C++ Please make the necessary changes and finish the code. Upvote for quick working code in Visual Studio. Thank You. Objectives • To reinforce previously learned fundamentals • To further apply OOP principles • To understand inheritance better Instructions Major steps outline: 1. Implement a BasicShape class, which will be a pure abstract base class 2. Implement a Circle class derived from the BasicShape class 3. Implement a Recentage class derived from the BasicShape class 4. Dynamically create objects of the derived classes (at least 6 objects), and store them in a vector 5. Iterate through the vector, and call the whatAmI, as well as the getArea method (described later) to ensure the correct areas are calculate for the given shape Implement a BasicShape class Write one file: • BasicShape.h The class is a pure abstract base class, which means all of its methods are pure virtual functions. If you don’t know what this means, please refer to the lecture video as well as the Chapter 11 and 15 material. The pure virtual methods in the BasicShape class are: • whatAmI • getArea The whatAmI function will be implemented by derived classes and return the name of its type, i.e., “Circle” or “Rectangle” as a string. The getArea function returns a double, which will return the correct area based on the type of shape (e.g., Circle, Rectangle) on which it is called. Implement Circle and Rectangle classes Both classes must inherit from the BasicShape class using public inheritance. Both classes must also implement, as a result, the pure virtual methods of the BasicShape class: • whatAmI • getArea Both classes must implement getters and setters for all private data members. Both classes must implement Constructors that take the required data (radius for Circle, length and width for Rectangle) as parameters The Circle class should contain a double radius for its private data, provide the following methods: • getCircumference – calculate and return the circumference of the circle • getArea (overridden implementation of the inherited BasicShape class) • whatAmI (also overridden; returns the string “Circle”) The Rectangle class should contain two double variables - length and width, as its private data. It should provide implementations of the following methods: • getPerimeter • getArea (overridden implementation of the inherited BasicShape class) • whatAmI (also overridden; returns the string “Rectangle”) The main File In the main file, you should dynamically create the instances of the Rectangles and Circles, storing pointers to the objects into the vector. When you test it out in main, loop through the vector, and print out the type of class (using the whatAmI) as well as the area, using getArea. You should get the correct values, based on the type of object at each position within the area. Also, when done, do not forget to delete the objects as well as clear the vector.
C++
Please make the necessary changes and finish the code.
Upvote for quick working code in Visual Studio. Thank You.
Objectives
• To reinforce previously learned fundamentals
• To further apply OOP principles
• To understand inheritance better
Instructions
Major steps outline:
1. Implement a BasicShape class, which will be a pure abstract base class
2. Implement a Circle class derived from the BasicShape class
3. Implement a Recentage class derived from the BasicShape class
4. Dynamically create objects of the derived classes (at least 6 objects), and store them in a
5. Iterate through the vector, and call the whatAmI, as well as the getArea method (described later) to ensure the correct areas are calculate for the given shape
Implement a BasicShape class
Write one file:
• BasicShape.h
The class is a pure abstract base class, which means all of its methods are pure virtual functions. If you don’t know what this means, please refer to the lecture video as well as the Chapter 11 and 15 material.
The pure virtual methods in the BasicShape class are:
• whatAmI
• getArea
The whatAmI function will be implemented by derived classes and return the name of its type, i.e., “Circle” or “Rectangle” as a string.
The getArea function returns a double, which will return the correct area based on the type of shape (e.g., Circle, Rectangle) on which it is called.
Implement Circle and Rectangle classes
Both classes must inherit from the BasicShape class using public inheritance.
Both classes must also implement, as a result, the pure virtual methods of the BasicShape class:
• whatAmI
• getArea
Both classes must implement getters and setters for all private data members.
Both classes must implement Constructors that take the required data (radius for Circle, length and width for Rectangle) as parameters
The Circle class should contain a double radius for its private data, provide the following methods:
• getCircumference – calculate and return the circumference of the circle
• getArea (overridden implementation of the inherited BasicShape class)
• whatAmI (also overridden; returns the string “Circle”)
The Rectangle class should contain two double variables - length and width, as its private data. It should provide implementations of the following methods:
• getPerimeter
• getArea (overridden implementation of the inherited BasicShape class)
• whatAmI (also overridden; returns the string “Rectangle”)
The main File
In the main file, you should dynamically create the instances of the Rectangles and Circles, storing pointers to the objects into the vector<BasicShape*>. When you test it out in main, loop through the vector, and print out the type of class (using the whatAmI) as well as the area, using getArea. You should get the correct values, based on the type of object at each position within the area.
Also, when done, do not forget to delete the objects as well as clear the vector.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

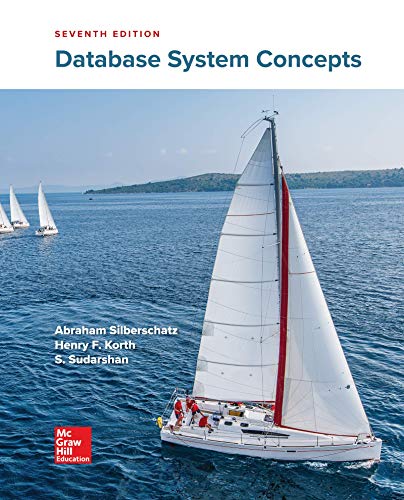
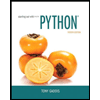
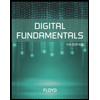
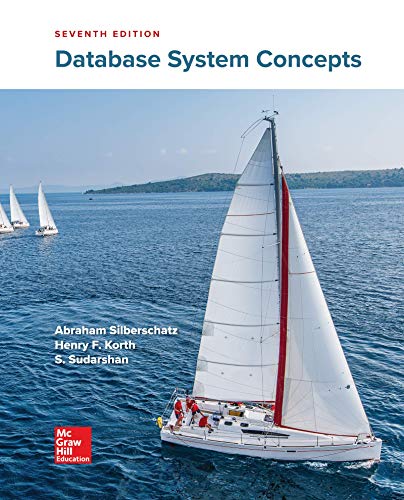
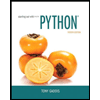
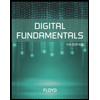
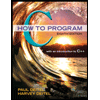
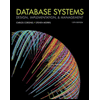
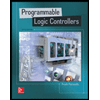