C++ Instructions Write the definition of the function moveNthFront that takes as a parameter a positive integer, n. The function moves the nth element of the queue to the front. The order of the remaining elements remains unchanged.
C++ Instructions Write the definition of the function moveNthFront that takes as a parameter a positive integer, n. The function moves the nth element of the queue to the front. The order of the remaining elements remains unchanged.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
17-14 C++
Instructions Write the definition of the function moveNthFront that takes as a parameter a positive integer, n. The function moves the nth element of the queue to the front. The order of the remaining elements remains unchanged. For example, suppose: queue = {5, 11, 34, 67, 43, 55} and n = 3. After a call to the function moveNthFront: queue = {34, 5, 11, 67, 43, 55} Add this function to the class queueType. Also, write a

Transcribed Image Text:Instructions
Instructions
a. Add the following operation to the class
linkedStackType.
void reverseStack (linkedStackType<Type> &otherSt
This operation copies the elements of a stack in reverse
order onto another stack.
Consider the following statements:
linkedStackType<int> stack1;
linkedStackType<int> stack2;
The statement
stack1.reverseStack (stack2);
copies the elements of stack1 onto stack2 in reverse
order. That is, the top element of stack1 is the bottom
element of stack2, and so on. The old contents of
stack2 are destroyed, and stack1 is unchanged.
b. Write the definition of the function template to
implement the operation reverseStack.
Write a program to test the class linkedStackType.
Grading
When you have completed your program, click the Submit
button to record your score.
main.cpp
1 //Header File: linkedStack.h
2
3 #ifndef H_StackType
4 #define H_StackType
5
6 #include <iostream>
7 #include <cassert>
8
9 #include "stackADT.h"
10
11 using namespace std;
12
linkedStack.h
13 //Definition of the node
14 template <class Type>
15 struct node Type
16 {
17
18
19 };
20
Type info;
nodeType<Type> *link;
21 template <class Type>
22 class linkedStackType: public stackADT<Type>
23 {
24 public:
25
26
27
28
29
30
31
const linkedStackType<Type>& operator=
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76 private:
77
78
79
80
81
82
83 };
84
//Overload the assignment operator.
//
bool isEmptyStack() const;
//Function to determine whether the stack is empty.
//Postcondition: Returns true if the stack is empty;
otherwise returns false.
191
192
193
194
195
bool isFullStack() const;
//Function to determine whether the stack is full.
//Postcondition: Returns false.
//
void initializeStack();
//Function to initialize the stack to an empty state.
//Postcondition: The stack elements are removed;
stackTop = nullptr;
//
void push(const Type& newItem);
//Function to add newItem to the stack.
//Precondition: The stack exists and is not full.
//Postcondition: The stack is changed and newItem
is added to the top of the stack.
//
//
Type top() const;
//Function to return the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: If the stack is empty, the program
terminates; otherwise, the top
element of the stack is returned.
//
void pop();
//Function to remove the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: The stack is changed and the top
element is removed from the stack.
linkedStackType();
//Default constructor
//Postcondition: stackTop = nullptr;
linkedStackType(const linkedStackType<Type>& otherStack);
//Copy constructor
//
{
-linkedStackType();
//Destructor
//Postcondition: All the elements of the stack are
removed from the stack.
void reverseStack (linkedStackType<Type> &otherStack);
nodeType<Type> *stackTop; //pointer to the stack
void copyStack (const linkedStackType<Type>& otherStack);
//Function to make a copy of otherStack.
//
//Default constructor
//Postcondition: A copy of otherStack is created and
assigned to this stack.
85
86 template <class Type>
87 linkedStackType<Type> ::linkedStackType()
88 {
89
90 }
91
92 template <class Type>
93 bool linkedStackType<Type>::isEmptyStack() const
94 {
95 return (stack Top == nullptr);
96}//end isEmptyStack
stackTop = nullptr;
stackADT.h
(const linkedStackType<Type>&);
97
98 template <class Type>
99 bool linkedStackType<Type> :: is FullStack() const
100 {
101
return false;
102 //end isFullStack
103
104 template <class Type>
105 void linkedStackType<Type>::initializeStack()
106 {
107 node Type<Type> *temp; //pointer to delete the node
108
109 while (stack Top != nullptr) //while there are elements in
110
//the stack
111
112
113
114
115
116
117
}
118} //end initializeStack
119
120 template <class Type>
121 void linkedStackType<Type>::push(const Type& newElement)
122 {
123
124
125
126
127
128
129 stackTop newNode;
130
131} //end push
132
133
temp stackTop;
//set temp to point to the
//current node
stackTop stackTop->link; //advance stackTop to the
//next node
delete temp; //deallocate memory occupied by temp
nodeType<Type> *newNode; //pointer to create the new node
134 template <class Type>
135 Type linkedStackType<Type> :: top() const
136 {
137
138
newNode = new nodeType<Type>; //create the node
newNode->info = newElement; //store newElement in the node
newNode->link= stackTop; //insert newNode before stackTop
//set stackTop to point to the
//top node
139
140 }//end top
141
return stackTop->info;
assert (stackTop != nullptr); //if stack is empty,
//terminate the program
//return the top element
142 template <class Type>
143 void linkedStackType<Type> : :pop()
144 {
else
if (stackTop != nullptr)
{
+
145 node Type<Type> *temp; //pointer to deallocate memory
146
147
148
149
150
151
152
153
154 }
155
156
157 }//end pop
158
else
{
159 template <class Type>
160 void linkedStackType<Type>::copyStack
161
162 {
163 nodeType<Type> *newNode, *current, *last;
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
temp = stackTop; //set temp to point to the top node
stackTop = stackTop->link; //advance stackTop to the
//next node
delete temp; //delete the top node.
