Call your class BigFraction.java and implement it according to this specification. You will note that BigFraction is composed of BigInteger numerator and BigInteger denominator. Like BigInteger and BigDecimal, BigFraction is immutable. Also, take care to simplify all fractions during construction using the BigInteger gcd method and dividing both numerator and denominator by their greatest common divisor, noting that BigInteger has its own given gcd method. In our specification, the denominator is always positive; only the numerator can be either positive or negative. For a refresher on fraction arithmetic, see here. Field Detail ONE public static final BigFraction ONE ZERO public static final BigFraction ZERO Constructor Detail BigFraction public BigFraction(java.math.BigInteger numerator, java.math.BigInteger denominator) Given a BigInteger numerator and denominator, simplify the fraction by dividing both numerator and denominator by their greatest common divisor, and change signs such that the denominator is always positive. (The numerator may be negative.) Remember that the BigInteger class contains a gcd method. Parameters:numerator - BigInteger numerator of the BigFractiondenominator - BigInteger denominator of the BigFraction BigFraction public BigFraction(BigFraction f) Given a BigFraction, copy its BigInteger numerator and denominator fields to the fields of the new BigFraction object. Parameters:f - given BigFraction object to copy in this constructor BigFraction public BigFraction(java.lang.String s) Given a String consisting of two integers separated by a single '/', initialize the numerator and denominator fields with BigIntegers constructed with the substrings before and after the '/', respectively. Parameters:s - a String representation of a fraction consisting of two integers separated by a single '/' BigFraction public BigFraction(long numerator, long denominator) Construct a BigFraction given two long numerator and denominator values, convert and assign them to BigInteger numerator and denominator values. Parameters:numerator - given long numerator valuedenominator - given long denominator value Method Detail getNum public java.math.BigInteger getNum() Return the BigInteger numerator of the BigFraction. Returns:the BigInteger numerator of the BigFraction getDen public java.math.BigInteger getDen() Return the BigInteger denominator of the BigFraction. Returns:the BigInteger denominator of the BigFraction toString public java.lang.String toString() Return a String consisting of the numerator and denominator separated by a single '/'. Overrides:toString in class java.lang.ObjectReturns:a String consisting of the numerator and denominator separated by a single '/' asBigDecimal public java.math.BigDecimal asBigDecimal(int scale, java.math.RoundingMode roundingMode) Return a BigDecimal formed by converting the BigInteger numerator and denominator to BigDecimals and then dividing the BigDecimal numerator by the BigDecimal denominator using the given scale and rounding mode. Parameters:scale - the number of decimal places for the division computationroundingMode - rounding mode for determining the correct final digit of the division result at the given scaleReturns:a BigDecimal formed by converting the BigInteger numerator and denominator to BigDecimals and then dividing the BigDecimal numerator by the BigDecimal denominator using the given scale and rounding mode negate public BigFraction negate() Return a new BigFraction object with the same denominator and the negated numerator. Note that BigInteger has a negate method. Returns:a new BigFraction object with the same denominator and the negated numerator add public BigFraction add(BigFraction b) Return a BigFraction resulting from the addition of a given BigFraction b to this BigFraction. Parameters:b - given BigFraction to add to this BigFractionReturns:a BigFraction resulting from the addition of this BigFraction to given BigFraction b subtract public BigFraction subtract(BigFraction b) Return a BigFraction resulting from the subtraction of a given BigFraction b from this BigFraction. Parameters:b - given BigFraction to subtract from this BigFractionReturns:a BigFraction resulting from the subtraction of a given BigFraction b from this BigFraction multiply public BigFraction multiply(BigFraction b) Return a BigFraction resulting from the multiplication of a given BigFraction b with this BigFraction. Parameters:b - given BigFraction to multiply with this BigFractionReturns:a BigFraction resulting from the multiplication of a given BigFraction b with this BigFraction divide public BigFraction divide(BigFraction b) Return a BigFraction resulting from the division of a given BigFraction b into this BigFraction. Parameters:b - given BigFraction to divide into this BigFractionReturns:a BigFraction resulting from the division of a given BigFraction b into this BigFraction
