C++ Write a program that uses a stack to print the prime factors of a positive integer (input by the user) in descending order.
C++ Write a program that uses a stack to print the prime factors of a positive integer (input by the user) in descending order.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
C++
Write a program that uses a stack to print the prime factors of a positive integer (input by the user) in descending order.

Transcribed Image Text:Instructions
Instructions
Write a program that uses a stack to print the prime factors of a positive
integer (input by the user) in descending order.
Grading
When you have completed your program, click the Submit button to
record your score.
linkedStack.h
1 //Header File: linkedStack.h
2
3 #ifndef H_StackType
4 #define H_StackType
5
6 #include <iostream>
7 #include <cassert>
8 #include "stackADT.h"
9 using namespace std;
10 template <class Type>
11 struct node Type
12 {
13
25
26
main.cpp
27
28
14
15 };
16 template <class Type>
17 class linkedStackType: public stackADT<Type>
18 {
19 public:
20
21
22
23
24
29
30
Type info;
nodeType<Type> *link;
33
34 };
stackADT.h
const linkedStackType<Type>& operator=
bool isEmptyStack() const;
bool isFullStack() const;
Type top() const;
void pop();
linkedStackType();
31 private:
32
void initializeStack();
void push(const Type& newItem);
(const linkedStackType<Type>&);
| +
linkedStackType(const linkedStackType<Type>& otherStack);
~linkedStackType();
nodeType<Type> *stackTop; //pointer to the stack
void copyStack (const linkedStackType<Type>& otherStack);
35 template <class Type>
36 1inkedStackType<Type> ::linkedStackType()
37
stackTop nullptr;
}
template <class Type>
bool linkedStackType<Type>::LsEmptyStack() const
{
return(stackTop== nullptr);
}//end isEmptyStack
template <class Type>
bool linkedStackType<Type>:: isFullStack() corst
{
return false;
}//end isFullStack
template <class Type>
void linkedStackType<Type>:: initializeStack()
{
node Type<Type> *temp; //pointer to delete the rode
while (stackTop != nullptr) //while there are elements in
//the stack
{
temp = stackToo;
//set temp to point to the
//currert noce
stackTop stackTop->link: //advance stackTop to the
//next noce
delete temp;
//deallocate memory occupied by temp
}
}//end initialize Stack
template <class Type>
void linkedStackType<Type>:: push(corst Type< rewElement)
{
node Type<Type> *newNode; //poirter to create the new node
newNode = new nodeType<Type>; //create the node
linkedStack.h
main.cpp
1 //Header file: stackADT.h
2
3 #ifndef H_StackADT
4 #define H_StackADT
5
6 template <class Type>
7 class stackADT
8
9 public:
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25;
8}
9
virtual void initializeStack() = 0;
virtual bool isEmptyStack() const = 0;
virtual bool isFullStack() const = 0;
virtual void push(const Type& newItem) = 0;
virtual Type top() const = 0;
virtual void pop() = 0;
linkedStack.h
1 #include <iostream>
2
3 using namespace std;
4
5 int main() {
6
7
stackADT.h
main.cpp
// Write your main here
return 0;
stackADT.h
+
| +
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
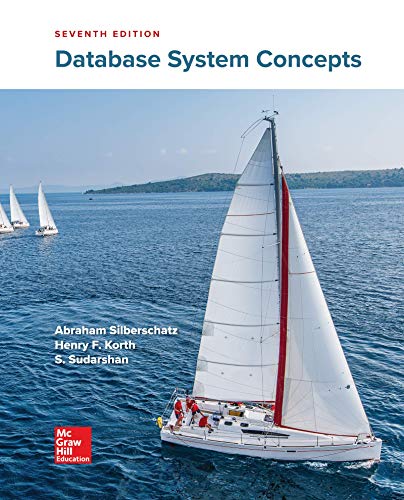
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
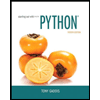
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
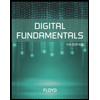
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
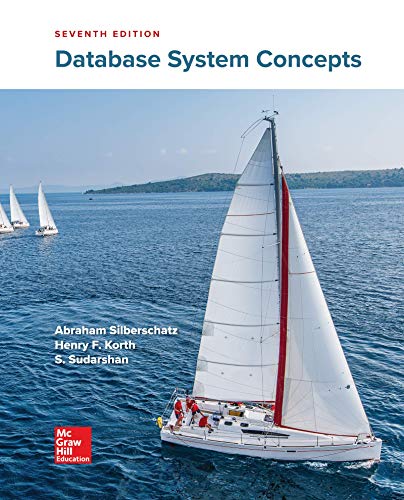
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
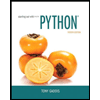
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
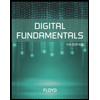
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
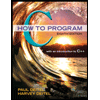
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
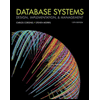
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
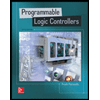
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education