cout << "Cannot remove from an empty stack." << endl;
if (otherStack.stackTop ==
stack Top = nullptr;
if (stackTop != nullptr) //if stack is nonempty, make it empty
initializeStack();
(const linkedStackType<Type>& otherStack)
current = otherStack.stackTop; //set current to point
//to the stack to be copied
//copy the stackTop element of the stack
stack Top = new nodeType<Type>; //create the node
last stackTop;
current current->link;
nullptr)
stackTop->info = current->info; //copy the info
stackTop->link = nullptr; //set the link field of the
}//end while
//copy the remaining stack
while (current != nullptr)
{
}//end else
196}//end copyStack
newNode= new nodeType<Type> ;
newNode->info = current->info;
newNode->link = nullptr;
last->link = newNode;
last newNode;
current = current->link;
//node to nullptr
//set last to point to the node
//set current to point to
//the next node
stack Top = nullptr;
copyStack (otherStack);
205 }//end copy constructor
206
197
198 //copy constructor
199 template <class Type>
200 linkedStack Type<Type> ::linkedStackType(
201
202 {
203
204
const linkedStackType<Type>& otherStack)
207 //destructor
208 template <class Type>
209 linkedStackType<Type>::-linkedStackType()
210 {
211 initializeStack();
212 }//end destructor
213
214 //overloading the assignment operator
215 template <class Type>
216 const linkedStackType<Type>& linkedStackType<Type>::operator=
217
(const linkedStack Type<Type>& otherStack)
218 {
219 if (this ! &otherStack) //avoid self-copy
220
copyStack (otherStack);
221
222
return *this;
223 }//end operator=
224
225 #endif
226
linkedStack.h
main.cpp
1 #include <iostream>
2 #include "linkedStack.h"
3
4 using namespace std;
5
6 int main() {
7
8
9}
// Write your main here
return 0;
stackADT.h
+
linkedStack.h
main.cpp
1 //Header file: stackADT.h
2
3 #ifndef H_StackADT
4 #define H_StackADT
5
6 template <class Type>
7 class stackADT
8 {
9 public:
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
virtual void initializeStack() = 0;
//Method to initialize the stack to an empty state.
//Postcondition: Stack is empty
//
virtual bool isEmptyStack() const = 0;
//Function to determine whether the stack is empty.
//Postcondition: Returns true if the stack is empty,
otherwise returns false.
//
virtual bool isFullStack() const = 0;
//Function to determine whether the stack is full.
//Postcondition: Returns true if the stack is full,
otherwise returns false.
stackADT.h
//
+
virtual void push(const Type& newItem) = 0;
//Function to add newItem to the stack.
//Precondition: The stack exists and is not full.
//Postcondition: The stack is changed and newItem
is added to the top of the stack.
//
//
virtual Type top() const = 0;
//Function to return the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: If the stack is empty, the program
terminates; otherwise, the top element
of the stack is returned.
39
40
41
42 };
43
44 #endif
45
virtual void pop() = 0;
//Function to remove the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: The stack is changed and the top
element is removed from the stack.
//
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Recommended textbooks for you
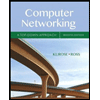
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
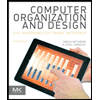
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
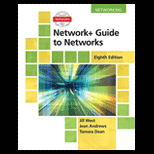
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
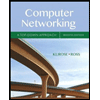
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
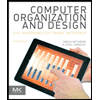
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
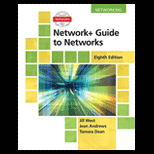
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
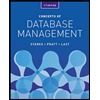
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
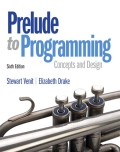
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
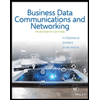
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY