c++ Need help with part 4 so far i have this: #include #include using namespace std; void printMenu() { cout << "\nMENU" << endl; cout << "c - Number of non-whitespace characters" << endl; cout << "w - Number of words" << endl; cout << "f - Find text" << endl; cout << "r - Replace all !'s" << endl; cout << "s - Shorten spaces" << endl; cout << "q - Quit" << endl; } string ExecuteMenu(string text, char choice) { int i, count; string temp; int found; int lenT = text.length(), lenTemp = 0, j; char t; switch (choice) { case 'q':exit(0); break; case 'c': count = 0; for (i = 0; i < text.length(); ++i) { if (text[i] != ' ') count++; } cout << "\nNumber of non-whitespace characters : " << count; break; case 'w': count = 1; for (i = 0; i < text.length(); ++i) { if (text[i] == ' ') count++; } cout << "\n Number of words : " << count; break; case 'f': cout << "Enter the string to find : "; cin >> temp; lenTemp = temp.length(); found = text.find(temp); if (found != string::npos) cout << "Found Text At : " << found << endl; break; case 'r': cout << "Enter the character to replace \"!\" : "; cin >> t; for (i = 0; i < text.length(); ++i) { if (text[i] == '!') text[i] = t; } cout << "Given string after replace : " << text; break; case 's': for (i = 0; i < text.length() - 2; ++i) { while (text[i] == ' ' && text[i + 1] == ' ') { for (j = i + 1; j < text.length(); j++) text[j] = text[j + 1]; text[j] = '\0'; } } cout << "The given text after shorten spaces : " << text; } return text; } int main() { string text; cout << "Enter a sample text:\n" << endl; getline(cin, text); cout << "You entered: "; cout << text << endl; int choice; string t; while (true) { printMenu(); cout << "\nChoose an option:" << endl; cin >> t; choice = t[0]; if (choice != 'c' && choice != 'w' && choice != 'f' && choice != 'r' && choice != 's' && choice != 'q') { cout << "Invalid Choice\nPlease enter Correct Option" << endl; } else text = ExecuteMenu(text, choice); } return 0; }
c++ Need help with part 4
so far i have this:
#include <iostream>
#include <string>
using namespace std;
void printMenu() {
cout << "\nMENU" << endl;
cout << "c - Number of non-whitespace characters" << endl;
cout << "w - Number of words" << endl;
cout << "f - Find text" << endl;
cout << "r - Replace all !'s" << endl;
cout << "s - Shorten spaces" << endl;
cout << "q - Quit" << endl;
}
string ExecuteMenu(string text, char choice) {
int i, count;
string temp;
int found;
int lenT = text.length(), lenTemp = 0, j;
char t;
switch (choice) {
case 'q':exit(0); break;
case 'c':
count = 0;
for (i = 0; i < text.length(); ++i)
{
if (text[i] != ' ')
count++;
}
cout << "\nNumber of non-whitespace characters : " << count; break;
case 'w':
count = 1;
for (i = 0; i < text.length(); ++i)
{
if (text[i] == ' ')
count++;
}
cout << "\n Number of words : " << count; break;
case 'f':
cout << "Enter the string to find : ";
cin >> temp;
lenTemp = temp.length();
found = text.find(temp);
if (found != string::npos)
cout << "Found Text At : " << found << endl; break;
case 'r':
cout << "Enter the character to replace \"!\" : ";
cin >> t;
for (i = 0; i < text.length(); ++i)
{
if (text[i] == '!')
text[i] = t;
}
cout << "Given string after replace : " << text; break;
case 's':
for (i = 0; i < text.length() - 2; ++i)
{
while (text[i] == ' ' && text[i + 1] == ' ')
{
for (j = i + 1; j < text.length(); j++)
text[j] = text[j + 1];
text[j] = '\0';
}
}
cout << "The given text after shorten spaces : " << text;
}
return text;
}
int main() {
string text;
cout << "Enter a sample text:\n" << endl;
getline(cin, text);
cout << "You entered: ";
cout << text << endl;
int choice;
string t;
while (true) {
printMenu();
cout << "\nChoose an option:" << endl;
cin >> t;
choice = t[0];
if (choice != 'c' && choice != 'w' && choice != 'f' && choice != 'r' && choice != 's' && choice != 'q')
{
cout << "Invalid Choice\nPlease enter Correct Option" << endl;
}
else
text = ExecuteMenu(text, choice);
}
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

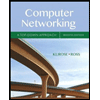
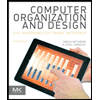
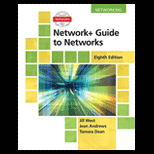
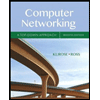
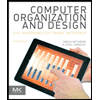
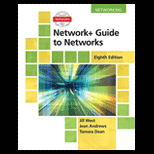
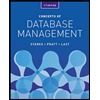
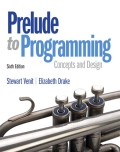
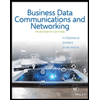