Author: _________________________ #======================================================== #======================================================== # Program to sum numbers in an # array of 8-bit integers (byte) # and an array of 32-bit integers (.word) # (You MUST use indexed access for the words[] array) # (You may use indexed or pointer access for the byte[] array) # (Feel free to use the MUL instruction to multiply..) # -------------------------- C++ code ------------------ # #include # #include # using namespace std; # void main() # { # const int SIZE = 8; # byte[] bytes = { 6, 5, -1, 9, 2, 3, -8, 2, -4, 7 }; # int[] words = { 6, 5, 1, 9, 2, 3, 8, 2, 4, 7 }; # doSums(bytes, words, SIZE); # cout << endl; # } # void doSums(byte& b, int& w, int n) # { # int sum = 0; # for(int x = 1; x <= n; x++) { # if ( b[x] < 0 ) # sum = sum + ( b[x] * w[x-1] ); # else # sum = sum + ( b[x] * w[x+1] ); # cout << sum << " "; # }//end for # } # OUTPUT: 5 0 18 24 48 24 32 24 # ------------------------------------------------------- # Data Declarations .data .eqv SYS_PRINT_WORD 1 #word, byte, character .eqv SYS_PRINT_TEXT 4 #text (zero terminated) .eqv SYS_EXIT 10 #terminate .eqv SIZE 8 bytes: .byte 6, 5, -1, 9, 2, 3, -8, 2, -4, 7 words: .word 6, 5, 1, 9, 2, 3, 8, 2, 4, 7 endl: .asciiz "\n" blank2: .asciiz " " # ------------------------------------------------- # text/code section .text .globl main main: # ------ #call doSum(bytes, words, SIZE) #sum returned in v0 la $a0, bytes la $a1, words li $a2, SIZE jal doSums la $a0, endl li $v0, SYS_PRINT_TEXT syscall # ------ # Done, terminate program. li $v0, SYS_EXIT # call code for terminate syscall #.end main #-------------------------------------------------------------- # Subroutine: doSums() # Inputs: $a0 - address of bytes[] array of .byte (pointer) # $a1 - address of words[] array of .word (indexed) # $a2 - number of elements in the array #-------------------------------------------------------------- # int sum = 0; # for(int x = 1; x <= n; x++) { # if ( b[x] < 0 ) # sum = sum + ( b[x] * w[x-1] ); # else # sum = sum + ( b[x] * w[x+1] ); # cout << sum << " "; # }//end for #TODO: write the subroutine doSums: move $s5, $zero #sum loop: print: move $a0, $s5 li $v0, SYS_PRINT_WORD syscall la $a0, blank2 li $v0, SYS_PRINT_TEXT syscall endLoop: #TODO: code bottom of loop jr $ra #return when loop done..
# Author: _________________________
#========================================================
#========================================================
# Program to sum numbers in an
# array of 8-bit integers (byte)
# and an array of 32-bit integers (.word)
# (You MUST use indexed access for the words[] array)
# (You may use indexed or pointer access for the byte[] array)
# (Feel free to use the MUL instruction to multiply..)
# -------------------------- C++ code ------------------
# #include <iostream>
# #include <string>
# using namespace std;
# void main()
# {
# const int SIZE = 8;
# byte[] bytes = { 6, 5, -1, 9, 2, 3, -8, 2, -4, 7 };
# int[] words = { 6, 5, 1, 9, 2, 3, 8, 2, 4, 7 };
# doSums(bytes, words, SIZE);
# cout << endl;
# }
# void doSums(byte& b, int& w, int n)
# {
# int sum = 0;
# for(int x = 1; x <= n; x++) {
# if ( b[x] < 0 )
# sum = sum + ( b[x] * w[x-1] );
# else
# sum = sum + ( b[x] * w[x+1] );
# cout << sum << " ";
# }//end for
# }
# OUTPUT: 5 0 18 24 48 24 32 24
# -------------------------------------------------------
# Data Declarations
.data
.eqv SYS_PRINT_WORD 1 #word, byte, character
.eqv SYS_PRINT_TEXT 4 #text (zero terminated)
.eqv SYS_EXIT 10 #terminate
.eqv SIZE 8
bytes: .byte 6, 5, -1, 9, 2, 3, -8, 2, -4, 7
words: .word 6, 5, 1, 9, 2, 3, 8, 2, 4, 7
endl: .asciiz "\n"
blank2: .asciiz " "
# -------------------------------------------------
# text/code section
.text
.globl main
main:
# ------
#call doSum(bytes, words, SIZE) #sum returned in v0
la $a0, bytes
la $a1, words
li $a2, SIZE
jal doSums
la $a0, endl
li $v0, SYS_PRINT_TEXT
syscall
# ------
# Done, terminate program.
li $v0, SYS_EXIT # call code for terminate
syscall
#.end main
#--------------------------------------------------------------
# Subroutine: doSums()
# Inputs: $a0 - address of bytes[] array of .byte (pointer)
# $a1 - address of words[] array of .word (indexed)
# $a2 - number of elements in the array
#--------------------------------------------------------------
# int sum = 0;
# for(int x = 1; x <= n; x++) {
# if ( b[x] < 0 )
# sum = sum + ( b[x] * w[x-1] );
# else
# sum = sum + ( b[x] * w[x+1] );
# cout << sum << " ";
# }//end for
#TODO: write the subroutine
doSums: move $s5, $zero #sum
loop:
print: move $a0, $s5
li $v0, SYS_PRINT_WORD
syscall
la $a0, blank2
li $v0, SYS_PRINT_TEXT
syscall
endLoop: #TODO: code bottom of loop
jr $ra #return when loop done..

Step by step
Solved in 3 steps

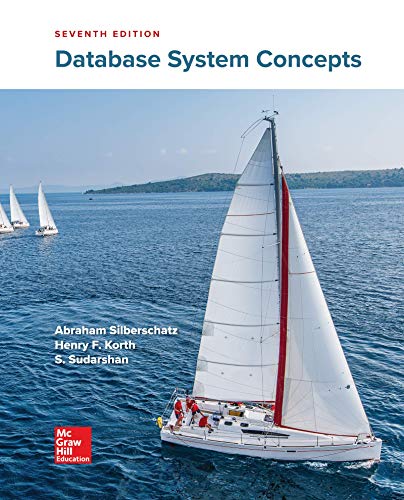
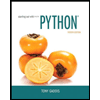
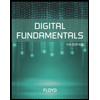
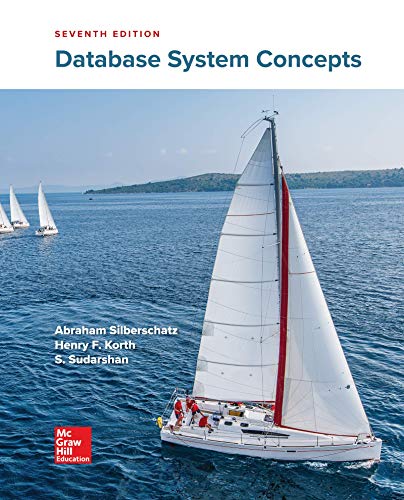
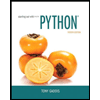
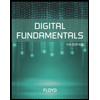
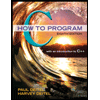
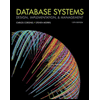
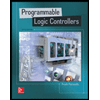