B. Complete the method named alarmClock(); You are given a day of the week, with Sun and a boolean indicating if you are on vacation. Your method should return a String of the form "7:00" indicating when the alarm clock should ring. On weekdays, the alarm should be "7:00" and on the weekend it should be "10:00". Unless you are on vacation-then on weekdays it should be "10:00" and weekends it should be "off". 0, Mon = 1, Tue = 2, ... 2, ... Sat 6, %3D
B. Complete the method named alarmClock(); You are given a day of the week, with Sun and a boolean indicating if you are on vacation. Your method should return a String of the form "7:00" indicating when the alarm clock should ring. On weekdays, the alarm should be "7:00" and on the weekend it should be "10:00". Unless you are on vacation-then on weekdays it should be "10:00" and weekends it should be "off". 0, Mon = 1, Tue = 2, ... 2, ... Sat 6, %3D
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please help to program this

Transcribed Image Text:```
/**
* A. Complete the method named outsideIn3().
*
* The method has one input: a String str that is
* at least 3 characters long and of odd length.
* The output of the method is a new String where
* the middle character has been removed and placed
* both at the beginning and end.
*
* Examples:
* outsideIn3("Cartons") returns "tCaront" // t removed and placed at both ends
* outsideIn3("Hip") returns "iHip" // i removed placed at both ends.
* outsideIn3("Barbara Ann") returns "rBarbaa Annr" // 2nd r removed
*
* @param str the String to process
* @return a new String as described above.
*/
public String outsideIn3(String str)
{
// Complete the method here
}
```

Transcribed Image Text:### Code Example: Alarm Clock Method
**Task Description:**
You need to complete a method named `alarmClock()`. The method requires the following inputs:
- An integer representing a day of the week, where Sunday = 0, Monday = 1, Tuesday = 2, ..., Saturday = 6.
- A boolean indicating if you are on vacation.
**Method Functionality:**
- Return a string in the form of a time, such as "7:00", indicating when the alarm clock should ring.
- On weekdays (Monday to Friday), the alarm should be "7:00".
- On weekends (Saturday and Sunday), it should be "10:00".
- If you are on vacation:
- On weekdays, the alarm should be "10:00".
- On weekends, the alarm should be "off".
**Examples:**
1. `alarmClock(1, false)` returns `"7:00"`
2. `alarmClock(5, false)` returns `"7:00"`
3. `alarmClock(0, false)` returns `"10:00"`
4. `alarmClock(0, true)` returns `"off"`
**Parameters:**
- `@param day`: An integer representing the day of the week.
- `@param onVacation`: A boolean that is true if you are on vacation.
- `@return`: The time the alarm should go off.
**Method Header:**
```java
public String alarmClock(int day, boolean onVacation) {
// Complete the method here
}
```
This illustrative exercise helps you understand how to manipulate conditional statements based on day and vacation status within Java.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
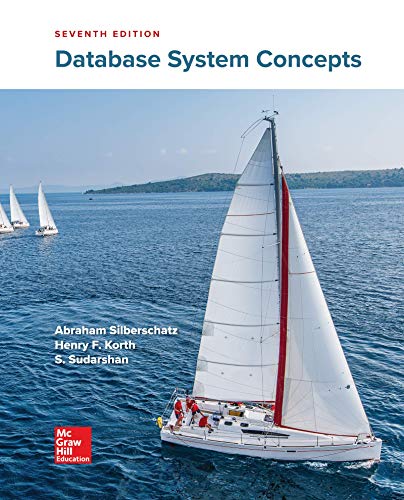
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
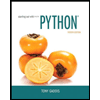
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
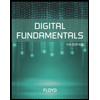
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
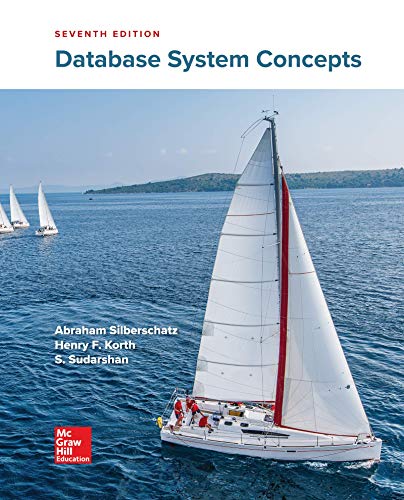
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
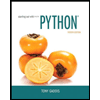
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
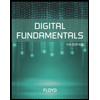
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
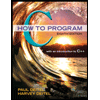
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
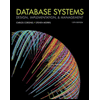
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
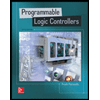
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education