(Bubble Sort) The bubble sort presented in Fig. 6.15 is inefficient for large arrays. Make the 6.11 following simple modifications to improve its performance. a) After the first pass, the largest number is guaranteed to be in the highest-numbered el- ement of the array; after the second pass, the two highest numbers are "in place," and so on. Instead of making nine comparisons on every pass, modify the bubble sort to make eight comparisons on the second pass, seven on the third b) The data in the array may already be in the proper or near-proper order, so why make nine passes if fewer will suffice? Modify the sort to check at the end of each any swaps have been made. If none has been made, then the data must already be in the proper order, so the program should terminate. If swaps have been made, then at least CC • and So on. pass whether pass one more pass is needed. adimarninnalonoronorotione 11 6.8 Sorting Arrays increasing order (or if the values are identical), v decreasing order, their values are swapped in the array. 235 // Fig. 6.15: fig06_15.c // Sorting an array's values into ascending order. #include #define SIZE 10 // function main begins program execution int main(void) // initialize a int a[SIZE] = {2, 6, 4, 8, 10, 12, 89, 68, 45, 37}; 10 %3D puts ("Data items in original order"); 12 13 // output original array for (size_ti = 0; i < SIZE; ++i) { printf("%4d", a[i]); } 14 15 16 17 18 // bubble sort //loop to control number of passes for (unsigned int pass 19 20 gmis 21 1; pass < SIZE; ++pass) { 22 // loop to control number of comparisons per pass for (size_t i = 0; i < SIZE 23 24 1; ++i) { 25 // compare adjacent elements and swap them if first // element is greater than second element if (a[i] > a[i + 1]) { int hold = a[i]; a[i] = a[i + 1]; a[i + 1] = hold; 26 27 28 29 30 2.0 Agnial) 31 32 33 } 34 za roy algms 35 aluesi puts("\nData items in ascending order"); 36 37 // output sorted array for (size_t i = 0; i < SIZE; ++i) { printf("%4d", a[i]); ibarm.nssm ad 38 39 40 41 42 puts(""); 43 44 } Data items in original order 2 6 4 8 10 12 89 68 45 37 Data items in ascending order 2 4 6 8 10 12 37 45 68 89 Fig. 6.15 | Sorting an array's values into ascending order.
(Bubble Sort) The bubble sort presented in Fig. 6.15 is inefficient for large arrays. Make the 6.11 following simple modifications to improve its performance. a) After the first pass, the largest number is guaranteed to be in the highest-numbered el- ement of the array; after the second pass, the two highest numbers are "in place," and so on. Instead of making nine comparisons on every pass, modify the bubble sort to make eight comparisons on the second pass, seven on the third b) The data in the array may already be in the proper or near-proper order, so why make nine passes if fewer will suffice? Modify the sort to check at the end of each any swaps have been made. If none has been made, then the data must already be in the proper order, so the program should terminate. If swaps have been made, then at least CC • and So on. pass whether pass one more pass is needed. adimarninnalonoronorotione 11 6.8 Sorting Arrays increasing order (or if the values are identical), v decreasing order, their values are swapped in the array. 235 // Fig. 6.15: fig06_15.c // Sorting an array's values into ascending order. #include #define SIZE 10 // function main begins program execution int main(void) // initialize a int a[SIZE] = {2, 6, 4, 8, 10, 12, 89, 68, 45, 37}; 10 %3D puts ("Data items in original order"); 12 13 // output original array for (size_ti = 0; i < SIZE; ++i) { printf("%4d", a[i]); } 14 15 16 17 18 // bubble sort //loop to control number of passes for (unsigned int pass 19 20 gmis 21 1; pass < SIZE; ++pass) { 22 // loop to control number of comparisons per pass for (size_t i = 0; i < SIZE 23 24 1; ++i) { 25 // compare adjacent elements and swap them if first // element is greater than second element if (a[i] > a[i + 1]) { int hold = a[i]; a[i] = a[i + 1]; a[i + 1] = hold; 26 27 28 29 30 2.0 Agnial) 31 32 33 } 34 za roy algms 35 aluesi puts("\nData items in ascending order"); 36 37 // output sorted array for (size_t i = 0; i < SIZE; ++i) { printf("%4d", a[i]); ibarm.nssm ad 38 39 40 41 42 puts(""); 43 44 } Data items in original order 2 6 4 8 10 12 89 68 45 37 Data items in ascending order 2 4 6 8 10 12 37 45 68 89 Fig. 6.15 | Sorting an array's values into ascending order.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Question 6.11 . Answer in c

Transcribed Image Text:(Bubble Sort) The bubble sort presented in Fig. 6.15 is inefficient for large arrays. Make the
6.11
following simple modifications to improve its performance.
a) After the first pass, the largest number is guaranteed to be in the highest-numbered el-
ement of the array; after the second pass, the two highest numbers are "in place," and
so on. Instead of making nine comparisons on every pass, modify the bubble sort to
make eight comparisons on the second pass, seven on the third
b) The data in the array may already be in the proper or near-proper order, so why make
nine passes if fewer will suffice? Modify the sort to check at the end of each
any swaps have been made. If none has been made, then the data must already be in the
proper order, so the program should terminate. If swaps have been made, then at least
CC •
and
So on.
pass
whether
pass
one more pass is needed.
adimarninnalonoronorotione
11
![6.8 Sorting Arrays
increasing order (or if the values are identical), v
decreasing order, their values are swapped in the array.
