Can someone help me scorrect the C program, no matter what i do it just wont compile and run.It is supposed to come out exactly like the example output .I keep geting these erro codes listed below. CODE THAT NEEDS CORRECTION: #include #include using namespace std; int validate_number(char *str) { while (*str) { if (!isdigit(*str)) { return 0; } str++; } return 1; } int validate(char *ip) { int i, num, dots = 0; char *ptr = strtok(ip, "."); if (ip == NULL) { return 0; } while (ptr) { if (!validate_number(ptr)) { if (!validate_number(ptr)) { return 0; } num = atoi(ptr); if (num >= 0 && num <= 255) { ptr = strtok(NULL, "."); if (ptr != NULL) { dots++; } else { return 0; } } else { return 0; } } if (dots != 3) { return 0; } return 1; } int ipVal(char *ip) { int z = validate(ip); if (z == 1) { return 0; } else { return 1; } } int subVal(char *mask) { int z = validate(mask); if (z == 1) { return 0; } else { return 1; } } int main() { int z, X; char ip[50], mask[50]; cout << "Welcome to ip158." << endl; cout << "IPv4Addressing" << endl; cout << "This program will display the IP address and subnet mask in binary" << endl; cout << "format.. It will also display the network address in binary and in" << endl; cout << "CIDR formats." << endl; cout << "Enter an IP address: "; cin >> ip; cout << "Enter the subnet mask: "; cin >> mask; char ipl[50]; char maskl[50]; strcpy(ipl, ip); strcpy(maskl, mask); z = ipVal(ip); X = subVal(mask); if (z || X) { cout << "Error"; } else { char *i1 = strtok(ipl, "."); char *i2 = strtok(NULL, "."); char *i3 = strtok(NULL, "."); char *i4 = strtok(NULL, "."); int i_1 = atoi(i1); int i_1 = atoi(i1); int i_2 = atoi(i2); int i_3 = atoi(i3); int i_4 = atoi(i4); char *m1 = strtok(maskl, "."); char *m2 = strtok(NULL, "."); char *m3 = strtok(NULL, "."); char *m4 = strtok(NULL, "."); int m_1 = atoi(m1); int m_2 = atoi(m2); int m_3 = atoi(m3); int m_4 = atoi(m4); bitset<8> bset_i_1(i_1); bitset<8> bset_i_2(i_2); bitset<8> bset_i_3(i_3); bitset<8> bset_i_4(i_4); bitset<8> bset_m_1(m_1); bitset<8> bset_m_2(m_2); bitset<8> bset_m_3(m_3); bitset<8> bset_m_4(m_4) ERROR CODES GIVEN DURING COMPILATION: : fatal error: bits/stdc++.h: No such file or directory #include ^ compilation terminated. EXAMPLE OUTPUT: Welcome to ip158. This program will display the IP address and subnet mask in binary format. It will also display the network address in binary and in CIDR formats. Enter an IP address: 10.197.152.8 Enter the subnet mask: 255.240.0.0 IP Address: 00001010 11000101 10011000 00001000 10.197.152.8 Subnet Mask: 11111111 11110000 00000000 00000000 255.240.0.0 Network Add: 00001010 11000000 00000000 00000000 10.192.0.0/12 Thank you for using ip158. Example Output for Errors (user input is in blue) IP address error Enter an ip address: 10.197.352.8 ← 352 is not a valid value The ip address you entered (10.197.352.8) is not valid. Subnet mask error Enter the subnet mask: 255.246.0.0 ← 246 is not a valid value The subnet mask you entered (255.246.0.0) is not valid.
Can someone help me scorrect the C program, no matter what i do it just wont compile and run.It is supposed to come out exactly like the example output .I keep geting these erro codes listed below.
