Assume we analyze a problem related to Geometrical Shapes and identify the following conceptual classes: Square, Circle, Cube, and Sphere. We also identify the following polymorphic behaviors: area). circumference(), and volume(). Square, Circle, Cube, and Sphere classes all have area() behavior. Square and Circle classes have circumference() ability. Cube and Sphere classes have volume() ability. Design and code a solution in C++ usingObject Oriented approach.
Assume we analyze a problem related to Geometrical Shapes and identify the following conceptual classes: Square, Circle, Cube, and Sphere. We also identify the following polymorphic behaviors: area). circumference(), and volume(). Square, Circle, Cube, and Sphere classes all have area() behavior. Square and Circle classes have circumference() ability. Cube and Sphere classes have volume() ability. Design and code a solution in C++ usingObject Oriented approach.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 3PE: Chapter 10 defined the class circleType to implement the basic properties of a circle. (Add the...
Related questions
Question
Assume we analyze a problem related to Geometrical Shapes and identify the following conceptual classes: Square, Circle, Cube, and Sphere. We also identify the following polymorphic behaviors: area). circumference(), and volume(). Square, Circle, Cube, and Sphere classes all have area() behavior. Square and Circle classes have circumference() ability. Cube and Sphere classes have volume() ability. Design and code a solution in C++ usingObject Oriented approach. We also provide you with an application code below to guide you.
Note: Volume of a sphere of radius R is 4/3 π R3. . Surface area of a sphere of radius R is 4πR2.
![### Artificial Intelligence Engineering Department
#### Bahçeşehir University
**Course Code: AIN-1002**
---
#### Sample Code for Managing Shape Objects in C++
The following sample code demonstrates how to manage and manipulate shape objects in C++. This code is intended to be used as a reference for learning purposes in the AI Engineering Department at Bahçeşehir University.
```cpp
for (int i=0; i<lengthOfShapes; ++i) {
cout << "Area of " << i << "th shape is " << shapes[i]->area() << endl;
}
for (int i=0; i<lengthOfPlanar; ++i) {
delete planar[i];
}
for (int i=0; i<lengthOfVolumetric; ++i) {
delete volumetric[i];
}
delete [] shapes;
return 0;
```
##### Explanation:
1. **Displaying Areas of Shapes**:
The first `for` loop iterates over all the shapes in the `shapes` array and prints out their respective areas using the `area()` method.
```cpp
for (int i=0; i<lengthOfShapes; ++i) {
cout << "Area of " << i << "th shape is " << shapes[i]->area() << endl;
}
```
2. **Deleting Planar Shapes**:
The second `for` loop iterates over all the planar shapes in the `planar` array to delete each object and free up memory.
```cpp
for (int i=0; i<lengthOfPlanar; ++i) {
delete planar[i];
}
```
3. **Deleting Volumetric Shapes**:
The third `for` loop iterates over all the volumetric shapes in the `volumetric` array to delete each object and free up memory.
```cpp
for (int i=0; i<lengthOfVolumetric; ++i) {
delete volumetric[i];
}
```
4. **Cleaning Up Remaining Shapes**:
Finally, the remaining shapes array is deleted to ensure all allocated memory is freed.
```cpp
delete [] shapes;
```
5. **Returning from the Main Function**:
The `return 0;` line indicates that the program has terminated successfully.
```cpp
return 0;
```
This](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2b3c45ef-1cfd-4f2a-b84e-474203f12787%2Fbeb97970-e135-4688-9811-e264fe36e2b9%2Fo3bo11b_processed.png&w=3840&q=75)
Transcribed Image Text:### Artificial Intelligence Engineering Department
#### Bahçeşehir University
**Course Code: AIN-1002**
---
#### Sample Code for Managing Shape Objects in C++
The following sample code demonstrates how to manage and manipulate shape objects in C++. This code is intended to be used as a reference for learning purposes in the AI Engineering Department at Bahçeşehir University.
```cpp
for (int i=0; i<lengthOfShapes; ++i) {
cout << "Area of " << i << "th shape is " << shapes[i]->area() << endl;
}
for (int i=0; i<lengthOfPlanar; ++i) {
delete planar[i];
}
for (int i=0; i<lengthOfVolumetric; ++i) {
delete volumetric[i];
}
delete [] shapes;
return 0;
```
##### Explanation:
1. **Displaying Areas of Shapes**:
The first `for` loop iterates over all the shapes in the `shapes` array and prints out their respective areas using the `area()` method.
```cpp
for (int i=0; i<lengthOfShapes; ++i) {
cout << "Area of " << i << "th shape is " << shapes[i]->area() << endl;
}
```
2. **Deleting Planar Shapes**:
The second `for` loop iterates over all the planar shapes in the `planar` array to delete each object and free up memory.