235
// Fig. 6.15: fig06_15.c
// Sorting an array's values into ascending order.
#include <stdio.h>
#define SIZE 10
// function main begins program execution
int main(void)
// initialize a
int a[SIZE] = {2, 6, 4, 8, 10, 12, 89, 68, 45, 37};
10
%3D
puts ("Data items in original order");
12
13
// output original array
for (size_ti = 0; i < SIZE; ++i) {
printf("%4d", a[i]);
}
14
15
16
17
18
// bubble sort
//loop to control number of passes
for (unsigned int pass
19
20
gmis
21
1; pass < SIZE; ++pass) {
22
// loop to control number of comparisons per pass
for (size_t i = 0; i < SIZE
23
24
1; ++i) {
25
// compare adjacent elements and swap them if first
// element is greater than second element
if (a[i] > a[i + 1]) {
int hold = a[i];
a[i] = a[i + 1];
a[i + 1] = hold;
26
27
28
29
30
2.0
Agnial)
31
32
33
}
34
za roy
algms
35
aluesi
puts("\nData items in ascending order");
36
37
// output sorted array
for (size_t i = 0; i < SIZE; ++i) {
printf("%4d", a[i]);
ibarm.nssm ad
38
39
40
41
42
puts("");
43
44 }
Data items in original order
2 6 4 8 10 12 89 68 45 37
Data items in ascending order
2 4 6 8 10 12 37 45 68 89
Fig. 6.15 | Sorting an array's values into ascending order.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F38737e37-1715-4516-bd22-2f4c8bfeebf8%2Fd158ce6e-219b-48bd-a321-7b292152403c%2Fztivkru.jpeg&w=3840&q=75)
Transcribed Image Text:6.8 Sorting Arrays
increasing order (or if the values are identical), v
decreasing order, their values are swapped in the array.
235
// Fig. 6.15: fig06_15.c
// Sorting an array's values into ascending order.
#include <stdio.h>
#define SIZE 10
// function main begins program execution
int main(void)
// initialize a
int a[SIZE] = {2, 6, 4, 8, 10, 12, 89, 68, 45, 37};
10
%3D
puts ("Data items in original order");
12
13
// output original array
for (size_ti = 0; i < SIZE; ++i) {
printf("%4d", a[i]);
}
14
15
16
17
18
// bubble sort
//loop to control number of passes
for (unsigned int pass
19
20
gmis
21
1; pass < SIZE; ++pass) {
22
// loop to control number of comparisons per pass
for (size_t i = 0; i < SIZE
23
24
1; ++i) {
25
// compare adjacent elements and swap them if first
// element is greater than second element
if (a[i] > a[i + 1]) {
int hold = a[i];
a[i] = a[i + 1];
a[i + 1] = hold;
26
27
28
29
30
2.0
Agnial)
31
32
33
}
34
za roy
algms
35
aluesi
puts("\nData items in ascending order");
36
37
// output sorted array
for (size_t i = 0; i < SIZE; ++i) {
printf("%4d", a[i]);
ibarm.nssm ad
38
39
40
41
42
puts("");
43
44 }
Data items in original order
2 6 4 8 10 12 89 68 45 37
Data items in ascending order
2 4 6 8 10 12 37 45 68 89
Fig. 6.15 | Sorting an array's values into ascending order.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
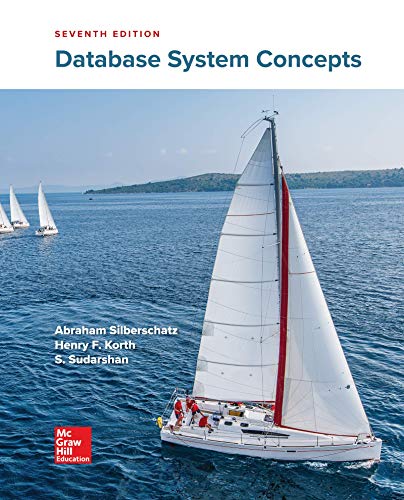
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
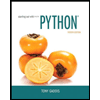
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
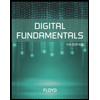
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
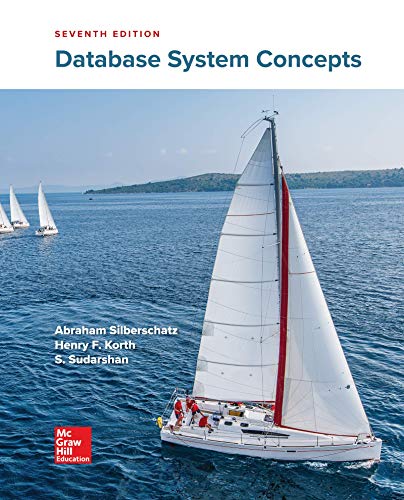
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
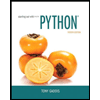
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
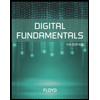
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
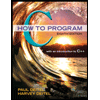
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
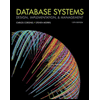
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
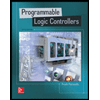
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education