Call your class BigFraction.java and implement it according to this specification. You will note that BigFraction is composed of BigInteger numerator and BigInteger denominator. Like BigInteger and BigDecimal, BigFraction is immutable. Also, take care to simplify all fractions during construction using the BigInteger gcd method and dividing both numerator and denominator by their greatest common divisor, noting that BigInteger has its own given gcd method. In our specification, the denominator is always positive; only the numerator can be either positive or negative. For a refresher on fraction arithmetic, see here. Field Detail ONE public static final BigFraction ONE ZERO public static final BigFraction ZERO Constructor Detail BigFraction public BigFraction(java.math.BigInteger numerator, java.math.BigInteger denominator) Given a BigInteger numerator and denominator, simplify the fraction by dividing both numerator and denominator by their greatest common divisor, and change signs such that the denominator is always positive. (The numerator may be negative.) Remember that the BigInteger class contains a gcd method. Parameters:numerator - BigInteger numerator of the BigFractiondenominator - BigInteger denominator of the BigFraction BigFraction public BigFraction(BigFraction f) Given a BigFraction, copy its BigInteger numerator and denominator fields to the fields of the new BigFraction object. Parameters:f - given BigFraction object to copy in this constructor BigFraction public BigFraction(java.lang.String s) Given a String consisting of two integers separated by a single '/', initialize the numerator and denominator fields with BigIntegers constructed with the substrings before and after the '/', respectively. Parameters:s - a String representation of a fraction consisting of two integers separated by a single '/' BigFraction public BigFraction(long numerator, long denominator) Construct a BigFraction given two long numerator and denominator values, convert and assign them to BigInteger numerator and denominator values. Parameters:numerator - given long numerator valuedenominator - given long denominator value Method Detail getNum public java.math.BigInteger getNum() Return the BigInteger numerator of the BigFraction. Returns:the BigInteger numerator of the BigFraction getDen public java.math.BigInteger getDen() Return the BigInteger denominator of the BigFraction. Returns:the BigInteger denominator of the BigFraction toString public java.lang.String toString() Return a String consisting of the numerator and denominator separated by a single '/'. Overrides:toString in class java.lang.ObjectReturns:a String consisting of the numerator and denominator separated by a single '/' asBigDecimal public java.math.BigDecimal asBigDecimal(int scale, java.math.RoundingMode roundingMode) Return a BigDecimal formed by converting the BigInteger numerator and denominator to BigDecimals and then dividing the BigDecimal numerator by the BigDecimal denominator using the given scale and rounding mode. Parameters:scale - the number of decimal places for the division computationroundingMode - rounding mode for determining the correct final digit of the division result at the given scaleReturns:a BigDecimal formed by converting the BigInteger numerator and denominator to BigDecimals and then dividing the BigDecimal numerator by the BigDecimal denominator using the given scale and rounding mode negate public BigFraction negate() Return a new BigFraction object with the same denominator and the negated numerator. Note that BigInteger has a negate method. Returns:a new BigFraction object with the same denominator and the negated numerator add public BigFraction add(BigFraction b) Return a BigFraction resulting from the addition of a given BigFraction b to this BigFraction. Parameters:b - given BigFraction to add to this BigFractionReturns:a BigFraction resulting from the addition of this BigFraction to given BigFraction b subtract public BigFraction subtract(BigFraction b) Return a BigFraction resulting from the subtraction of a given BigFraction b from this BigFraction. Parameters:b - given BigFraction to subtract from this BigFractionReturns:a BigFraction resulting from the subtraction of a given BigFraction b from this BigFraction multiply public BigFraction multiply(BigFraction b) Return a BigFraction resulting from the multiplication of a given BigFraction b with this BigFraction. Parameters:b - given BigFraction to multiply with this BigFractionReturns:a BigFraction resulting from the multiplication of a given BigFraction b with this BigFraction divide public BigFraction divide(BigFraction b) Return a BigFraction resulting from the division of a given BigFraction b into this BigFraction. Parameters:b - given BigFraction to divide into this BigFractionReturns:a BigFraction resulting from the division of a given BigFraction b into this BigFraction
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Call your class BigFraction.java and implement it according to this specification. You will note that BigFraction is composed of BigInteger numerator and BigInteger denominator. Like BigInteger and BigDecimal, BigFraction is immutable. Also, take care to simplify all fractions during construction using the BigInteger gcd method and dividing both numerator and denominator by their greatest common divisor, noting that BigInteger has its own given gcd method. In our specification, the denominator is always positive; only the numerator can be either positive or negative. For a refresher on fraction arithmetic, see here.
