C++ Programming Instructions Write a program that prompts the user for a sequence of characters (all typed on a single line) and counts the number of vowels ('a', 'e', 'i', 'o', 'u'), consonants, and any other characters that appear in the input. The user terminates input by typing either the period (.) or exclamation mark character (!) followed by the Enter key on your keyboard. Your program will not count white space characters.I.e., you will ignore white space characters.Though, the cin statement will skip white space characters in the input for you. Your program will not be case-sensitive. Thus, the characters 'a' and 'A' are both vowels and the characters 'b' and 'B' are both consonants.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
C++ Programming Instructions
Write a program that prompts the user for a sequence of characters (all typed on a single line) and counts the number of vowels ('a', 'e', 'i', 'o', 'u'), consonants, and any other characters that appear in the input. The user terminates input by typing either the period (.) or exclamation mark character (!) followed by the Enter key on your keyboard.
- Your program will not count white space characters.I.e., you will ignore white space characters.Though, the cin statement will skip white space characters in the input for you.
- Your program will not be case-sensitive. Thus, the characters 'a' and 'A' are both vowels and the characters 'b' and 'B' are both consonants.
For example, the input How? 1, 2, 3. contains one vowel, two consonants, and six other kinds of characters.
IMPORTANT: Your program must use the while statement only when looping. I.e., do not use a for statement or any other looping statement.
Test 1
> run
Enter text:
.
Your sentence has 0 letter(s).
Number of a's: 0
Number of e's: 0
Number of i's: 0
Number of o's: 0
Number of u's: 0
Number of consonants: 0
Number of digits: 0
Number of other characters: 0
Vowels make up 0% of the sentence.
Test 2
> run
Enter text:
!
Your sentence has 0 letter(s).
Number of a's: 0
Number of e's: 0
Number of i's: 0
Number of o's: 0
Number of u's: 0
Number of consonants: 0
Number of digits: 0
Number of other characters: 0
Vowels make up 0% of the sentence.
Test 3
> run
Enter text:
a.
Your sentence has 1 letter(s).
Number of a's: 1
Number of e's: 0
Number of i's: 0
Number of o's: 0
Number of u's: 0
Number of consonants: 0
Number of digits: 0
Number of other characters: 0
Vowels make up 100.00% of the sentence.
Test 4
> run
Enter text:
ia!
Your sentence has 2 letter(s).
Number of a's: 1
Number of e's: 0
Number of i's: 1
Number of o's: 0
Number of u's: 0
Number of consonants: 0
Number of digits: 0
Number of other characters: 0
Vowels make up 100.00% of the sentence.
Test 5
> run
Enter text:
apple.
Your sentence has 5 letter(s).
Number of a's: 1
Number of e's: 1
Number of i's: 0
Number of o's: 0
Number of u's: 0
Number of consonants: 3
Number of digits: 0
Number of other characters: 0
Vowels make up 40.00% of the sentence.
Test 6
> run
Enter text:
orange#pear!
Your sentence has 11 letter(s).
Number of a's: 2
Number of e's: 2
Number of i's: 0
Number of o's: 1
Number of u's: 0
Number of consonants: 5
Number of digits: 0
Number of other characters: 1
Vowels make up 45.45% of the sentence.
Test 7
> run
Enter text:
JacKsoN,MiSsiSSipPI.
Your sentence has 19 letter(s).
Number of a's: 1
Number of e's: 0
Number of i's: 4
Number of o's: 1
Number of u's: 0
Number of consonants: 12
Number of digits: 0
Number of other characters: 1
Vowels make up 31.58% of the sentence.
Test 8
> run
Enter text:
OsU ruLez, Cols, OH.
Your sentence has 16 letter(s).
Number of a's: 0
Number of e's: 1
Number of i's: 0
Number of o's: 3
Number of u's: 2
Number of consonants: 8
Number of digits: 0
Number of other characters: 2
Vowels make up 37.50% of the sentence.
Test 9
> run
Enter text:
Equation 5 * 3 - 2 / (3 % 5).
Your sentence has 19 letter(s).
Number of a's: 1
Number of e's: 1
Number of i's: 1
Number of o's: 1
Number of u's: 1
Number of consonants: 3
Number of digits: 5
Number of other characters: 6
Vowels make up 26.32% of the sentence.
Test 10
> run
Enter text:
You passed the final test :) AWESOME.
Your sentence has 30 letter(s).
Number of a's: 3
Number of e's: 5
Number of i's: 1
Number of o's: 2
Number of u's: 1
Number of consonants: 16
Number of digits: 0
Number of other characters: 2
Vowels make up 40.00% of the sentence.
\



Use strlength () function to count the number of characters .
Below is the code:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

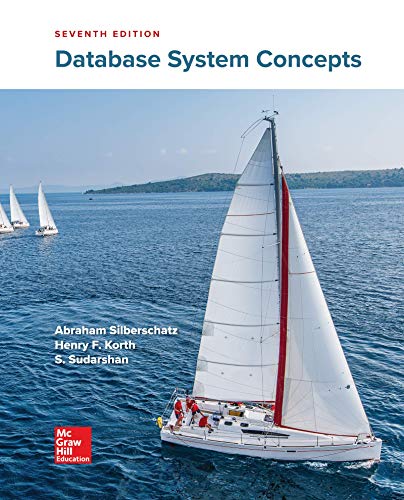
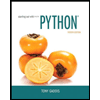
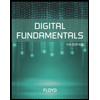
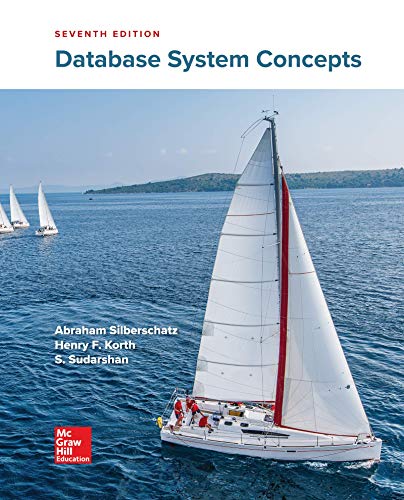
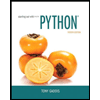
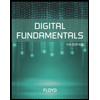
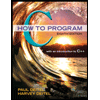
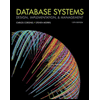
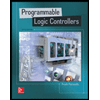