Build a class called BankAccount that manages checking and savings accounts. The class has three private member fields: a custome name (String), the customer's savings account balance (double), and the customer's checking account balance (double).
Build a class called BankAccount that manages checking and savings accounts. The class has three private member fields: a custome name (String), the customer's savings account balance (double), and the customer's checking account balance (double).
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
JAVA
![1 public class BankAccount {
IN45N89
2
3/***
6
7
10
11
12
13
14
15
16
17 }
555588
BankAccount.java
********NEW JAVA CODE HERE
public static void main(String args[]) {
BankAccount account = new BankAccount ("Mickey", 500.00, 1000.00);
account.setChecking (500);
account.setSavings (500);
account.withdrawSavings (100);
account.withdrawChecking(100);
account.transfer ToSavings (300);
System.out.println(account.getName());
System.out.printf("$ %.2f\n", account.getChecking());
System.out.printf("$ %.2f\n", account.getSavings());
Load default template...
*******/](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0cc153ae-b205-4fb2-9991-7cf6a21d5016%2Fe2104b1f-ec29-4aa6-9270-ff07df4a1ae6%2Fau39027_processed.png&w=3840&q=75)
Transcribed Image Text:1 public class BankAccount {
IN45N89
2
3/***
6
7
10
11
12
13
14
15
16
17 }
555588
BankAccount.java
********NEW JAVA CODE HERE
public static void main(String args[]) {
BankAccount account = new BankAccount ("Mickey", 500.00, 1000.00);
account.setChecking (500);
account.setSavings (500);
account.withdrawSavings (100);
account.withdrawChecking(100);
account.transfer ToSavings (300);
System.out.println(account.getName());
System.out.printf("$ %.2f\n", account.getChecking());
System.out.printf("$ %.2f\n", account.getSavings());
Load default template...
*******/

Transcribed Image Text:Build a class called BankAccount that manages checking and savings accounts. The class has three private member fields: a customer
name (String), the customer's savings account balance (double), and the customer's checking account balance (double).
Implement the following Constructor and instance methods:
• public BankAccount(String newName, double amt1, double amt2) - set the customer name to parameter newName, set the checking
account balance to parameter amt1 and set the savings account balance to parameter amt2. (amt stands for amount)
• public void setName(String new Name) - set the customer name
• public String getName() - return the customer name
public void setChecking(double amt) - set the checking account balance to parameter amt
• public double getChecking() - return the checking account balance
• public void setSavings (double amt) - set the savings account balance to parameter amt
public double getSavings() - return the savings account balance
public void depositChecking(double amt) - add parameter amt to the checking account balance (only if positive)
public void depositSavings(double amt) - add parameter amt to the savings account balance (only if positive)
• public void withdrawChecking (double amt) - subtract parameter amt from the checking account balance (only if positive)
• public void withdrawSavings(double amt) - subtract parameter amt from the savings account balance (only if positive)
public void transfer ToSavings(double amt) - subtract parameter amt from the checking account balance and add to the savings
account balance (only if positive)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
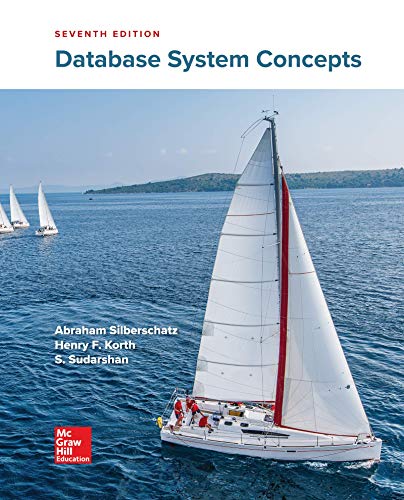
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
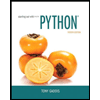
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
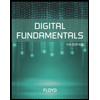
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
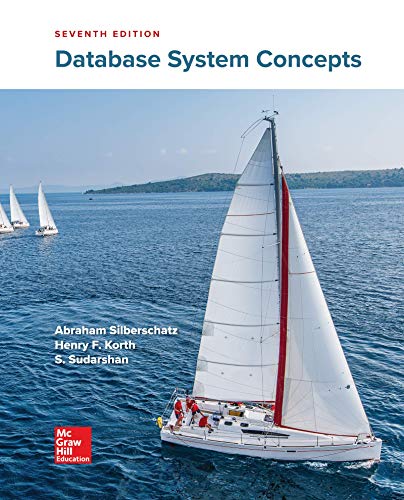
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
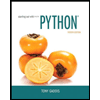
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
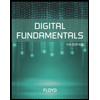
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
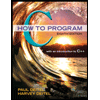
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
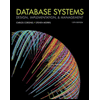
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
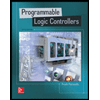
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education