-
Field Detail
-
ONE
public static final BigFraction ONE
-
ZERO
public static final BigFraction ZERO
-
-
Constructor Detail
-
BigFraction
public BigFraction(java.math.BigInteger numerator, java.math.BigInteger denominator)Given a BigInteger numerator and denominator, simplify the fraction by dividing both numerator and denominator by their greatest common divisor, and change signs such that the denominator is always positive. (The numerator may be negative.) Remember that the BigInteger class contains a gcd method.Parameters:numerator - BigInteger numerator of the BigFractiondenominator - BigInteger denominator of the BigFraction
-
BigFraction
public BigFraction(BigFraction f)Given a BigFraction, copy its BigInteger numerator and denominator fields to the fields of the new BigFraction object.Parameters:f - given BigFraction object to copy in this constructor
-
BigFraction
public BigFraction(java.lang.String s)Given a String consisting of two integers separated by a single '/', initialize the numerator and denominator fields with BigIntegers constructed with the substrings before and after the '/', respectively.Parameters:s - a String representation of a fraction consisting of two integers separated by a single '/'
-
BigFraction
public BigFraction(long numerator, long denominator)Construct a BigFraction given two long numerator and denominator values, convert and assign them to BigInteger numerator and denominator values.Parameters:numerator - given long numerator valuedenominator - given long denominator value
-
-
Method Detail
-
getNum
public java.math.BigInteger getNum()Return the BigInteger numerator of the BigFraction.Returns:the BigInteger numerator of the BigFraction
-
getDen
public java.math.BigInteger getDen()Return the BigInteger denominator of the BigFraction.Returns:the BigInteger denominator of the BigFraction
-
toString
public java.lang.String toString()Return a String consisting of the numerator and denominator separated by a single '/'.Overrides:toString in class java.lang.ObjectReturns:a String consisting of the numerator and denominator separated by a single '/'
-
asBigDecimal
public java.math.BigDecimal asBigDecimal(int scale, java.math.RoundingMode roundingMode)Return a BigDecimal formed by converting the BigInteger numerator and denominator to BigDecimals and then dividing the BigDecimal numerator by the BigDecimal denominator using the given scale and rounding mode.Parameters:scale - the number of decimal places for the division computationroundingMode - rounding mode for determining the correct final digit of the division result at the given scaleReturns:a BigDecimal formed by converting the BigInteger numerator and denominator to BigDecimals and then dividing the BigDecimal numerator by the BigDecimal denominator using the given scale and rounding mode
-
negate
public BigFraction negate()Return a new BigFraction object with the same denominator and the negated numerator. Note that BigInteger has a negate method.Returns:a new BigFraction object with the same denominator and the negated numerator
-
add
public BigFraction add(BigFraction b)Return a BigFraction resulting from the addition of a given BigFraction b to this BigFraction.Parameters:b - given BigFraction to add to this BigFractionReturns:a BigFraction resulting from the addition of this BigFraction to given BigFraction b
-
subtract
public BigFraction subtract(BigFraction b)Return a BigFraction resulting from the subtraction of a given BigFraction b from this BigFraction.Parameters:b - given BigFraction to subtract from this BigFractionReturns:a BigFraction resulting from the subtraction of a given BigFraction b from this BigFraction
-
multiply
public BigFraction multiply(BigFraction b)Return a BigFraction resulting from the multiplication of a given BigFraction b with this BigFraction.Parameters:b - given BigFraction to multiply with this BigFractionReturns:a BigFraction resulting from the multiplication of a given BigFraction b with this BigFraction
-
divide
public BigFraction divide(BigFraction b)Return a BigFraction resulting from the division of a given BigFraction b into this BigFraction.Parameters:b - given BigFraction to divide into this BigFractionReturns:a BigFraction resulting from the division of a given BigFraction b into this BigFraction
-
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
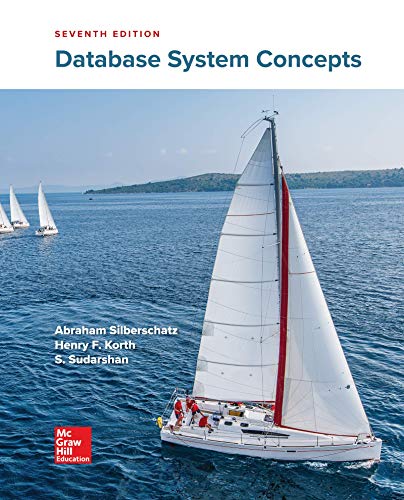
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
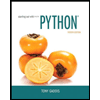
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
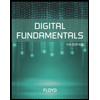
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
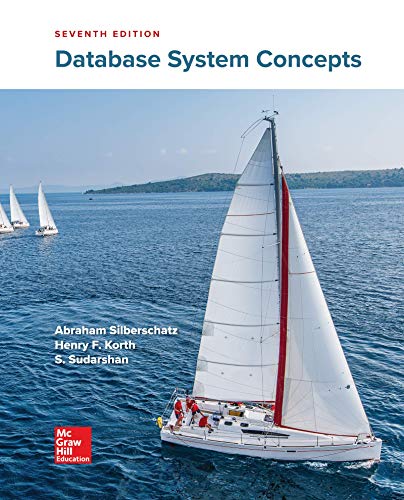
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
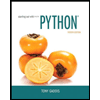
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
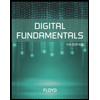
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
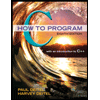
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
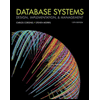
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
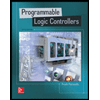
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education