Assume n is a positive integer representing input size for the following pseudocodes. Determine a function T(n) that relates input size n to number of runtime steps. (If you can't get the exact T(n), what it should roughly look like is enough. E.g. T(n) = an^2 +k) What Big O set does this function belong to? Use common Big O sets when possible. Show your thought process. Note: The ellipsis (...) is meant to indicate a repetition until the pattern is finished. (i.e. you can rewrite (1, 2, 3, 4, 5, 6, 7, 8, 9, 10) as (1, 2, ..., 10)). For fl it means the print() is repeated until you reach print(n). For f6, the looping is repeated until you reach for i_n in range(n). print() may be thought of as always being O(1). Hint: Treat these snippets not as code that would actually be written and run in python. Rather treat this as pseudocodes (especially the ones with ellipses). Think of what algorithm is represented (instead of how it is written) and how it scales with respect to n def f1(n): def f5(n) : print(1) print(2) while n > 0: print (n) n = (n/2) + 0.1 .. print (n-4) print (n-3) def f6(n): for i 1 in range (n) : for i 2 in range (n) : def f2(n): total = 0 ... for i n in range (n): for z in range (n): for i in range (n): for j in range (i): total += 1 f3 (n) def f7(n, b = ""): if n > 1: f7 (n/3, b + "0") f7 (n/3, b + "1") f7 (n/3, b + "2") f7 (n/3, b + "3") idef f3(n) : for i in range(n): for j in range (n): break else: print (n) print (b) idef f4(n, list_of_length_n): print (list_of_length_n)
Assume n is a positive integer representing input size for the following pseudocodes. Determine a function T(n) that relates input size n to number of runtime steps. (If you can't get the exact T(n), what it should roughly look like is enough. E.g. T(n) = an^2 +k) What Big O set does this function belong to? Use common Big O sets when possible. Show your thought process. Note: The ellipsis (...) is meant to indicate a repetition until the pattern is finished. (i.e. you can rewrite (1, 2, 3, 4, 5, 6, 7, 8, 9, 10) as (1, 2, ..., 10)). For fl it means the print() is repeated until you reach print(n). For f6, the looping is repeated until you reach for i_n in range(n). print() may be thought of as always being O(1). Hint: Treat these snippets not as code that would actually be written and run in python. Rather treat this as pseudocodes (especially the ones with ellipses). Think of what algorithm is represented (instead of how it is written) and how it scales with respect to n def f1(n): def f5(n) : print(1) print(2) while n > 0: print (n) n = (n/2) + 0.1 .. print (n-4) print (n-3) def f6(n): for i 1 in range (n) : for i 2 in range (n) : def f2(n): total = 0 ... for i n in range (n): for z in range (n): for i in range (n): for j in range (i): total += 1 f3 (n) def f7(n, b = ""): if n > 1: f7 (n/3, b + "0") f7 (n/3, b + "1") f7 (n/3, b + "2") f7 (n/3, b + "3") idef f3(n) : for i in range(n): for j in range (n): break else: print (n) print (b) idef f4(n, list_of_length_n): print (list_of_length_n)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Use python language

Transcribed Image Text:Assume n is a positive integer representing input size for the following
pseudocodes. Determine a function T(n) that relates input size n to number of runtime steps. (If you can't get the
exact T(n), what it should roughly look like is enough. E.g. T(n)= an^2 +k) What Big O set does this function
belong to? Use common Big O sets when possible. Show your thought process.
Note: The ellipsis (...) is meant to indicate a repetition until the pattern is finished. (i.e. you can rewrite (1, 2, 3, 4, 5, 6,
7, 8, 9, 10) as (1, 2, ..., 10)). For fl it means the print() is repeated until you reach print(n). For f6, the looping is
repeated until you reach for i_n in range(n). print() may be thought of as always being O(1).
Hint: Treat these snippets not as code that would actually be written and run in python. Rather treat this as
pseudocodes (especially the ones with ellipses). Think of what algorithm is represented (instead of how it is written)
and how it scales with respect to n
def f1(n) :
def f5(n) :
print(1)
print(2)
while n > 0:
print (n)
n = (n/2) + 0.1
..
print(n-4)
print (n-3)
def f6(n):
for i 1 in range(n):
for i 2 in range(n) :
def f2(n) :
total = 0
for i n in range (n):
for z in range (n):
for i in range(n):
for j in range(i):
total += 1
f3 (n)
def f7(n, b = ""):
if n > 1:
idef f3(n) :
for i in range(n):
f7 (n/3, b + "0")
f7 (n/3, b + "1")
f7(n/3, b + "2")
f7 (n/3, b +
for j in range(n):
"3")
break
else:
print (n)
print (b)
list of_length_n):
print (list_of_length_n)
idef f4(n,
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
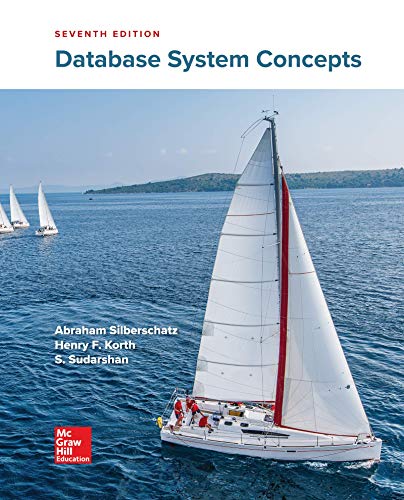
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
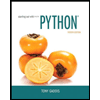
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
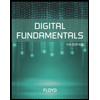
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
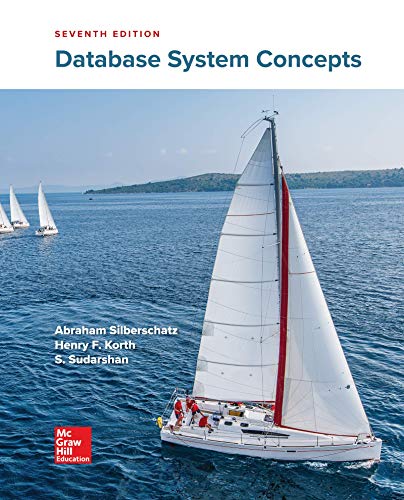
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
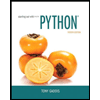
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
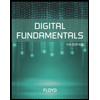
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
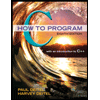
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
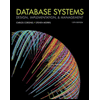
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
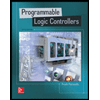
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education