C++ starter code /* Cipher Lab using C strings */
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
C++ starter code
/* Cipher Lab using C strings */
/* Running the program looks like:
Enter some lower-case text: hello
Shifting 0 gives: hello
Shifting 1 gives: ifmmp
Shifting 2 gives: jgnnq
Shifting 3 gives: khoor
Shifting 4 gives: lipps
Shifting 5 gives: mjqqt
Shifting 6 gives: nkrru
Shifting 7 gives: olssv
Shifting 8 gives: pmttw
Shifting 9 gives: qnuux
Shifting 10 gives: rovvy
Shifting 11 gives: spwwz
Shifting 12 gives: tqxxa
Shifting 13 gives: uryyb
Shifting 14 gives: vszzc
Shifting 15 gives: wtaad
Shifting 16 gives: xubbe
Shifting 17 gives: yvccf
Shifting 18 gives: zwddg
Shifting 19 gives: axeeh
Shifting 20 gives: byffi
Shifting 21 gives: czggj
Shifting 22 gives: dahhk
Shifting 23 gives: ebiil
Shifting 24 gives: fcjjm
Shifting 25 gives: gdkkn
*/
#include <iostream>
#include <iomanip>
#include <cctype>
using namespace std;
// Global constants
const int MaxWordSize = 81; // 80 characters + 1 for NULL
// Given an array of characters and a shift value:
// shift each character in the original text by some amount,
// storing the result into the shiftedText array.
// Remember: Wrap around at the end of the alphabet.
// *** In the line below you must supply the function
// return type and the parameter(s) ***
? shiftTheText( ? )
{
// Loop through each character in the C string, startingText
// When the character is an alphabetic character,
// shift it by adding the shift value.
// Then store the resulting character in its proper spot
// in the shiftedText C string.
}
int main()
{
// Initialize the variables
char startingText[ MaxWordSize];
char shiftedText[ MaxWordSize];
cout << "Enter some lower-case text: ";
cin >> startingText;
for( int shiftValue = 0; shiftValue < 26; shiftValue++) {
// In the function call below you need to pass the starting text array, the shift value, and the shifted text array.
shiftTheText( );
cout << "Shifting " << setw( 2) << shiftValue << " gives: " << shiftedText << endl;
}
return 0; // Keep C++ happy
}// end main()


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

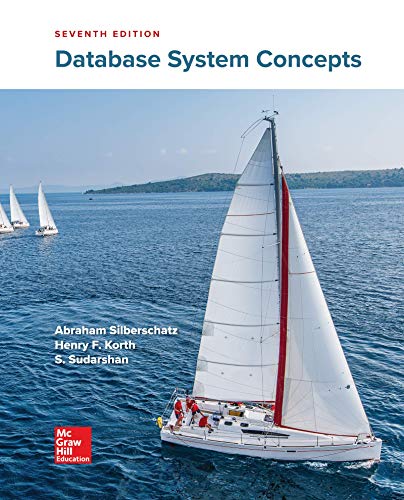
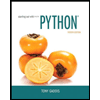
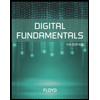
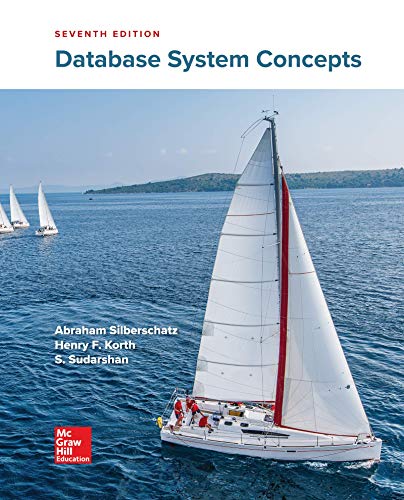
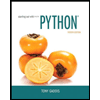
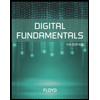
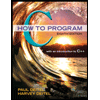
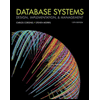
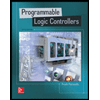