C Code. Dining Philosopher’s problem is a famous problem in OS. A deadlock may happen when all philosophers want to start eating at the same time and pick up one chopstick and wait for the other chopstick. We can use semaphores to simulate the availability of chopsticks. To prevent the deadlock: a) Use an asymmetric solution: an odd-numbered philosopher picks up first the left chopstick and then the right chopstick. Even-numbered philosopher picks up first the right chopstick and then the left chopstick. The following program can lead to a deadlock. Based on the program, please implement the above solution to prevent the deadlock.
C Code. Dining Philosopher’s problem is a famous problem in OS. A deadlock may happen when all philosophers want to start eating at the same time and pick up one chopstick and wait for the other chopstick. We can use semaphores to simulate the availability of chopsticks. To prevent the deadlock: a) Use an asymmetric solution: an odd-numbered philosopher picks up first the left chopstick and then the right chopstick. Even-numbered philosopher picks up first the right chopstick and then the left chopstick. The following program can lead to a deadlock. Based on the program, please implement the above solution to prevent the deadlock.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
C Code. Dining Philosopher’s problem is a famous problem in OS. A deadlock may happen when all philosophers want to start eating at the same time and pick up one chopstick and wait for the other chopstick. We can use semaphores to simulate the availability of chopsticks.
To prevent the deadlock:
a) Use an asymmetric solution: an odd-numbered philosopher picks up first the left chopstick and then the right chopstick. Even-numbered philosopher picks up first the right chopstick and then the left chopstick.
The following program can lead to a deadlock. Based on the program, please implement the above solution to prevent the deadlock.
![#include<stdio.h>
#include<semaphore.h>
#include<pthread.h>
#define N 5 //the number of philosophers
sem_t S[N]; //semaphores for chopsticks
void *
void take_chopsticks (int);
void put_chopsticks (int);
philospher (void *num);
int phil_num [N]= {0,1,2,3, 4}; //philosopher ID
int main ()
int i;
pthread_t thread_id[N];
for (i=0;i<N;i++)
sem_init (&S[i],0,1);
for (i=0;i<N;i++)
pthread_create (&thread_id[i],NULL,philospher, &phil_num[i]);
for (i=0;i<N;i++)
pthread_join (thread_id[i] , NULL);
void *philospher (void *num)
{
while (1)
{
int *i = num;
take_chopsticks (*i);
put_chopsticks (*i);
void take_chopsticks (int ph_num)
{
printf("Philosopher %d is Hungry\n",ph_num);
sem_wait (&S[ph_num]);
printf ("Philosopher %d takes chopstick %d \n",ph_num, ph_num);
//take the left chopstick
sleep (1);
sem_wait (&S[ (ph_num+1) %N]);
printf ("Philosopher %d takes chopstick %d \n",ph_num, (ph_num+1) %N);
//take the right chopstick
printf ("Philosopher %d is eating\n", ph_num);
sleep (1);
void put_chopsticks (int ph_num)
{
sem_post (&S[ph_num]); //put the left chopstick
printf("Philosopher %d putting chopstick %d \n",ph_num, ph_num);
sleep (1);
sem_post (&S [ (ph_num+1)%N]); //put the right chopstick
printf("Philosopher %d putting chopstick %d \n",ph_num, (ph_num+1)%N);
printf("Philosopher %d is thinking\n",ph_num);
sleep (1);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F75dd0177-aa2e-4a60-8fd3-85ac4f4eaf24%2Fb4bc7b49-8ad2-4d64-a6ac-fc20608ed69d%2Ft44qr2d_processed.jpeg&w=3840&q=75)
Transcribed Image Text:#include<stdio.h>
#include<semaphore.h>
#include<pthread.h>
#define N 5 //the number of philosophers
sem_t S[N]; //semaphores for chopsticks
void *
void take_chopsticks (int);
void put_chopsticks (int);
philospher (void *num);
int phil_num [N]= {0,1,2,3, 4}; //philosopher ID
int main ()
int i;
pthread_t thread_id[N];
for (i=0;i<N;i++)
sem_init (&S[i],0,1);
for (i=0;i<N;i++)
pthread_create (&thread_id[i],NULL,philospher, &phil_num[i]);
for (i=0;i<N;i++)
pthread_join (thread_id[i] , NULL);
void *philospher (void *num)
{
while (1)
{
int *i = num;
take_chopsticks (*i);
put_chopsticks (*i);
void take_chopsticks (int ph_num)
{
printf("Philosopher %d is Hungry\n",ph_num);
sem_wait (&S[ph_num]);
printf ("Philosopher %d takes chopstick %d \n",ph_num, ph_num);
//take the left chopstick
sleep (1);
sem_wait (&S[ (ph_num+1) %N]);
printf ("Philosopher %d takes chopstick %d \n",ph_num, (ph_num+1) %N);
//take the right chopstick
printf ("Philosopher %d is eating\n", ph_num);
sleep (1);
void put_chopsticks (int ph_num)
{
sem_post (&S[ph_num]); //put the left chopstick
printf("Philosopher %d putting chopstick %d \n",ph_num, ph_num);
sleep (1);
sem_post (&S [ (ph_num+1)%N]); //put the right chopstick
printf("Philosopher %d putting chopstick %d \n",ph_num, (ph_num+1)%N);
printf("Philosopher %d is thinking\n",ph_num);
sleep (1);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
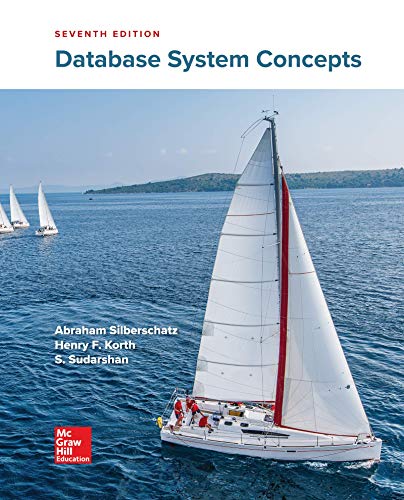
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
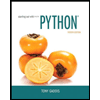
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
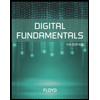
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
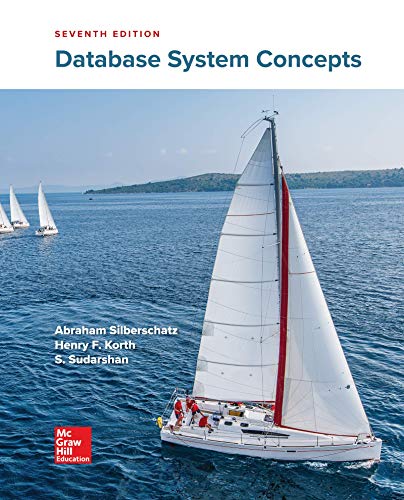
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
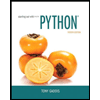
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
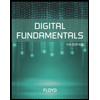
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
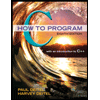
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
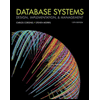
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
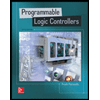
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education