Assume you are using a backtracking algorithm to search for the node 40 in the max heap represented by the array 100, 50, 60, 40, 10, 20, 30. Assuming that you search from the root of the heap downwards recursively, which of the following is a valid order in which the nodes could have been first visited? 30, 20, 10, 40 100, 50, 60, 10, 30, 40 100, 60, 30, 20, 50, 10, 40 100, 60, 50, 30, 20, 10, 40 100, 60, 30, 20, 10, 40 Assume you are using a backtracking algorithm to search for the node 40 in the max heap represented by the array 100, 50, 60, 40, 10, 20, 30. What is the minimum number of nodes that must be searched in order to find the node 30? 1 5 6 3 4 Assume you have the following backtracking algorithm for finding a way to a desired location in a city organized in a grid as represented by the image. What is the problem with this proposed algorithm? Bool SearchCity(currentLocation,, desiredLocation, stepsToLocation) { If(currentLocation == desiredLocation) { Return true; } potentialSteps = new Stack(); if (NotBlocked(RightLocation)) { //location to the right of where I am facing potentialSteps.Push(RightLocation); } If (NotBlocked(ForwardLocation)) { //Location forward of where I am facing potentialSteps.Push(forwardLocation) } If (NotBlocked(LeftLocation)) { //Location to the left of where I am facing potentialSteps.Push(leftLocation) } While(NotEmpty(potentialSteps)) { nextLocation = potentialSteps.Pop(); stepsToLocation.Add(nextLocation) if(SearchCity(nextLocation, desiredLocation, stepsToLocation) == true) { return true; } stepsToLocation.RemoveLast() } Return false; } It does not return to previous locations after a dead end It will always go forward It will never take the right path It can be caught in an infinite loop by always going right first It does not check if a direction is blocked What is a potential solution to the problem in the previous question? Check if the current location is the destination Add the backwards location to the stack of locations to visit Do not visit a location contained in stepsToLocation Replace the stack with a Queue Only add either the left or right destinations as candidates Assuming the above algorithm is fixed, which of the following would improve its performance in finding a desired location? Always checking the forward direction first before the other directions Checking if the next location is the destination before making the recursive call Choosing candidate locations that are closest to the destination first Randomly selecting from the next possible locations Rotating which direction is to be searched first in a fixed order
Assume you are using a backtracking
30, 20, 10, 40
100, 50, 60, 10, 30, 40
100, 60, 30, 20, 50, 10, 40
100, 60, 50, 30, 20, 10, 40
100, 60, 30, 20, 10, 40
Assume you are using a backtracking algorithm to search for the node 40 in the max heap represented by the array 100, 50, 60, 40, 10, 20, 30. What is the minimum number of nodes that must be searched in order to find the node 30?
1
5
6
3
4
Assume you have the following backtracking algorithm for finding a way to a desired location in a city organized in a grid as represented by the image. What is the problem with this proposed algorithm?
Bool SearchCity(currentLocation,, desiredLocation, stepsToLocation) {
If(currentLocation == desiredLocation) {
Return true;
}
potentialSteps = new Stack();
if (NotBlocked(RightLocation)) { //location to the right of where I am facing
potentialSteps.Push(RightLocation);
}
If (NotBlocked(ForwardLocation)) { //Location forward of where I am facing
potentialSteps.Push(forwardLocation)
}
If (NotBlocked(LeftLocation)) { //Location to the left of where I am facing
potentialSteps.Push(leftLocation)
}
While(NotEmpty(potentialSteps)) {
nextLocation = potentialSteps.Pop();
stepsToLocation.Add(nextLocation)
if(SearchCity(nextLocation, desiredLocation, stepsToLocation) == true) {
return true;
}
stepsToLocation.RemoveLast()
}
Return false;
}
It does not return to previous locations after a dead end
It will always go forward
It will never take the right path
It can be caught in an infinite loop by always going right first
It does not check if a direction is blocked
What is a potential solution to the problem in the previous question?
Check if the current location is the destination
Add the backwards location to the stack of locations to visit
Do not visit a location contained in stepsToLocation
Replace the stack with a Queue
Only add either the left or right destinations as candidates
Assuming the above algorithm is fixed, which of the following would improve its performance in finding a desired location?
Always checking the forward direction first before the other directions
Checking if the next location is the destination before making the recursive call
Choosing candidate locations that are closest to the destination first
Randomly selecting from the next possible locations
Rotating which direction is to be searched first in a fixed order

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

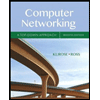
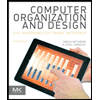
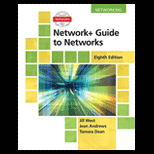
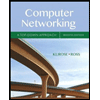
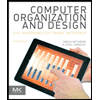
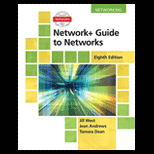
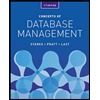
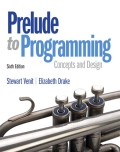
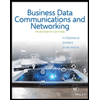