C++ Do not use str:: First, read in an input value for variable numVals. Then, read numVals integers from input and output each on the same line with the character " _ " between each value. End with a newline. Note: " _ " should not be at the beginning or end of the output. Ex: If the input is 3 50 70 10, the output is: 50 _ 70 _ 10 #include using namespace std; int main() { int numVals; cin >> numVals; int inputNum; for (int i = 0; i < numVals; i++) { cin >> inputNum; cout << inputNum << " _"; } if (int i != numVals - 1) { cout << inputNum <<" _"; } cout << endl; return 0; } main.cpp: In function ‘int main()’: main.cpp:15:17: error: expected initializer before ‘!=’ token 15 | if (int i != numVals - 1) { | ^~ main.cpp:15:16: error: expected ‘)’ before ‘!=’ token 15 | if (int i != numVals - 1) { | ~ ^~~ | )
C++
Do not use str::
First, read in an input value for variable numVals. Then, read numVals integers from input and output each on the same line with the character " _ " between each value. End with a newline.
Note: " _ " should not be at the beginning or end of the output.
Ex: If the input is 3 50 70 10, the output is:
50 _ 70 _ 10
#include <iostream>
using namespace std;
int main() {
int numVals;
cin >> numVals;
int inputNum;
for (int i = 0; i < numVals; i++) {
cin >> inputNum;
cout << inputNum << " _";
}
if (int i != numVals - 1) {
cout << inputNum <<" _";
}
cout << endl;
return 0;
}

Algorithm of the code:
1. Declare an integer variable numVals
2. Prompt the user for an input numVals
3. Set a for loop to iterate over numVals times
4. Inside the for loop, prompt the user for an input number
5. Print the input number
6. If the current iteration of the loop is not the last iteration, print an underscore
7. End the for loop
8. End the program
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

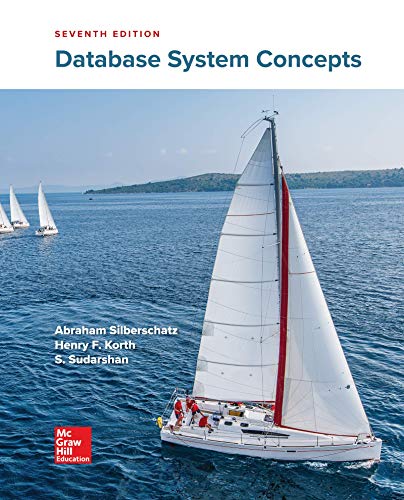
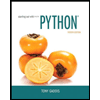
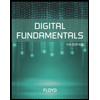
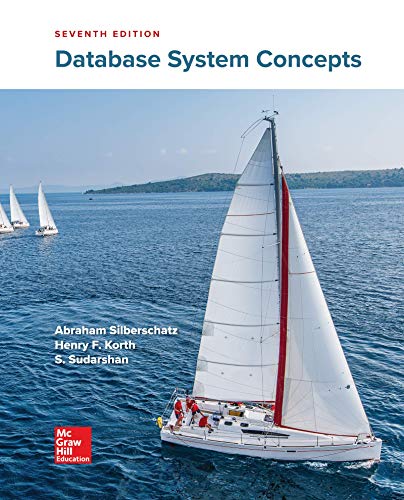
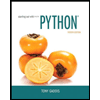
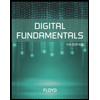
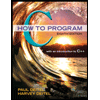
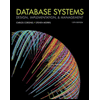
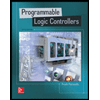