CODE THAT NEEDS CORRECTION:
#include <bits/stdc++.h>
#include <bitset>
using namespace std;
int validate_number(char *str) {
while (*str) {
if (!isdigit(*str)) {
return 0;
}
str++;
}
return 1;
}
int validate(char *ip) {
int i, num, dots = 0;
char *ptr = strtok(ip, ".");
if (ip == NULL) {
return 0;
}
while (ptr) {
if (!validate_number(ptr)) {
if (!validate_number(ptr)) {
return 0;
}
num = atoi(ptr);
if (num >= 0 && num <= 255) {
ptr = strtok(NULL, ".");
if (ptr != NULL) {
dots++;
} else {
return 0;
}
} else {
return 0;
}
}
if (dots != 3) {
return 0;
}
return 1;
}
int ipVal(char *ip) {
int z = validate(ip);
if (z == 1) {
return 0;
} else {
return 1;
}
}
int subVal(char *mask) {
int z = validate(mask);
if (z == 1) {
return 0;
} else {
return 1;
}
}
int main() {
int z, X;
char ip[50], mask[50];
cout << "Welcome to ip158." << endl;
cout << "IPv4Addressing" << endl;
cout << "This program will display the IP address and subnet mask in binary" << endl;
cout << "format.. It will also display the network address in binary and in" << endl;
cout << "CIDR formats." << endl;
cout << "Enter an IP address: ";
cin >> ip;
cout << "Enter the subnet mask: ";
cin >> mask;
char ipl[50];
char maskl[50];
strcpy(ipl, ip);
strcpy(maskl, mask);
z = ipVal(ip);
X = subVal(mask);
if (z || X) {
cout << "Error";
} else {
char *i1 = strtok(ipl, ".");
char *i2 = strtok(NULL, ".");
char *i3 = strtok(NULL, ".");
char *i4 = strtok(NULL, ".");
int i_1 = atoi(i1);
int i_1 = atoi(i1);
int i_2 = atoi(i2);
int i_3 = atoi(i3);
int i_4 = atoi(i4);
char *m1 = strtok(maskl, ".");
char *m2 = strtok(NULL, ".");
char *m3 = strtok(NULL, ".");
char *m4 = strtok(NULL, ".");
int m_1 = atoi(m1);
int m_2 = atoi(m2);
int m_3 = atoi(m3);
int m_4 = atoi(m4);
bitset<8> bset_i_1(i_1);
bitset<8> bset_i_2(i_2);
bitset<8> bset_i_3(i_3);
bitset<8> bset_i_4(i_4);
bitset<8> bset_m_1(m_1);
bitset<8> bset_m_2(m_2);
bitset<8> bset_m_3(m_3);
bitset<8> bset_m_4(m_4)
ERROR CODES GIVEN DURING COMPILATION:
: fatal error: bits/stdc++.h: No such file or directory
#include <bits/stdc++.h>
^
compilation terminated.
EXAMPLE OUTPUT:
Welcome to ip158.
This program will display the IP address and subnet mask in binary
format. It will also display the network address in binary and in
CIDR formats.
Enter an IP address: 10.197.152.8
Enter the subnet mask: 255.240.0.0
IP Address: 00001010 11000101 10011000 00001000 10.197.152.8
Subnet Mask: 11111111 11110000 00000000 00000000 255.240.0.0
Network Add: 00001010 11000000 00000000 00000000 10.192.0.0/12
Thank you for using ip158.
Example Output for Errors (user input is in blue)
IP address error
Enter an ip address: 10.197.352.8 ← 352 is not a valid value
The ip address you entered (10.197.352.8) is not valid.
Subnet mask error
Enter the subnet mask: 255.246.0.0 ← 246 is not a valid value
The subnet mask you entered (255.246.0.0) is not valid.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

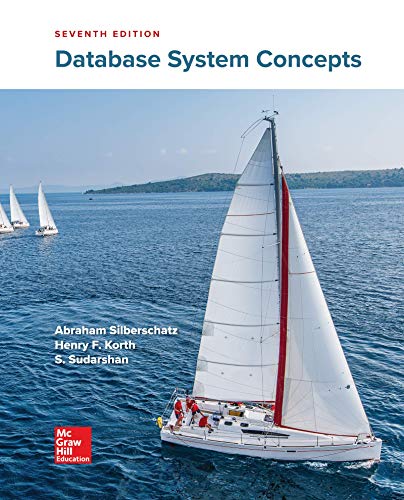
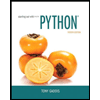
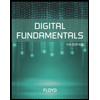
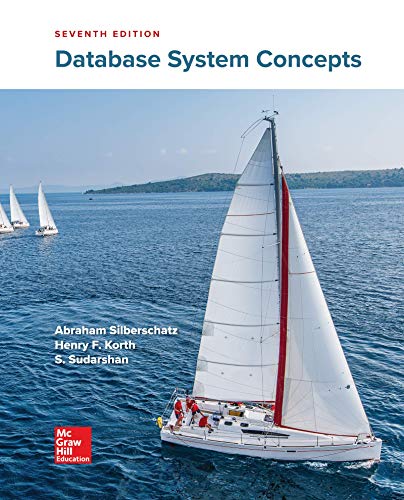
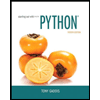
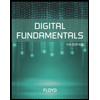
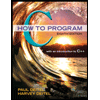
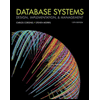
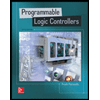