel, moving the consonants to the back of the array, * following a '-'. Then add 'ay' at the end of the word. * If a word contains no vowels, just add "ay" at the end of the word following a '-'. * NOTE: Do not use string methods to rearrange your arrays; you should use the indexes * * @param input - The char array to rearrange to pig-latin. * @return A new char array containing the pig-latin of input. */ public static char[] startsConsonant(char[] input) { return null; } /** * This method is to transform words that begin with a vowel into pig-latin. * If a word starts with a vowel add the word "yay" at the end of the word following a '-'. * NOTE: Do not use string methods to rearrange your arrays; you should use the indexes * * @param input - The char array to rearrange to pig-latin. * @return A new char array containing the pig-latin of input. */ public static char[] startsVowel(char[] input) { return null; } /** * Takes a letter, and checks if it is a vowel, then returns true is yes, false if no. * * Vowels are: a, e, i, o, u * (Note: We are not considering y for this program.) * * @param letter, the letter being checked if it is a vowel * @return A boolean, true if letter is vowel, otherwise false */ public static boolean isVowel(char letter) { return false; } /** * This is test method for the pigLatin() method * * This method already contains 3 tests, but you should consider adding more to * test different input. * */ public static void testPigLatin() { char arr[] = {'f', 'l', 'a', 'r', 'e'}; char arr2[] = {'b', 'o', 'o', 'k'}; char arr3[] = {'i', 't'}; System.out.print("Expected: are-flay\nActual: "); System.out.println(pigLatin(arr)); System.out.print("Expected: ook-bay\nActual: "); System.out.println(pigLatin(arr2)); System.out.print("Expected: it-yay\nActual: "); System.out.println(pigLatin(arr3)); } /** * This is the main method of your program. This is where we will call our test method. * * @param args */ public static void main(String[] args) { testPigLatin(); } }
Can someone help me with this?
code:
public class PigLatinator
{
/**
* Takes a character array and arranges the characters into it's pig-latin form
*
* The rules for pig-latin are as follows:
* 1. If a word starts with a consonant, search through the word beginning at index 0 for the first
* instance of a vowel. Split the array at this vowel, moving the consonants to the back of the array,
* following a '-'. The add 'ay' at the end of the word.
* 2. If a word starts with a vowel add the word "yay" at the end of the word following a '-'.
* 3. If a word contains no vowels, just add "ay" at the end of the word following a '-'.
* 4. If input is null, return null.
*
* Hint: Use your isVowel method to cut down on repetitive code
* NOTE: Do not use string methods to rearrange your arrays; you should use the indexes
*
* Example: char[] input = {'h', 'e', 'l', 'l', 'o'} gives
* char[] output = {'e', 'l', 'l', 'o', '-', 'h', 'a', 'y'}
* char[] input = {'f', 'l', 'a', 'r', 'e'} gives
* char[] output = {'a', 'r', 'e', '-', 'f', 'l', 'a', 'y'}
* char[] input = {'i', 't'} gives
* char[] output = {'i', 't', '-', 'y', 'a', 'y'}
* char[] input = {'s', 'h', 'h'} gives
* char[] output = {'s', 'h', 'h', '-', 'a', 'y'}
*
*
* @param input The char array to rearrange to pig-latin.
* @return A new char array containing the pig-latin of input. If input is null, return null.
*/
public static char[] pigLatin(char[] input)
{
return null;
}
/**
* This method is to transform words that begin with a consonant into pig-latin.
* If a word starts with a consonant, search through the word beginning at index 0 for the first
* instance of a vowel. Split the array at this vowel, moving the consonants to the back of the array,
* following a '-'. Then add 'ay' at the end of the word.
* If a word contains no vowels, just add "ay" at the end of the word following a '-'.
* NOTE: Do not use string methods to rearrange your arrays; you should use the indexes
*
* @param input - The char array to rearrange to pig-latin.
* @return A new char array containing the pig-latin of input.
*/
public static char[] startsConsonant(char[] input) {
return null;
}
/**
* This method is to transform words that begin with a vowel into pig-latin.
* If a word starts with a vowel add the word "yay" at the end of the word following a '-'.
* NOTE: Do not use string methods to rearrange your arrays; you should use the indexes
*
* @param input - The char array to rearrange to pig-latin.
* @return A new char array containing the pig-latin of input.
*/
public static char[] startsVowel(char[] input) {
return null;
}
/**
* Takes a letter, and checks if it is a vowel, then returns true is yes, false if no.
*
* Vowels are: a, e, i, o, u
* (Note: We are not considering y for this program.)
*
* @param letter, the letter being checked if it is a vowel
* @return A boolean, true if letter is vowel, otherwise false
*/
public static boolean isVowel(char letter)
{
return false;
}
/**
* This is test method for the pigLatin() method
*
* This method already contains 3 tests, but you should consider adding more to
* test different input.
*
*/
public static void testPigLatin()
{
char arr[] = {'f', 'l', 'a', 'r', 'e'};
char arr2[] = {'b', 'o', 'o', 'k'};
char arr3[] = {'i', 't'};
System.out.print("Expected: are-flay\nActual: ");
System.out.println(pigLatin(arr));
System.out.print("Expected: ook-bay\nActual: ");
System.out.println(pigLatin(arr2));
System.out.print("Expected: it-yay\nActual: ");
System.out.println(pigLatin(arr3));
}
/**
* This is the main method of your program. This is where we will call our test method.
*
* @param args
*/
public static void main(String[] args)
{
testPigLatin();
}
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

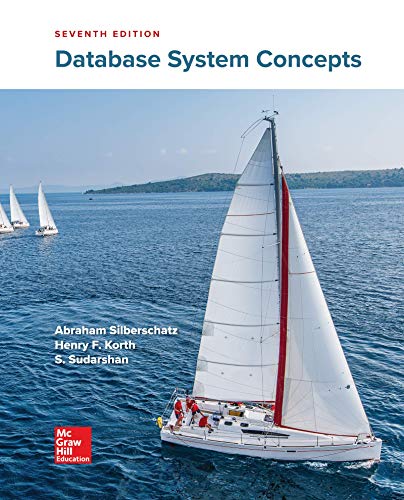
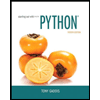
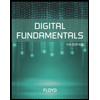
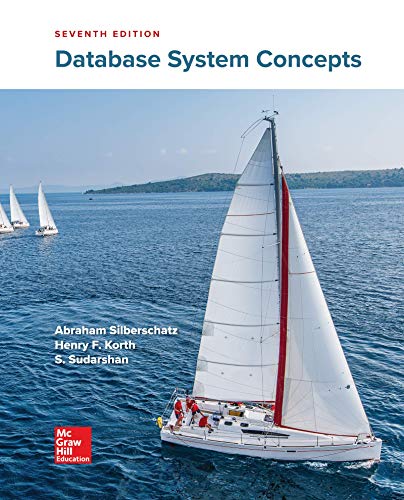
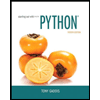
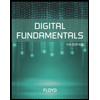
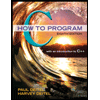
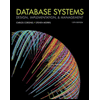
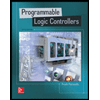