C Program: An integer number is said to be a perfect number if its factors, including 1 (but not the number itself), sum to the number. For example, 6 is a perfect number because 6 = 1 + 2 + 3. I have written a function called isPerfect (see below), that determines whether parameter passed to the function is a perfect number. Use this function in a C program that determines and prints all the perfect numbers between 1 and 1000. Print the factors of each perfect number to confirm that the number is indeed perfect. // isPerfect returns true if value is perfect integer, // i.e., if value is equal to sum of its factors int isPerfect(int value) { int factorSum = 1; // current sum of factors // loop through possible factor values for (int i = 2; i <= value / 2; ++i) { // if i is factor if (value % i == 0) { factorSum += i; // add to sum } } // return true if value is equal to sum of factors if (factorSum == value) { return 1; } else { return 0; } }
C
An integer number is said to be a perfect number if its factors, including 1 (but not the number itself), sum to the number. For example, 6 is a perfect number because 6 = 1 + 2 + 3. I have written a function called isPerfect (see below), that determines whether parameter passed to the function is a perfect number. Use this function in a C program that determines and prints all the perfect numbers between 1 and 1000. Print the factors of each perfect number to confirm that the number is indeed perfect.
// isPerfect returns true if value is perfect integer,
// i.e., if value is equal to sum of its factors
int isPerfect(int value)
{
int factorSum = 1; // current sum of factors
// loop through possible factor values
for (int i = 2; i <= value / 2; ++i) {
// if i is factor
if (value % i == 0) {
factorSum += i; // add to sum
}
}
// return true if value is equal to sum of factors
if (factorSum == value) {
return 1;
}
else {
return 0;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

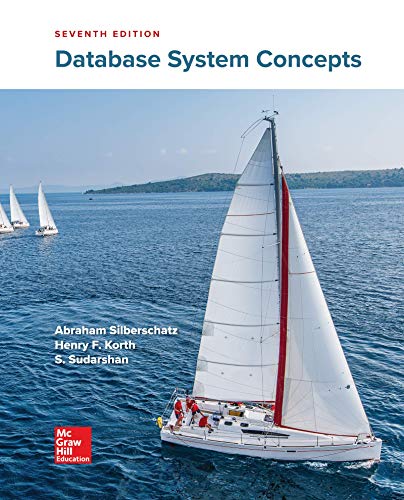
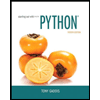
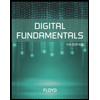
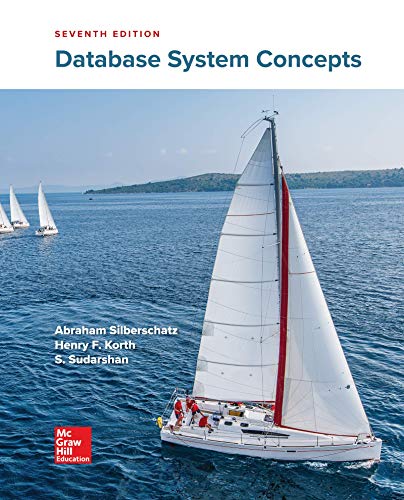
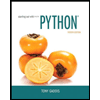
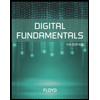
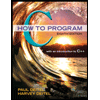
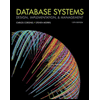
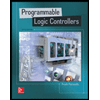