```cpp
for (int i=0; i<lengthOfPlanar; ++i) {
delete planar[i];
}
```
3. **Deleting Volumetric Shapes**:
The third `for` loop iterates over all the volumetric shapes in the `volumetric` array to delete each object and free up memory.
```cpp
for (int i=0; i<lengthOfVolumetric; ++i) {
delete volumetric[i];
}
```
4. **Cleaning Up Remaining Shapes**:
Finally, the remaining shapes array is deleted to ensure all allocated memory is freed.
```cpp
delete [] shapes;
```
5. **Returning from the Main Function**:
The `return 0;` line indicates that the program has terminated successfully.
```cpp
return 0;
```
This
![**Geometrical Shapes Analysis and Computation**
In this module, we will analyze a problem related to Geometrical Shapes and identify the following conceptual classes: Square, Circle, Cube, and Sphere. Additionally, we will identify the following polymorphic behaviors: `area()`, `circumference()`, and `volume()`. The following are the behaviors for each class:
- **Square and Circle:** Both have `area()` and `circumference()` abilities.
- **Cube and Sphere:** Both have `area()` and `volume()` abilities.
A solution in C++ using Object-Oriented Programming is provided below. This code illustrates the implementation of these behaviors.
### C++ Code for Geometrical Shapes
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class PlanarShape {
public:
virtual double area() const = 0;
virtual double circumference() const = 0;
};
class Circle : public PlanarShape {
double radius;
public:
Circle(double r) : radius(r) {}
double area() const override { return M_PI * radius * radius; }
double circumference() const override { return 2 * M_PI * radius; }
};
class Square : public PlanarShape {
double side;
public:
Square(double s) : side(s) {}
double area() const override { return side * side; }
double circumference() const override { return 4 * side; }
};
class VolumetricShape {
public:
virtual double area() const = 0;
virtual double volume() const = 0;
};
class Sphere : public VolumetricShape {
double radius;
public:
Sphere(double r) : radius(r) {}
double area() const override { return 4 * M_PI * radius * radius; }
double volume() const override { return (4.0 / 3.0) * M_PI * pow(radius, 3); }
};
class Cube : public VolumetricShape {
double side;
public:
Cube(double s) : side(s) {}
double area() const override { return 6 * side * side; }
double volume() const override { return side * side * side; }
};
int main() {
PlanarShape *planar[] = {
new Circle(1), // unit circle at (0,0)
new Square(1), // unit square, top-left corner](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2b3c45ef-1cfd-4f2a-b84e-474203f12787%2Fbeb97970-e135-4688-9811-e264fe36e2b9%2F3r8hrt_processed.png&w=3840&q=75)
Transcribed Image Text:**Geometrical Shapes Analysis and Computation**
In this module, we will analyze a problem related to Geometrical Shapes and identify the following conceptual classes: Square, Circle, Cube, and Sphere. Additionally, we will identify the following polymorphic behaviors: `area()`, `circumference()`, and `volume()`. The following are the behaviors for each class:
- **Square and Circle:** Both have `area()` and `circumference()` abilities.
- **Cube and Sphere:** Both have `area()` and `volume()` abilities.
A solution in C++ using Object-Oriented Programming is provided below. This code illustrates the implementation of these behaviors.
### C++ Code for Geometrical Shapes
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class PlanarShape {
public:
virtual double area() const = 0;
virtual double circumference() const = 0;
};
class Circle : public PlanarShape {
double radius;
public:
Circle(double r) : radius(r) {}
double area() const override { return M_PI * radius * radius; }
double circumference() const override { return 2 * M_PI * radius; }
};
class Square : public PlanarShape {
double side;
public:
Square(double s) : side(s) {}
double area() const override { return side * side; }
double circumference() const override { return 4 * side; }
};
class VolumetricShape {
public:
virtual double area() const = 0;
virtual double volume() const = 0;
};
class Sphere : public VolumetricShape {
double radius;
public:
Sphere(double r) : radius(r) {}
double area() const override { return 4 * M_PI * radius * radius; }
double volume() const override { return (4.0 / 3.0) * M_PI * pow(radius, 3); }
};
class Cube : public VolumetricShape {
double side;
public:
Cube(double s) : side(s) {}
double area() const override { return 6 * side * side; }
double volume() const override { return side * side * side; }
};
int main() {
PlanarShape *planar[] = {
new Circle(1), // unit circle at (0,0)
new Square(1), // unit square, top-left corner
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
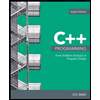
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
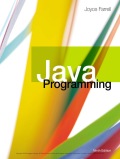
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
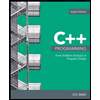
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
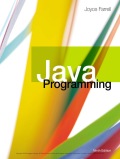
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT