c program add a report to count the total number of beaches in the file and how many are open, and how many are closed. //include the required header file. #include "stdio.h" //Define the main function. int main(void) { //Declare the variable. int b_num, num_samples, num_orgs_per_100; char c = 'a'; //Create a file pointer. FILE *in_file; //Open the file in read mode. in_file = fopen("inp.txt", "r"); //Check if the file exists. if (in_file == NULL) printf("Error opening the file.\n"); //Read the data from the file. else { //Get the beach number and number of //samples from the file. fscanf(in_file, "%d", &b_num); fscanf(in_file, "%d", &num_samples); //Check for end of file and beach //number value and dispaly the samples. while(!feof(in_file)) { printf("\nb_num = %d, num_samples = %d", b_num,num_samples); for(int i =0;i< num_samples;i++) { fscanf(in_file, "%d", &num_orgs_per_100); //Display the input number. printf("\nSample value %d: %d",i+1, num_orgs_per_100); } //Read the newline, number of samples and b_num for //next record fscanf(in_file, "%c", &c); printf("\n"); fscanf(in_file, "%d", &b_num); fscanf(in_file, "%d", &num_samples); }} //Close the file. fclose(in_file); return 0; }
c program
- add a report to count the total number of beaches in the file and how many are open, and how many are closed.
//include the required header file.
#include "stdio.h"
//Define the main function.
int main(void)
{
//Declare the variable.
int b_num, num_samples, num_orgs_per_100;
char c = 'a';
//Create a file pointer.
FILE *in_file;
//Open the file in read mode.
in_file = fopen("inp.txt", "r");
//Check if the file exists.
if (in_file == NULL)
printf("Error opening the file.\n");
//Read the data from the file.
else
{
//Get the beach number and number of
//samples from the file.
fscanf(in_file, "%d", &b_num);
fscanf(in_file, "%d", &num_samples);
//Check for end of file and beach
//number value and dispaly the samples.
while(!feof(in_file))
{
printf("\nb_num = %d, num_samples = %d",
b_num,num_samples);
for(int i =0;i< num_samples;i++)
{
fscanf(in_file, "%d", &num_orgs_per_100);
//Display the input number.
printf("\nSample value %d: %d",i+1,
num_orgs_per_100);
}
//Read the newline, number of samples and b_num for
//next record
fscanf(in_file, "%c", &c);
printf("\n");
fscanf(in_file, "%d", &b_num);
fscanf(in_file, "%d", &num_samples);
}}
//Close the file.
fclose(in_file);
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

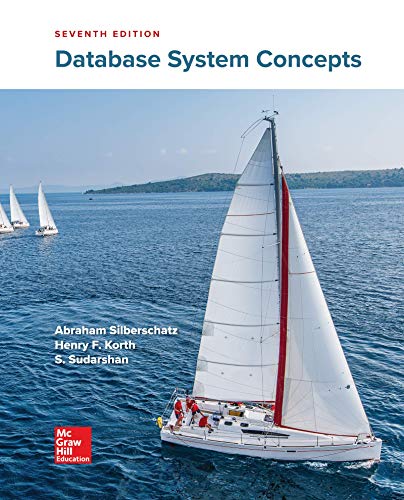
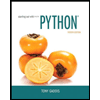
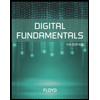
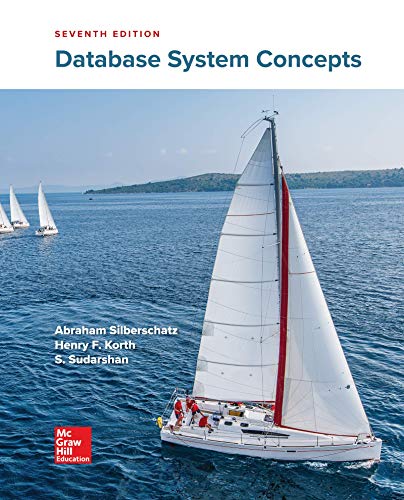
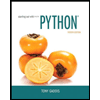
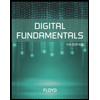
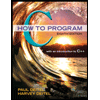
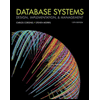
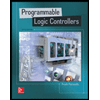