C++ In this project you will implement two small programs. One program will show an example of stack use and another program will show the use of a Queue 1. Use the STL to create your data structures 2. You should have the following features: a. Add a record b. Find a record

#include <iostream>
#include <bits/stdc++.h>
using namespace std;
//Function to print the Maximum element in the stack
void findstack(stack<int> s)
{
int m = s.top(); //initialization
int a;
int n;
cout<<"Enter value to find:";
cin >> a;
while(!s.empty())
{
if (m==a)
{n=1;
}
s.pop();
}
if(n==1)
{
cout << "Element is found";
}
else
{
cout<<"Not found";
}
}
//Function to print the elements of the stack
void show(stack<int> s)
{
while (!s.empty())
{
cout << " " << s.top(); //printing the topmost element
s.pop(); //removing the topmost element to bring next element at the top
}
cout << endl;
}
int main()
{
int i;
//Stack declaration (stack of integers)
stack<int> s;
//Filling the elements by using the push() method.
cout << "Filling the Stack in LIFO order using the push() method:"; //LIFO= Last In First Out
s.push(4);
s.push(2);
s.push(20);
s.push(12);
s.push(52);
s.push(14);
cout << "\n\nThe elements of the Stack in LIFO order are: ";
show(s);
findstack(s); //to find the stack element
cout << "\n\n\n";
return 0;
}
Step by step
Solved in 2 steps

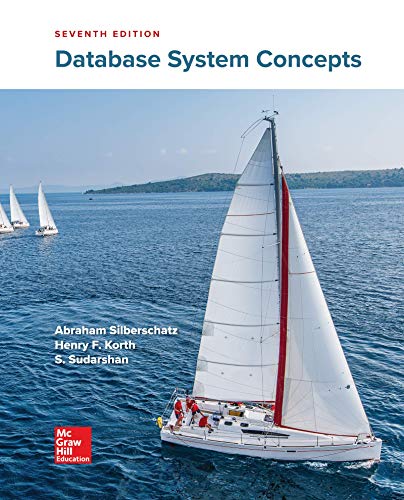
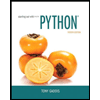
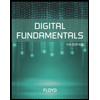
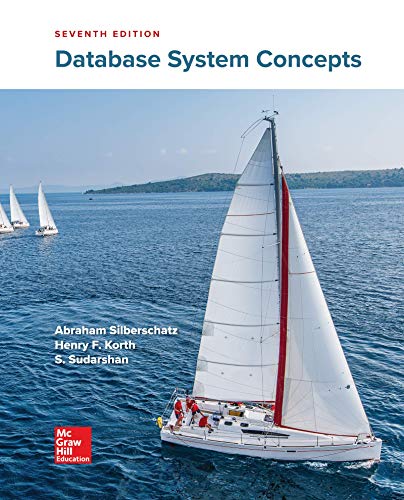
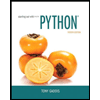
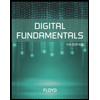
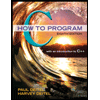
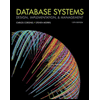
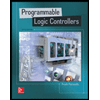